Python 教程 - 異常處理
Jinku Hu
2023年1月30日
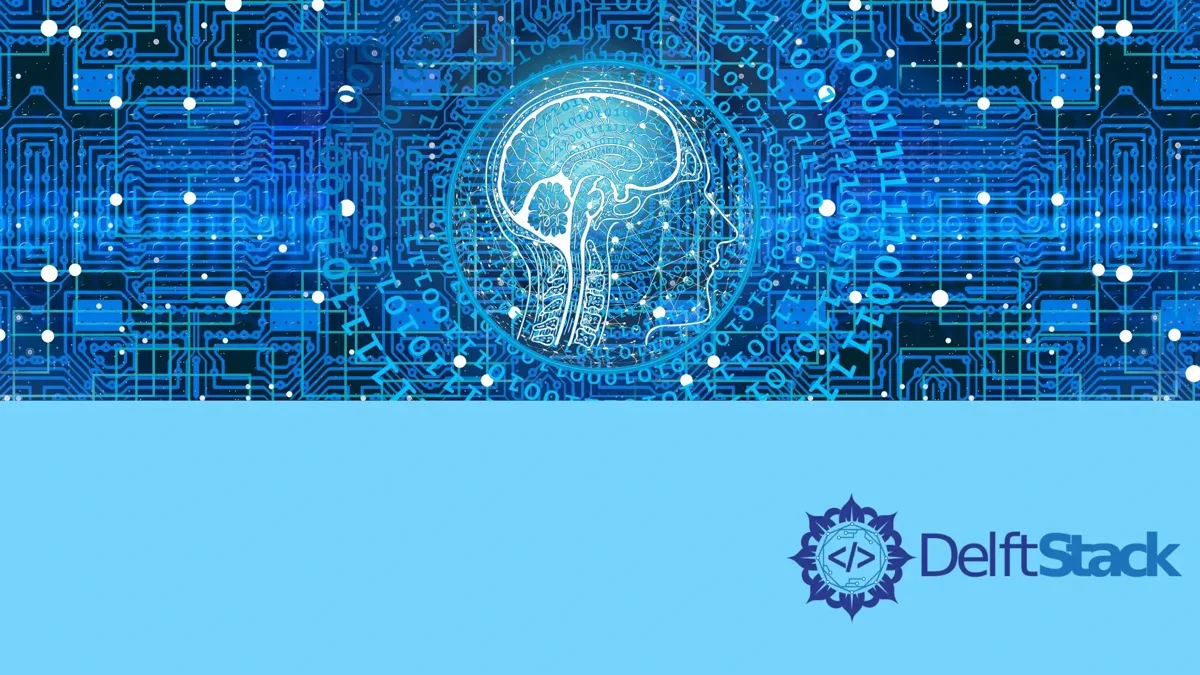
你將在本節中學習如何處理 Python 異常。此外,你將學習引發和捕獲異常。
當程式中發生異常時,程式執行將終止。但是大多數時候,你不希望程式異常終止,因此你可以使用 Python 異常處理來避免程式崩潰。
try...except
在下面的示例中,要求使用者輸入 10 個整數。如果使用者輸入其他型別而不是整數,則將引發異常並執行 except
中的語句:
>>> n = 10
>>> i = 1
>>> sum = 0
>>> while i <= n:
try:
num = int(input('Enter a number: '))
sum = sum + num
i = i+1
except:
print('Please enter an integer!!')
break
Enter a number: 23
Enter a number: 12
Enter a number: Asd
Please enter an integer!!
>>> print('Sum of numbers =', sum)
Sum of numbers = 35
你還可以捕獲指定的異常,例如,如果發生被零除的情況,則可以捕獲對應的異常為:
try:
# statements
except ZeroDivisionError:
# exception handling
except:
# all other exceptions are handled here
raise
異常
你可以使用 raise
關鍵字強制發生特定的異常:
>>> raise ZeroDivisionError
Traceback (most recent call last):
File "<pyshell#17>", line 1, in <module>
raise ZeroDivisionError
ZeroDivisionError
>>> raise ValueError
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
raise ValueError
ValueError
如果需要向自舉異常新增一些自定義資訊,則可以將其新增到異常錯誤型別後的括號中。
>>> raise NameError("This is a customized exception")
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
raise NameError("This is a customized exception")
NameError: This is a customized exception
try...finally
finally
在異常處理 try
中是一個可選項。如果有一個 finally
塊,無論結果如何,程式都會執行它。
在以下示例中我們對此進行演示:
>>> try:
raise ValueError
finally:
print('You are in finally block')
You are in finally block
Traceback (most recent call last):
File "<pyshell#23>", line 2, in <module>
raise ValueError
ValueError
try...finally
結構用於清理操作,例如在處理檔案時,最好關閉檔案。因此,檔案將在 finally
塊中關閉。
Python 內建異常
在本節中,你將學習 Python 程式設計中的標準異常。
異常是解釋期間引發的執行時錯誤。例如,將任何數字除以零將產生一個稱為 ZeroDivisionError
的執行時錯誤。
在 Python 中,下面描述了一些內建的異常:
異常 | 什麼時候觸發? |
---|---|
AssertionError |
當 assert 語句失敗 |
AttributeError |
當 attribute 引用失敗時 |
EOFError |
當 input() 函式未讀取任何資料併到達檔案末尾時 |
FloatingPointError |
當浮點計算失敗時 |
GeneratorExit |
當協程或生成器關閉時,GeneratorExit 發生異常 |
ImportError |
當 import 找不到模組時 |
ModuleNotFoundError |
當 import 找不到模組時。此異常是 ImportError 的子類 |
IndexError |
當序列的索引不在範圍內時 |
KeyError |
當在字典中找不到字典鍵時 |
KeyboardInterrupt |
當按下中斷鍵時 |
MemoryError |
當指定操作的記憶體量較少時 |
NameError |
當找不到變數時 |
NotImplementedError |
需要但不提供抽象方法的實現時 |
OSError |
遇到系統錯誤時 |
OverFlowError |
當值太大而無法表示時 |
RecursionError |
當遞迴限制超過最大數量時 |
IndentationError |
為任何函式,類等的定義正確指定縮排時 |
SystemError |
當發現與系統相關的錯誤時 |
SystemExit |
當 sys.exit() 用於退出直譯器時 |
TypeError |
當操作對於指定的資料型別無效時 |
ValueError |
當內建函式具有有效的自變數但提供的值無效時 |
RunTimeError |
當生成的錯誤不在其他類別中時 |
IOError |
當 I/O 操作失敗時 |
UnBoundLocalError |
當訪問區域性變數但沒有值時 |
SyntaxError |
當有語法錯誤時 |
TabError |
當有不必要的製表符縮排時 |
UnicodeError |
當遇到 Unicode 相關錯誤時 |
UnicodeEncodeError |
由於編碼遇到 Unicode 相關錯誤時 |
UnicodeDecodeError |
由於解碼遇到 Unicode 相關錯誤時 |
UnicodeTranslateError |
由於翻譯而遇到 Unicode 相關錯誤時 |
ZeroDivisionError |
當數字除以零時 |