在 Ruby 中解析 JSON 字串
Nurudeen Ibrahim
2022年5月18日
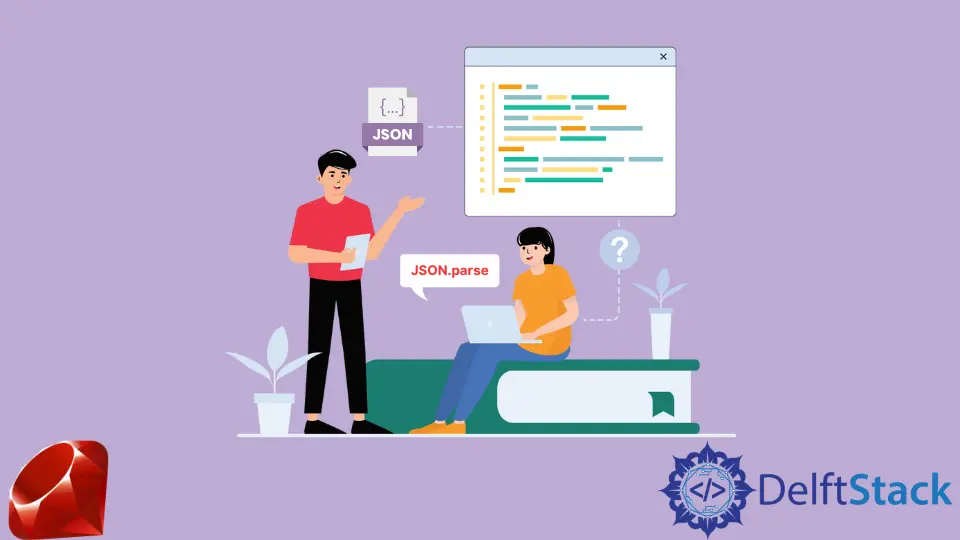
JSON(JavaScript Object Notation)是一種流行的資料交換格式,主要用於 Web 應用程式之間傳送和接收資料。在本教程中,我們將研究如何解析 JSON 字串。
JSON 值的常見示例可能是:
- 方括號括起來的值列表。例如
["Orange", "Apple", [1, 2]]
- 由花括號括起來的鍵/對的列表。例如
{ "name": "John", "sex": "male", "grades": [60, 70, 80, 90] }
這些 JSON 值有時以字串形式出現,例如,作為來自外部 API 的響應,並且總是需要將它們轉換成 Ruby 可以工作的形式。JSON
模組有一個 parse
方法允許我們這樣做。
示例程式碼:
require 'json'
json_string = '["Orange", "Apple", [1, 2]]'
another_json_string = '{ "name": "John", "sex": "male", "grades": [60, 70, 80, 90] }'
puts JSON.parse(json_string)
puts JSON.parse(another_json_string)
輸出:
["Orange", "Apple", [1, 2]]
{"name"=>"John", "sex"=>"male", "grades"=>[60, 70, 80, 90]}
為了使 JSON 模組在我們的程式碼中可用,我們首先需要該檔案,這就是我們程式碼的第一行所做的。
如上例所示,JSON.parse
將 json_string
轉換為 Ruby Array,並將 another_json_string
轉換為 Ruby Hash。
如果你有一個不是字串形式的 JSON 值,你可以在其上呼叫 to_json
以首先將其轉換為 JSON 字串,然後使用 JSON.parse
將其轉換為 Array 或 Hash 視情況而定.
示例程式碼:
require 'json'
json_one = ["Orange", "Apple", [1, 2]]
json_two = { "name": "John", "sex": "male", "grades": [60, 70, 80, 90] }
puts JSON.parse(json_one.to_json)
puts JSON.parse(json_two.to_json)
輸出:
["Orange", "Apple", [1, 2]]
{"name"=>"John", "sex"=>"male", "grades"=>[60, 70, 80, 90]}