在 C# 中将字符串数组转换为字符串
-
在
C#
中使用Join()
方法将字符串数组转换为字符串 -
在
C#
中使用Concat()
将字符串数组转换为字符串 -
在
C#
中使用String Builder()
将字符串数组转换为字符串
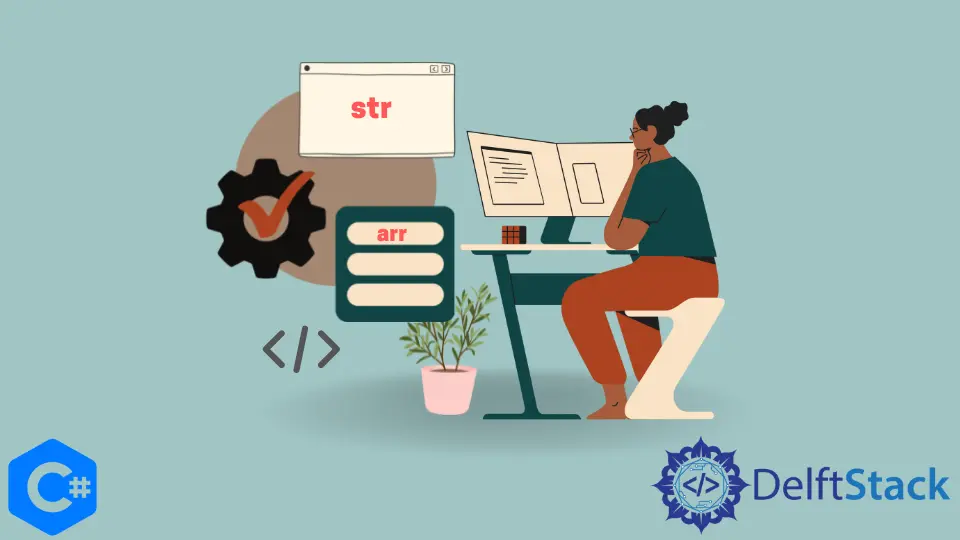
在本文中,我们将学习如何在 C# 中将字符串数组转换为单个字符串。
在 C#
中使用 Join()
方法将字符串数组转换为字符串
Join()
方法用于将字符串数组转换为单个字符串。我们可以使用它来创建一个包含两个元素的数组,每个字符串都表示为一个严格的字符串。
语法:
string.Join("", str);
例子:
using System;
class HelloWorld
{
static void Main()
{
string[] str = new string[2];
str[0] = "Hello";
str[1] = "World";
string res = string.Join(".", str);
Console.WriteLine($"{res}");
}
}
输出:
Hello.World
我们将字符串变量 str
初始化为 2 来存储初始化的字符串,并使用 Join()
将 str[0]
和 str[1]
组合起来。
在 C#
中使用 Concat()
将字符串数组转换为字符串
Concat()
方法用于轻松地将字符串数组转换为单个字符串。
语法:
string result = String.Concat(str);
例子:
using System;
public class HelloWorld {
public static void Main(string[] args) {
string[] str = new string[2];
str[0] = "Hello ";
str[1] = "World";
string res = String.Concat(str);
Console.WriteLine($"{res}");
}
}
输出:
Hello World
我们初始化一个字符串变量 str
为 2
和一个名为 result 的字符串变量来存储结果字符串并使用 Concat()
结合 str[0]
和 str[1]
并且存储导致结果
中的字符串。
一个优点是在 Concat()
方法中,我们不必像 Join()
那样使用 null 或空参数。
在 C#
中使用 String Builder()
将字符串数组转换为字符串
当我们在添加元素之前循环遍历字符串数组时,String Builder()
技术是理想的。
语法:
StringBuilder bdr = new StringBuilder();
foreach (string value in array)
{
bdr.Append(value);
bdr.Append('.');
}
例子:
using System;
using System.Text;
class HelloWorld
{
static void Main()
{
string[] array = new string[] { "Hello","World"};
StringBuilder bdr = new StringBuilder();
foreach (string value in array)
{
bdr.Append(value);
bdr.Append('.');
}
string res = bdr.ToString();
Console.WriteLine($" {res}");
}
}
输出:
Hello.World.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn相关文章 - Csharp Array
- 在 C# 中对数组排序
- 在 C# 中以降序对数组进行排序
- 如何在 C# 中删除数组的元素
- 如何在 C# 中将字符串转换为字节数组
- 向 C# 数组中添加数值
- C# 读取 CSV 文件并将其值存储到数组中