如何在 Python 中運行 Bash 腳本
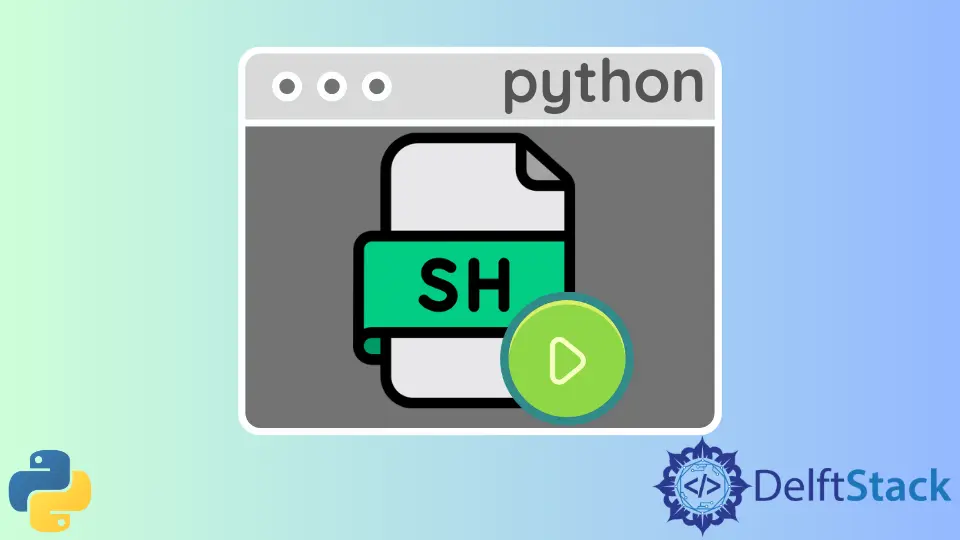
從 Python 執行 Bash 腳本可以顯著增強您的自動化能力並簡化工作流程。無論您是想將系統命令整合到應用程序中的開發人員,還是希望利用 Shell 功能的數據科學家,了解如何使用 Python 執行 Bash 腳本都是必不可少的。在本文中,我們將探討多種通過 Python 執行 Bash 腳本的方法,包括使用 subprocess 模組、os.system 等等。在本指南結束時,您將對如何在 Python 項目中無縫運行 Bash 命令和腳本有一個堅實的理解,使您能夠充分利用這兩種語言的強大功能。
方法 1: 使用 subprocess 模組
subprocess
模組是 Python 中一個強大的工具,允許您產生新進程,連接它們的輸入/輸出/錯誤管道,並獲取它們的返回代碼。這種方法在執行 Bash 腳本時是首選,因為它比其他方法提供了更多的控制和靈活性。
下面是一個使用 subprocess
模組執行 Bash 腳本的簡單示例:
import subprocess
# Define the command to execute
command = ["bash", "your_script.sh"]
# Execute the command
process = subprocess.run(command, capture_output=True, text=True)
# Print the output and error (if any)
print("Output:")
print(process.stdout)
print("Error:")
print(process.stderr)
輸出:
example of output
subprocess.run
函數執行指定的命令並等待其完成。capture_output=True
參數允許您捕獲輸出和錯誤消息,然後可以通過返回的進程對象的 stdout
和 stderr
屬性訪問這些消息。這種方法的優勢在於,它允許您通過檢查 stderr
輸出優雅地處理錯誤。此外,使用列表來定義命令有助於避免 Shell 注入漏洞。
方法 2: 使用 os.system
os.system
函數是從 Python 執行 Bash 腳本的另一種簡單方法。雖然它比 subprocess
更簡單使用,但它靈活性較差,且不提供對輸出或錯誤消息的直接訪問。
這裡有一個使用 os.system
運行 Bash 腳本的方法:
import os
# Define the command to execute
command = "bash your_script.sh"
# Execute the command
exit_code = os.system(command)
# Print the exit code
print("Exit Code:", exit_code)
輸出:
example of output
os.system
函數在一個子 Shell 中執行命令。它返回命令的退出代碼,您可以利用這個代碼來判斷腳本是否成功運行。然而,這種方法的一個限制是您無法直接捕獲執行腳本的輸出,這對於更複雜的任務來說可能是一個缺點。如果您需要處理輸出或錯誤,建議使用 subprocess
。
方法 3: 使用 subprocess.Popen
對於更高級的用例,subprocess.Popen
類提供了更大的控制權來執行 Bash 腳本。這種方法允許您在進程運行時與其互動,使其適用於長時間運行的腳本或需要實時輸入的腳本。
下面是一個使用 subprocess.Popen
執行 Bash 腳本的示例:
import subprocess
# Define the command to execute
command = ["bash", "your_script.sh"]
# Open the process
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
# Read output and error
stdout, stderr = process.communicate()
# Print the output and error
print("Output:")
print(stdout)
print("Error:")
print(stderr)
輸出:
example of output
在這種方法中,subprocess.Popen
啟動了進程,並允許您通過管道與其互動。communicate()
方法在進程完成後讀取輸出和錯誤消息。這種方法特別適用於長時間運行的腳本,因為它使您能夠實時處理輸出。此外,您可以根據需要向腳本提供輸入,這使得此方法具有高度的靈活性。
結論
總之,使用 Python 執行 Bash 腳本可以顯著增強您的編程能力,實現更大的自動化和效率。討論的三種方法——使用 subprocess
模組、os.system
和 subprocess.Popen
——各自具有獨特的優勢和用例。根據您的需求,您可以選擇最合適的方法來支持您的項目。通過掌握這些技術,您可以無縫地將 Bash 腳本集成到您的 Python 應用中,為自動化和系統管理開闢新的可能性。
常見問題
-
從 Python 執行 Bash 腳本的最佳方法是什麼?
最好的方法取決於您的具體需求。對於大多數情況,建議使用subprocess
模組,因為它具有靈活性和錯誤處理能力。 -
我可以在 Python 中捕獲 Bash 腳本的輸出嗎?
是的,您可以使用subprocess
模組通過設置capture_output=True
或使用subprocess.PIPE
與Popen
來捕獲輸出。 -
從 Python 執行 Bash 腳本是否安全?
是的,但要小心 Shell 注入漏洞。使用subprocess
時,將命令作為列表傳遞比作為單個字串更安全。 -
我可以從 Python 向我的 Bash 腳本傳遞參數嗎?
是的,您可以通過在使用subprocess
或os.system
時將其包含在命令列表中來傳遞參數。 -
如果我的 Bash 腳本需要用戶輸入怎麼辦?
您可以使用subprocess.Popen
與進程互動並根據需要提供輸入。