在 TypeScript 中使用 try..catch..finally 處理異常
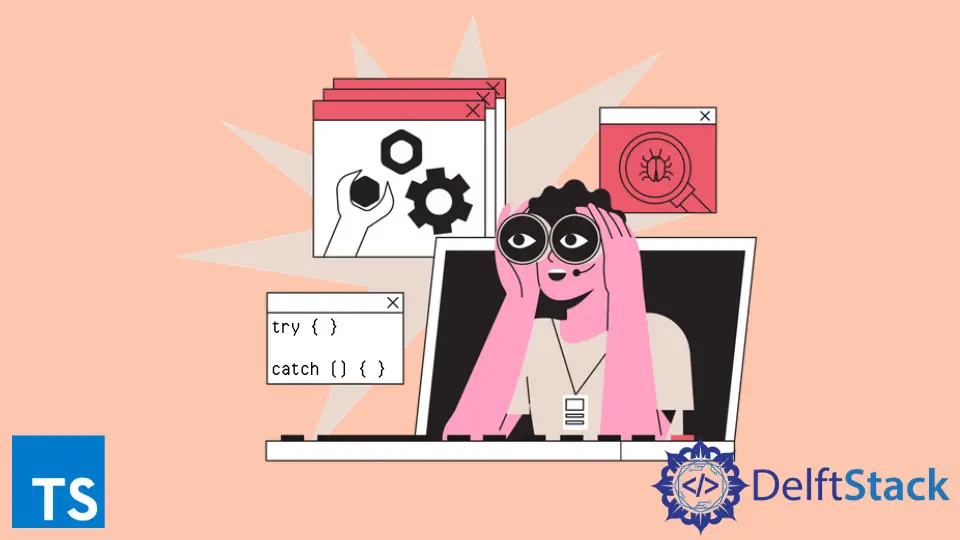
本教程將討論使用 try..catch..finally
語句在 TypeScript 中處理異常。
在 TypeScript 中處理異常
在 TypeScript 中,try..catch..finally
塊處理程式在執行時出現的異常。它讓程式正確執行,不會隨意結束。
可能出現異常的主要程式碼放在 try
塊內。如果發生異常,它會轉到處理它的 catch
塊;但是,如果沒有遇到錯誤,則會跳過 catch
塊。
在任何情況下,無論程式中是否出現錯誤,finally
塊都將始終執行。
下面是一些程式碼示例,說明我們如何在 TypeScript 中使用 try..catch..finally
進行異常處理。
function doOrThrow<T>(error: T): true{
if (Math.random() > .5){
console.log('true')
return true;
}
else{
throw error;
}
}
try{
doOrThrow('err1');
doOrThrow('err2');
doOrThrow('err3');
}
catch (e:any){
console.log(e,'error')
}
finally{
console.log("Terminated");
}
輸出:
try
塊內的函式被呼叫三次,它傳遞一個引數。如果生成的數字大於 0.5,它將執行 if
塊並返回 true
。
否則,它將丟擲一個錯誤,該錯誤在 catch
塊中處理。它告訴在執行 try
和 catch
之後它會丟擲哪個呼叫。
下面再考慮一個例子。
let fun: number; // Notice use of `let` and explicit type annotation
const runTask=()=>Math.random();
try{
fun = runTask();
console.log('Try Block Executed');
throw new Error("Done");
}
catch(e){
console.log("Error",e);
}
finally{
console.log("The Code is finished executing.");
}
輸出:
unknown
型別的 catch
子句變數
在 TypeScript 的 4.0 版
之前,catch
子句變數的型別是 any
。由於變數具有分配給它們的型別 any
,它們缺乏型別安全性,導致無效操作和錯誤。
現在,TypeScript 的 4.0 版
允許我們指定 catch
子句變數的型別 unknown
,這比型別 any
安全得多。
它提醒我們,在對值進行操作之前,我們需要執行某種型別的檢查。
try {
// ...
}
catch (err: unknown) {
// error!
// property 'toUpperCase' does not exist on 'unknown' type.
console.log(err.toUpperCase());
if (typeof err === "string") {
// works!
// We have narrowed 'err' down to the type 'string'.
console.log(err.toUpperCase());
}
}
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn