C# 中的 Telnet 库
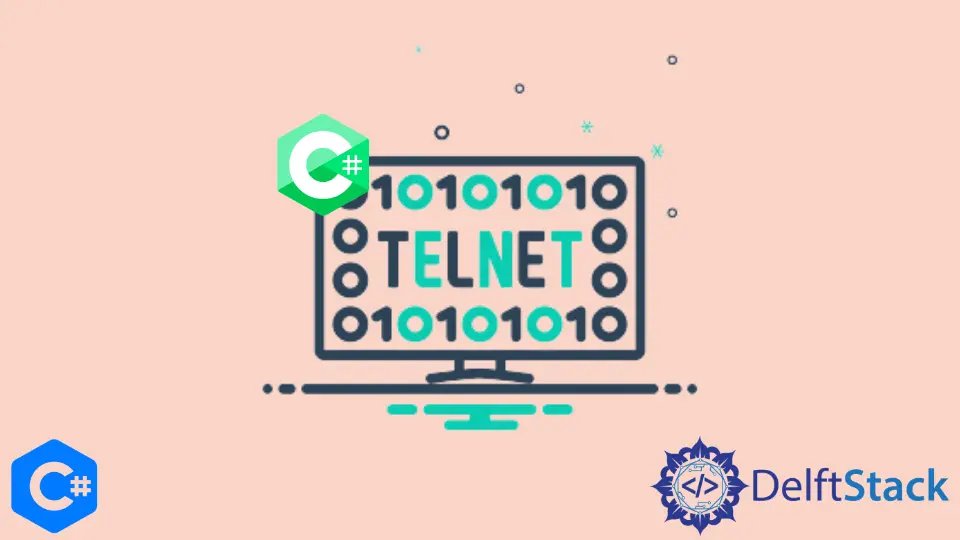
本教程将解释 Telnet 库并用 C# 演示一个简单的 Telnet 接口。
C#
中的 Telnet 库
Telnet 是一种网络协议,允许你虚拟连接到计算机并在两个工作站之间建立双向、相互支持、基于文本的通信通道。
它使用用户命令传输控制协议/互联网协议 (TCP/IP) 网络协议创建远程会话。
在 Web 上,超文本传输协议 (HTTP) 和文件传输协议 (FTP) 仅允许用户从远程计算机请求特定文件。
但是,Telnet 允许用户以普通用户的身份登录,并具有为该机器上的特定应用程序和数据提供的功能。
例子:
此类的可能应用程序包括 Telnet 终端、基于必须在服务器上运行的某些 UNIX 命令的 Windows GUI,以及执行 telnet 脚本的软件。
此代码提供了一个简约的终端和通过文件管道部署脚本的选项。
首先,导入以下库:
using System;
using System.Collections.Generic;
using System.Text;
在命名空间 MinimalisticTelnet
中创建一个 Telnet
类来编写代码。
namespace MinimalisticTelnet {
class Telnet {}
}
在 Main
函数中的端口 23
上创建一个名为 Tcon
到主机名 shani
的新 telnet 连接。Telnet 协议通常使用端口 Port 23
。
TelnetConnection Tcon = new TelnetConnection("shani", 23);
在 Login()
方法的帮助下,构造一个字符串变量 t
,它将包含登录凭据,例如 root
、rootpassword
和 timeout
。我们提供了 root
、rootpassword
和 130 秒的 timeout
。
它将显示服务器输出,如你在以下代码行中所见。
string t = Tcon.Login("root", "rootpassword", 130);
Console.Write(t);
获得服务器输出后,我们将验证服务器输出。它应该以 $
或 >
结尾;否则,将引发异常,并显示消息 Something went wrong, failed to connect
。
string Prmpt = t.TrimEnd();
Prmpt = t.Substring(Prmpt.Length - 1, 1);
if (Prmpt != "$" && Prmpt != ">")
throw new Exception("Something went wrong failed to connect ");
Prmpt = "";
现在,我们将使用 while
循环并将 telnet 连接 Tcon
作为参数传递,以查看它是否已连接。如果已连接,则循环运行;否则,它将终止并显示消息 Disconnected
。
while (Tcon.IsConnected && Prmpt.Trim() != "exit") {
}
Console.WriteLine("Disconnected");
Console.ReadLine();
最后,在 while
循环的主体中编写以下代码。
while (Tcon.IsConnected && Prmpt.Trim() != "exit") {
Console.Write(Tcon.Read());
Prmpt = Console.ReadLine();
Tcon.WriteLine(Prmpt);
Console.Write(Tcon.Read());
}
服务器的输出将显示在以下代码行中。
Console.Write(Tcon.Read());
这会将所有客户端的信息或输入传输到服务器。
Prmpt = Console.ReadLine();
Tcon.WriteLine(Prmpt);
输入客户端信息后,此代码将显示服务器的输出。
Console.Write(Tcon.Read());
示例代码:
using System;
using System.Collections.Generic;
using System.Text;
namespace MinimalisticTelnet {
class Telnet {
static void Main(string[] args) {
TelnetConnection Tcon = new TelnetConnection("shani", 23);
string t = Tcon.Login("root", "rootpassword", 130);
Console.Write(t);
string Prmpt = t.TrimEnd();
Prmpt = t.Substring(Prmpt.Length - 1, 1);
if (Prmpt != "$" && Prmpt != ">")
throw new Exception("Something went wrong failed to connect ");
Prmpt = "";
while (Tcon.IsConnected && Prmpt.Trim() != "exit") {
Console.Write(Tcon.Read());
Prmpt = Console.ReadLine();
Tcon.WriteLine(Prmpt);
Console.Write(Tcon.Read());
}
Console.WriteLine("Disconnected");
Console.ReadLine();
}
}
}
C#
中 Telnet 库的应用
Telnet 允许用户连接到任何使用基于文本的未加密协议的程序,例如 Web 服务器和端口。
Telnet 可用于在服务器上执行一系列任务,包括文件编辑、程序执行和电子邮件检查。
各种服务器通过 Telnet 提供对公共数据的远程访问,允许用户玩简单的游戏或查看天气预报。其中许多功能之所以持续存在,是因为它们怀旧或与需要某些数据的早期系统兼容。
C#
中 Telnet 库的后果
Telnet 存在安全漏洞。这是一个未加密的、不安全的协议。
在 Telnet 会话期间,任何监视用户连接的人都可以看到用户的登录名、密码和其他以明文形式编写的私人信息。此数据可用于访问用户的设备。
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn