TypeScript 中排除屬性
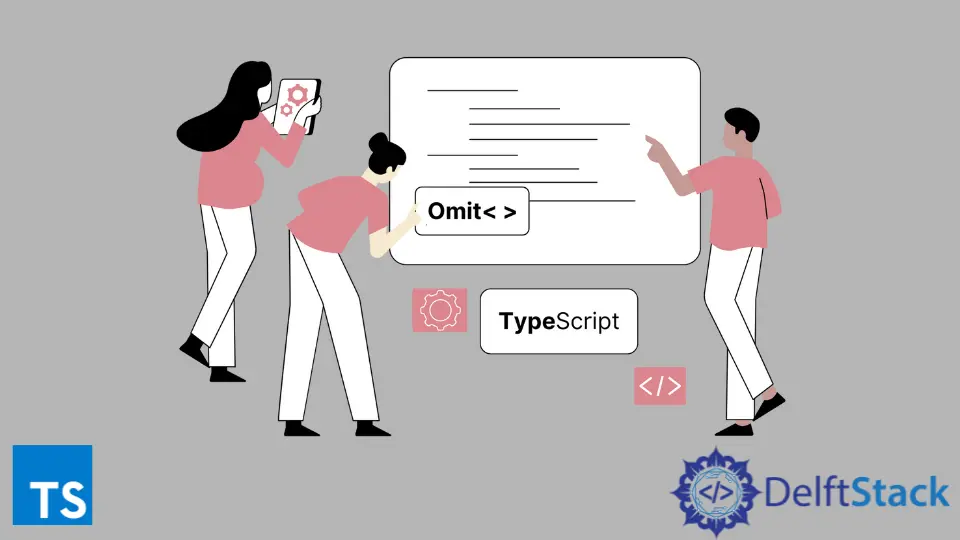
本教程將討論從 TypeScript 型別中排除屬性。exclude 屬性用於從現有型別建立具有較少或修改屬性的另一種型別。
在 TypeScript 中使用 Omit
從型別中排除屬性
TypeScript 允許使用者從型別中排除屬性。使用者必須使用 Omit
型別從現有型別中排除屬性。
Omit
型別從現有型別建立一個新型別。
語法:
Omit<Type_Name, 'property'>
例子:
type Info = {
firstName: string,
lastName: string,
age: number,
country: string,
};
type Info1 = Omit<Info, 'country'>;
const info1: Info1 = {
firstName: 'John',
lastName: 'Nash',
age: 27,
};
console.log(info1);
輸出:
{
"firstName": "John",
"lastName": "Nash",
"age": 27
}
在上面的示例中,使用 Omit
從現有型別中引入了一個新型別。Info
型別有四個屬性,而 Info1
有三個。
我們甚至可以從現有型別 Info
中引入一個新型別,並且可以排除多個屬性。
例子:
type Info = {
firstName: string,
lastName: string,
age: number,
country: string,
};
type Info1 = Omit<Info, 'country'>;
const info1: Info1 = {
firstName: 'John',
lastName: 'Nash',
age: 27,
};
type Info2 = Omit<Info, 'country' | 'age'>;
const info2: Info2 = {
firstName: 'Stewen',
lastName: 'Gold',
};
console.log(info1);
console.log(info2);
輸出:
{
"firstName": "John",
"lastName": "Nash",
"age": 27
}
{
"firstName": "Stewen",
"lastName": "Gold"
}
如上所示,型別 Info2
是型別 Info
的修改版本。請注意 Omit
型別中如何排除多個屬性。
使用 Omit
修改 TypeScript 中的屬性
TypeScript 允許使用者修改屬性。如果使用者嘗試修改沒有 Omit
型別的型別,則會引發錯誤。
例子:
type Info = {
firstName: string,
lastName: string,
age: number,
country: string,
};
type Info1 = Info & {
country: {
countryName: string,
cityName: string,
};
};
const info1: Info1 = {
firstName: 'John',
lastName: 'Nash',
age: 27,
country: {
countryName: 'China',
cityName: 'Wuhan',
},
}
此程式碼沒有輸出,因為物件 info1
屬性 country
將引發錯誤。錯誤訊息是:Type countryName and cityName is not assignable to type string
。
為了克服這個錯誤,使用者必須使用 omit
型別來修改型別。
例子:
type Info = {
firstName: string,
lastName: string,
age: number,
country: string,
};
type Info1 = Omit<Info, 'country'> & {
country: {
countryName: string,
cityName: string,
};
};
const info1: Info1 = {
firstName: 'John',
lastName: 'Nash',
age: 27,
country: {
countryName: 'China',
cityName: 'Wuhan'
},
}
console.log(info1);
輸出:
{
"firstName": "John",
"lastName": "Nash",
"age": 27,
"country": {
"countryName": "China",
"cityName": "Wuhan"
}
}
在上面的示例中,請注意 Info1
具有修改後的 country
型別。注意更新屬性的語法。
使用 Omit
型別是消除複雜性和提高工作效率的好習慣。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn