如何在 C++ 中循环遍历数组
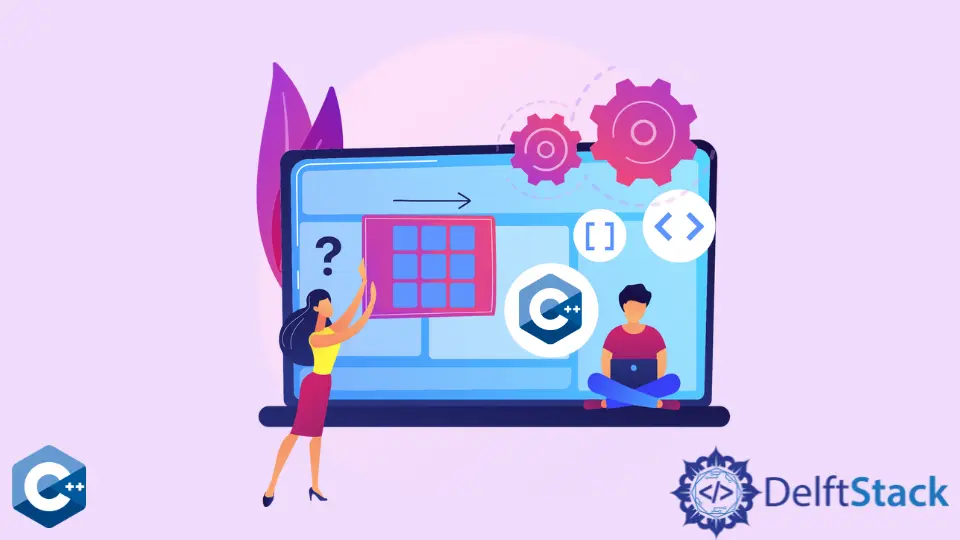
本文将说明如何使用 C++ 中的不同方法遍历数组。
使用 for
循环在数组上迭代的方法
对数组元素进行遍历的一个明显可见的方法是 for
循环。它由三部分语句组成,每一部分用逗号分隔。首先,我们应该初始化计数器变量-i
,根据设计,它只执行一次。接下来的部分声明一个条件,这个条件将在每次迭代时被评估,如果返回 false
,循环就停止运行。在这种情况下,我们比较计数器和数组的大小。最后一部分递增计数器,它也在每个循环周期执行。
#include <array>
#include <iostream>
using std::array;
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
array<string, 10> str_arr = {"Albatross", "Auklet", "Bluebird", "Blackbird",
"Cowbird", "Dove", "Duck", "Godwit",
"Gull", "Hawk"};
for (size_t i = 0; i < str_arr.size(); ++i) {
cout << str_arr[i] << " - ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
Albatross - Auklet - Bluebird - Blackbird - Cowbird - Dove - Duck - Godwit - Gull - Hawk -
使用基于范围的循环来迭代一个数组
基于范围的循环是传统 for
循环的可读版本。这种方法是一种强大的替代方法,因为它在复杂的容器上提供了简单的迭代,并且仍然保持了访问每个元素的灵活性。下面的代码示例是前面例子的精确再实现。
#include <array>
#include <iostream>
using std::array;
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
array<string, 10> str_arr = {"Albatross", "Auklet", "Bluebird", "Blackbird",
"Cowbird", "Dove", "Duck", "Godwit",
"Gull", "Hawk"};
for (auto &item : str_arr) {
cout << item << " - ";
}
cout << endl;
return EXIT_SUCCESS;
}
使用 std::for_each
算法遍历数组
for_each
是一个强大的 STL 算法,用于操作范围元素和应用自定义定义的函数。它将范围起始和最后一个迭代器对象作为前两个参数,函数对象作为第三个参数。本例中,我们将函数对象声明为 lambda
表达式,直接将计算结果输出到控制台。最后,我们可以将 custom_func
变量作为参数传递给 for_each
方法,对数组元素进行操作。
#include <array>
#include <iostream>
using std::array;
using std::cin;
using std::cout;
using std::endl;
using std::for_each;
using std::string;
int main() {
array<int, 10> int_arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto custom_func = [](auto &i) { cout << i * (i + i) << "; "; };
;
for_each(int_arr.begin(), int_arr.end(), custom_func);
cout << endl;
return EXIT_SUCCESS;
}
输出:
2; 8; 18; 32; 50; 72; 98; 128; 162; 200;
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu