在 Python 中獲取長度不確定的輸入值
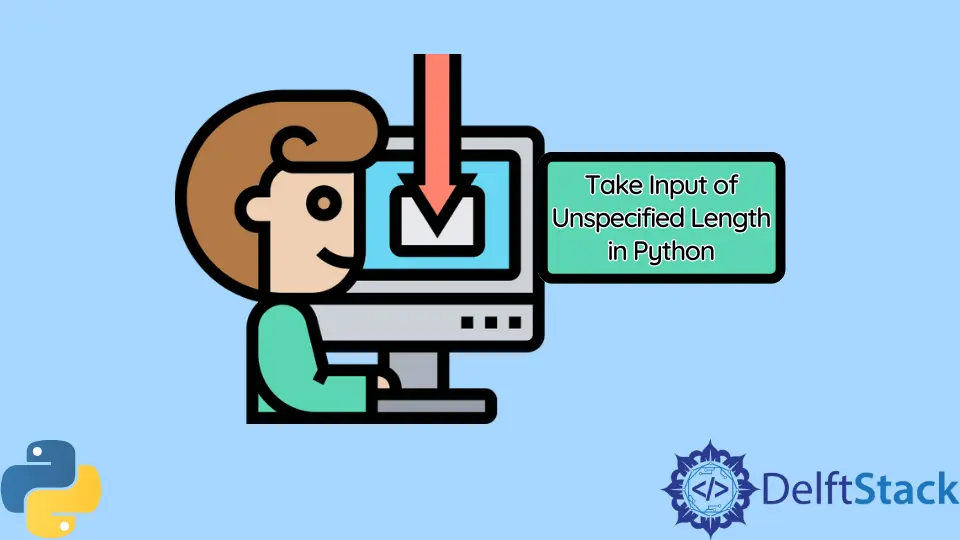
本文將介紹在輸入長度未知的情況下,我們如何在 Python 中獲取多個輸入。
在 Python 中使用 stdin
獲取未指定長度的輸入
要使用 sys
模組獲取輸入,我們可以使用 sys.stdin
。
Python stdin
代表標準輸入,它使我們能夠直接從命令列獲取輸入。在接受輸入時,stdin
在內部呼叫 input()
方法並在每一行之後新增一個 \n
。
例子:
import sys
print("Input something")
for x in sys.stdin:
if "end" == x.rstrip():
break
print(f"Either input something or input end to quit: {x}")
print("Exit")
輸出:
Input something
Pasta
Either input something or input end to quit: Pasta
Watermelon
Either input something or input end to quit: Watermelon
Banana
Either input something or input end to quit: Banana
Noodles
Either input something or input end to quit: Noodles
end
Exit
在這裡,我們首先匯入 sys
模組。然後,我們使用 for
迴圈來連續獲取輸入。
在這個 for
迴圈中,我們有一個 if
語句來檢查輸入是否等於字串 end
。如果是,那麼我們跳出迴圈。
請注意,我們可以指定任何字串,例如 quit
或 q
來代替 end
。我們必須告訴使用者輸入什麼才能退出。
請注意,我們還可以在此程式碼中輸入整數資料型別。但是,最好將輸入顯式更改為整數,因為 Python 會將每個輸入轉換為字串。
我們可以使用 int()
函式來執行此操作。
import sys
print("Give an input")
for val in sys.stdin:
if "end" == val.rstrip():
break
val = int(val)
print(f"Either input something or input end to quit: {val}")
print("Exit")
輸出:
Give an input
10
Either input something or input end to quit: 10
12
Either input something or input end to quit: 12
Dumbledore
Traceback (most recent call last):
File "tutorial.py", line 6, in <module>
x = int(x)
ValueError: invalid literal for int() with base 10: 'Dumbledore\n'
如上圖,我們只能輸入整數資料型別,否則會報錯。
在 Python 中使用 while
迴圈獲取未指定長度的輸入
當我們不知道必須接受輸入的次數時,我們可以使用 while
迴圈。
例子:
while True:
x = input("Grocery: ")
if x == "done":
break
輸出:
Grocery: Vinegar
Grocery: Soya sauce
Grocery: done
在這裡,我們使用 True
關鍵字來定義一個每次都會評估為真的條件。這樣,使用者不斷得到輸入提示。
但是,如果我們不定義中斷條件,這個程式將永遠不會終止。因此,在 while
塊中,我們定義了一個 break
條件,當它接收到值 done
時將把控制帶出迴圈。
請注意,我們可以使用諸如 quit
、bye
、0
等任何字串來代替字串 done
。
例子:
while True:
print("Input 0 to exit")
x = int(input("Enter the number: "))
if x == 0:
print("Bye")
break
輸出:
Input 0 to exit
Enter the number: 4
Input 0 to exit
Enter the number: 5
Input 0 to exit
Enter the number: 3
Input 0 to exit
Enter the number: 0
Bye
這個例子告訴使用者輸入 0
退出。除了在 while 迴圈中使用 True
關鍵字外,我們還可以指定一個評估為 true 的條件。
在 Python 中使用等價的 C++ cin
函式獲取未指定長度的輸入
讓我們在 Python 中建立與下面的 C++ 程式碼類似的輔助函式。
while (cin >> var) {
// code here
}
在 C++ 中,我們可以使用上述方法來獲取未指定長度的輸入。請注意,在 C++ 中,cin>>var
以兩種方式工作,作為輸入運算子和作為布林表示式。
在 Python 中,接受輸入的等價物是 var = input()
,它僅充當輸入通道。我們將使用一個名為 ‘var’ 的列表物件來模擬 Python 的 cin>>var
行為。
例子:
# Function that works like the cin function in C++
def cin(var):
try:
var[0] = int(input())
return True
except:
return False
# main function similar to that of C++
def main():
var = [0]
while cin(var):
print("Value:", var[0])
main()
輸出
5
Value: 5
7
Value: 7
3
Value: 3
9
Value: 9
6
Value: 6
4
Value: 4
>>>
首先,要知道 var
是一個列表物件。因此,如果我們在輔助方法中更改 var[0]
的值,更改將發生在 main
方法中。
在 cin
函式中,我們在變數 var[0]
中獲取輸入。此外,使用 try
語句,我們每次獲得整數輸入時都返回 True
。
請注意,如果我們按下 Enter 鍵而不是輸入,則上述程式碼中的程式碼將停止執行。不僅如此,輸入除整數以外的任何內容都會停止程式碼執行。
在 main
函式內部,我們不斷地使用 while
迴圈來呼叫 cin
函式。在此示例中,我們採用整數輸入,但我們可以根據需要進行更改。