在 C++ 中初始化对象数组
Jinku Hu
2023年10月12日
-
使用
new
运算符在 C++ 中使用参数化构造函数初始化对象数组 -
使用
std::vector::push_back
函数初始化带有参数化构造函数的对象数组 -
使用
std::vector::emplace_back
函数初始化带有参数化构造函数的对象数组
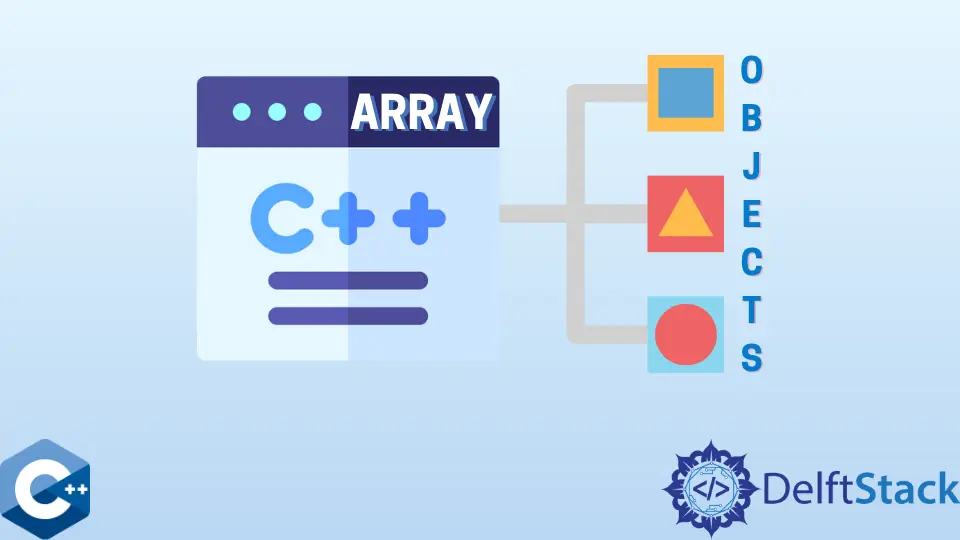
本文将演示在 C++ 中用参数化构造函数初始化对象数组的多种方法。
使用 new
运算符在 C++ 中使用参数化构造函数初始化对象数组
new
运算符是 C++ 动态内存管理接口的一部分,它等效于 C 语言中的 malloc
函数。请注意,动态内存管理需要用户分配指定固定大小的内存并将其在不再需要时返回给系统的流程。
new
用于从系统请求固定数量的字节,如果调用成功,它将返回指向存储区域的指针。在下面的示例中,我们定义了一个名为 Pair
的类,它具有两个构造函数和一个 printPair
,以将数据成员输出到控制台。首先,我们需要使用 new
运算符分配一个由四对组成的 Pairs
数组。接下来,我们可以使用 for 循环遍历数组,并且每次迭代都使用参数化的构造函数初始化该元素。请注意,应该在程序退出之前使用 delete
操作符释放分配的数组。还要注意,delete []
符号对于取消分配数组的每个元素是必需的。
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
class Pair {
int x, y;
public:
Pair() = default;
Pair(int a, int b) : x(a), y(b) {}
void printPair() const { cout << "x: " << x << endl << "y: " << y << endl; }
};
int main() {
Pair *arr = new Pair[4];
for (int i = 0; i < 4; ++i) {
arr[i] = Pair(i * 2, i * i);
}
for (int i = 0; i < 4; ++i) {
arr[i].printPair();
}
delete[] arr;
return EXIT_SUCCESS;
}
输出:
x: 0
y: 0
x: 2
y: 1
x: 4
y: 4
x: 6
y: 9
使用 std::vector::push_back
函数初始化带有参数化构造函数的对象数组
另外,更多的无头方法是将对象存储在 std::vector
容器中,该容器将提供内置函数来动态初始化新元素。即,Pair
定义保持不变,但是每次迭代时,我们将调用 push_back
函数,并将其从第二个构造函数传递给 Pair
值。从好的方面来说,我们不必担心内存资源的释放。他们会自动清理。
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
class Pair {
int x, y;
public:
Pair() = default;
Pair(int a, int b) : x(a), y(b) {}
void printPair() const { cout << "x: " << x << endl << "y: " << y << endl; }
};
int main() {
vector<Pair> array;
for (int i = 0; i < 4; ++i) {
array.push_back(Pair(i * 2, i * i));
}
for (const auto &item : array) {
item.printPair();
}
return EXIT_SUCCESS;
}
输出:
x: 0
y: 0
x: 2
y: 1
x: 4
y: 4
x: 6
y: 9
使用 std::vector::emplace_back
函数初始化带有参数化构造函数的对象数组
用参数化构造函数初始化对象数组的另一种方法是利用 std::vector
类的 emplace_back
函数。这样,我们只需要传递 Pair
构造函数的值,而 emplace_back
会自动为我们构造一个新元素。像以前的方法一样,该方法也不需要用户担心内存管理。
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
class Pair {
int x, y;
public:
Pair() = default;
Pair(int a, int b) : x(a), y(b) {}
void printPair() const { cout << "x: " << x << endl << "y: " << y << endl; }
};
int main() {
vector<Pair> array;
for (int i = 0; i < 4; ++i) {
array.emplace_back(i * 2, i * i);
}
for (const auto &item : array) {
item.printPair();
}
return EXIT_SUCCESS;
}
输出:
x: 0
y: 0
x: 2
y: 1
x: 4
y: 4
x: 6
y: 9
作者: Jinku Hu