用 C# 写入输出窗口
-
使用 C# 中的
Debug.Write()
方法写入 Microsoft Visual Studio IDE 的调试窗口 -
使用 C# 中的
Debug.WriteLine()
方法写入 Microsoft Visual Studio IDE 的调试窗口 -
使用 C# 中的
Debug.Print()
方法写入 Microsoft Visual Studio IDE 的调试窗口
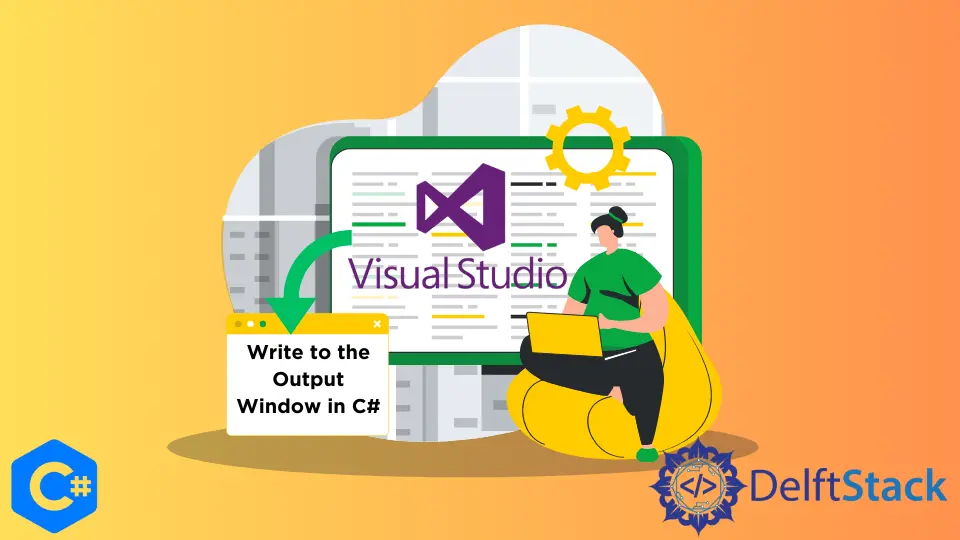
本教程将讨论如何将数据写入 C# 中的 Microsoft Visual Studio IDE 调试窗口。
使用 C# 中的 Debug.Write()
方法写入 Microsoft Visual Studio IDE 的调试窗口
C# 中的 Debug.Write()
方法(Debug.Write 方法(System.Diagnostics)| Microsoft Docs)将信息写入 Microsoft Visual Studio IDE 的调试窗口。它的工作方式与常规的 Console.Write()
方法类似,但会打印到调试窗口。我们必须使用 System.Diagnostics
命名空间才能在 C# 中使用 Debug.Write()
方法。下面的代码示例向我们展示了如何使用 C# 中的 Debug.Write()
方法向 Microsoft Visual Studio IDE 调试窗口中写入内容。
using System.Diagnostics;
namespace write_to_debug_window {
class Program {
static void Main(string[] args) {
Debug.Write("Hello, This is written in the Debug window");
}
}
}
调试窗口输出:
Hello, This is written in the Debug window
在上面的代码中,我们在 Microsoft Visual Studio IDE 调试窗口中打印了消息你好,这是在调试窗口中写的
。仅当我们在调试模式下运行应用程序时,此输出才会显示在调试窗口中。要以调试模式运行我们的应用程序,我们必须通过单击顶部的开始
按钮来运行我们的应用程序。还有其他一些功能,例如下面讨论的 Debug.Write()
方法。
使用 C# 中的 Debug.WriteLine()
方法写入 Microsoft Visual Studio IDE 的调试窗口
C# 中的 Debug.WriteLine()
方法将信息写入 Microsoft Visual Studio IDE 的调试窗口中。它的工作原理与常规的 Console.WriteLine()
方法类似,但会打印到调试窗口中。Debug.Write()
方法和 Debug.WriteLine()
方法之间的区别在于,Debug.Write()
方法仅将字符串写入调试窗口,但 Debug.WriteLine()
方法将写入字符串,并在调试窗口中使用整行。下面的代码示例向我们展示了如何使用 Debug.WriteLine()
方法将整个行打印到 Microsoft Visual Studio IDE 调试窗口。
using System.Diagnostics;
namespace write_to_debug_window {
class Program {
static void Main(string[] args) {
Debug.WriteLine("This is line1 in the Debug window");
Debug.WriteLine("This is line2 in the Debug window");
}
}
}
调试窗口输出:
This is line1 in the Debug window
This is line2 in the Debug window
在上面的代码中,我们使用 Debug.WriteLine()
方法在 Microsoft Visual Studio IDE 调试窗口中打印了两行。
使用 C# 中的 Debug.Print()
方法写入 Microsoft Visual Studio IDE 的调试窗口
C# 中的 [Debug.Print()
方法)还可以将信息写入 Microsoft Visual Studio IDE 的调试窗口。它也可以在 Microsoft Visual Studio IDE 调试窗口中打印一行,就像 Debug.WriteLine()
方法一样。请参见以下示例。
using System.Diagnostics;
namespace write_to_debug_window {
class Program {
static void Main(string[] args) {
Debug.Print("This is the print method");
Debug.Print("This is the 2nd print method");
}
}
}
调试窗口输出:
This is the print method
This is the 2nd print method
在上面的代码中,我们使用 Debug.Print()
方法在 Microsoft Visual Studio IDE 调试窗口中打印了两行。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn