如何使用索引為 Pandas DataFrame 中的特定單元格設定值
Puneet Dobhal
2023年1月30日
-
使用
pandas.dataframe.at
方法為 pandas DataFrame 中的特定單元格設定值 -
使用
Dataframe.set_value()
方法為 pandas DataFrame 中的特定單元格設定值 -
使用
Dataframe.loc
方法為 pandas DataFrame 中的特定單元格設定值
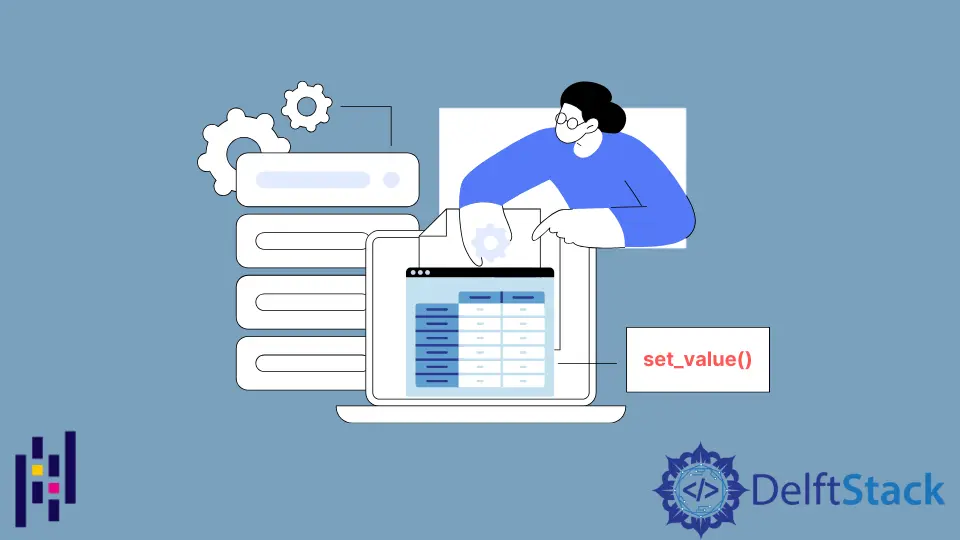
Pandas 是一個以資料為中心的 python 軟體包,它使 python 中的資料分析變得容易且一致。在本文中,我們將研究使用索引訪問和設定 pandas DataFrame 資料結構中特定單元格值的不同方法。
使用 pandas.dataframe.at
方法為 pandas DataFrame 中的特定單元格設定值
當需要在 DataFrame 中設定單個值時,主要使用 pandas.dataframe.at
方法。
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.at[0, "Col1"] = 99
sample_df.at[1, "Col2"] = 99
sample_df.at[2, "Col3"] = 99
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
輸出:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 99 20 30
1 11 99 31
2 15 25 99
你可能會注意到,在訪問單元格時,我們已將索引和列指定為 .at[0, 'Col1']
,其中第一個引數是索引,第二個引數是列。
如果你省略列而僅指定索引,則該索引的所有值都將被修改。
使用 Dataframe.set_value()
方法為 pandas DataFrame 中的特定單元格設定值
另一種選擇是 Dataframe.set_value()
方法。這與以前的方法非常相似,一次訪問一個值,但是語法略有不同。
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.set_value(0, "Col1", 99)
sample_df.set_value(1, "Col2", 99)
sample_df.set_value(2, "Col3", 99)
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
輸出:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 99 20 30
1 11 99 31
2 15 25 99
使用 Dataframe.loc
方法為 pandas DataFrame 中的特定單元格設定值
設定特定單元格的語法略有不同的另一種可行方法是 dataframe.loc
方法。
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.loc[0, "Col3"] = 99
sample_df.loc[1, "Col2"] = 99
sample_df.loc[2, "Col1"] = 99
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
輸出:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 10 20 99
1 11 99 31
2 99 25 35
本文中所有上述方法都是在 Pandas DataFrame
中修改或設定特定單元格的便捷方法,語法和規範上有微小差異。