在 VBA 中宣告和初始化字串陣列
Glen Alfaro
2023年1月30日
VBA
VBA Array
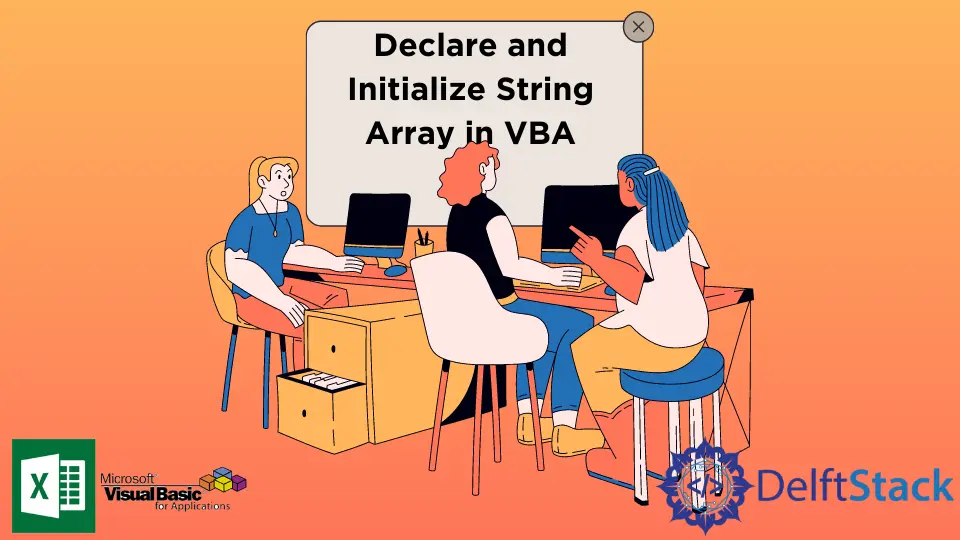
在任何程式語言的任何程式碼執行中,儲存資訊的需求有時是不可避免的。好訊息是 VBA 在儲存資料時允許多種選擇,其中之一就是陣列。
在 VBA 中,陣列根據其長度和資料型別的靈活性進行分類。
- 動態陣列 - 長度和資料型別可變的陣列
- 靜態陣列 - 長度和資料型別固定的陣列。
此外,VBA 中的陣列也可以根據它們的維度進行分類。
- 單維陣列 - 只有一個單行陣列的陣列。
- 多維陣列——具有多個單行陣列的陣列。
宣告並初始化動態字串陣列
下面的程式碼塊將演示如何建立和初始化字串陣列。
方法 1 - 宣告為 Variant
資料型別
通過在變體資料型別中建立變數來宣告動態陣列。然後陣列將由一個集合(Array()
)初始化。
Sub DynamicArrayDemo()
Dim stringArray As Variant
stringArray = Array("Lion", "Tiger", "Cheetah", "Monkey", "Elephant", "Zebra")
Debug.Print stringArray(3)
End Sub
DynamicArrayDemo
輸出:
Monkey
方法 2 - 宣告為字串,然後使用 Split()
函式
宣告一個名為 stringArray
的字串陣列,而不顯式宣告邊界。
Sub DynamicArrayDemo()
Dim stringArray() As String
Dim str As String
str = "Lion,Tiger,Cheetah,Monkey,Elephant,Zebra"
stringArray = Split("Lion,Tiger,Cheetah,Monkey,Elephant,Zebra", ",")
Debug.Print stringArray(2)
End Sub
DynamicArrayDemo
輸出:
Cheetah
宣告並初始化靜態字串陣列
下面的程式碼塊演示了宣告和初始化靜態字串陣列的不同方法。
方法 1 - 宣告 LowerBound
和 UpperBound
:
通過顯式宣告其第一個和最後一個元素來宣告一個靜態字串陣列。
語法:
Dim stringArray([LowerBound] To [UpperBound]) As String
引數:
[LowerBound] |
陣列的第一個元素所引用的鍵整數。 |
[UpperBound] |
引用陣列最後一個元素的鍵整數。 |
下面的示例將宣告一個名為 stringArray
的字串陣列,其中包含從元素 0 到 5 的六個元素。
Sub StaticArrayDemo()
Dim stringArray(0 To 5) As String
stringArray(0) = "Lion"
stringArray(1) = "Tiger"
stringArray(2) = "Cheetah"
stringArray(3) = "Monkey"
stringArray(4) = "Elephant"
stringArray(5) = "Zebra"
Debug.Print stringArray(4)
End Sub
StaticArrayDemo
輸出:
Elephant
方法 2 - 顯式更改下限
用一個通用的 Lower bound
值宣告一個字串陣列。
Option Base 1
Sub StaticArrayDemo()
Dim stringArray(6) As String
stringArray(1) = "Lion"
stringArray(2) = "Tiger"
stringArray(3) = "Cheetah"
stringArray(4) = "Monkey"
stringArray(5) = "Elephant"
stringArray(6) = "Zebra"
Debug.Print stringArray(1)
End Sub
StaticArrayDemo
輸出:
Lion
方法 3 - 使用多維陣列宣告和初始化
在 VBA 中,你可以宣告最多 60 維的陣列。
語法:
Dim stingArray( [LowerBound1] to [UpperBound1],[LowerBound2] to [UpperBound2], . . . ) as String
引數:
[LowerBound1] |
關鍵整數是第一個陣列維度上引用的第一個陣列元素。 |
[UpperBound1] |
關鍵整數是第一個陣列維度上引用的最後一個陣列元素。 |
[LowerBound2] |
關鍵整數是在第二個陣列維度上引用的第一個陣列元素。 |
[UpperBound2] |
關鍵整數是第二個陣列維度上引用的最後一個陣列元素。 |
在下面的示例中,宣告瞭一個多維陣列,其中第一個維度是 1 到 5;然後另一個是 1 到 5。
Sub MultiStaticArrayDemo()
Dim stringArray(1 To 5, 1 To 5) As String
Dim i, j As Integer
For i = 1 To 5
For j = 1 To 5
stringArray(i, j) = "The value of (" & i & "," & j & ") is " & i * j
Debug.Print stringArray(i, j)
Next j
Next i
End Sub
MultiStaticArrayDemo
輸出:
The value of (1,1) is 1
The value of (1,2) is 2
The value of (1,3) is 3
The value of (1,4) is 4
The value of (1,5) is 5
The value of (2,1) is 2
The value of (2,2) is 4
The value of (2,3) is 6
The value of (2,4) is 8
The value of (2,5) is 10
The value of (3,1) is 3
The value of (3,2) is 6
The value of (3,3) is 9
The value of (3,4) is 12
The value of (3,5) is 15
The value of (4,1) is 4
The value of (4,2) is 8
The value of (4,3) is 12
The value of (4,4) is 16
The value of (4,5) is 20
The value of (5,1) is 5
The value of (5,2) is 10
The value of (5,3) is 15
The value of (5,4) is 20
The value of (5,5) is 25
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe