如何在 Pandas 中遍歷 DataFrame 的行
- 使用 index 屬性來遍歷 Pandas DataFrame 中的行
-
loc[]
方法來遍歷 Python 中的 DataFrame 行 -
在 Python 中用
iloc[]
方法遍歷 DataFrame 行 -
pandas.DataFrame.iterrows()
遍歷 Pandas 行 - pandas.DataFrame.itertuples 遍歷 Pandas 行
-
##
pandas.DataFrame.apply
遍歷 Pandas 行
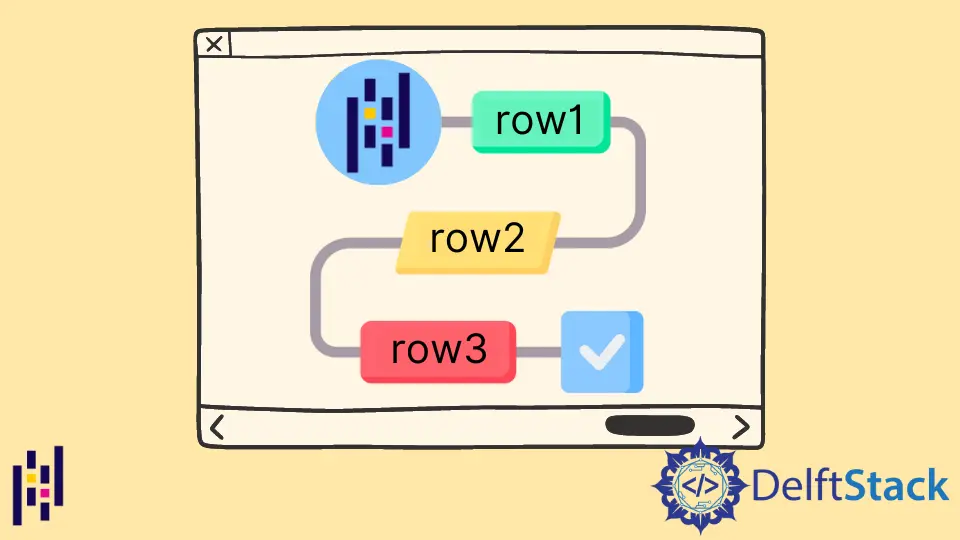
我們可以使用 DataFrame 的 index 屬性遍歷 Pandas DataFrame 的行。我們還可以使用 DataFrame 物件的 loc()
,iloc()
,iterrows()
,itertuples()
,iteritems()
和 apply()
方法遍歷 Pandas DataFrame 的行。
在以下各節中,我們將使用以下 DataFrame
作為示例。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
print(df)
輸出:
Date Income_1 Income_2
0 April-10 10 20
1 April-11 20 30
2 April-12 10 10
3 April-13 15 5
4 April-14 10 40
5 April-16 12 13
使用 index 屬性來遍歷 Pandas DataFrame 中的行
Pandas DataFrame 的 index 屬性提供了從 DataFrame 的頂行到底行的範圍物件。我們可以使用範圍來迭代 Pandas 中的行。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for i in df.index:
print(
"Total income in "
+ df["Date"][i]
+ " is:"
+ str(df["Income_1"][i] + df["Income_2"][i])
)
輸出:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
它將每行的 Income_1
和 Income_2
相加並列印總收入。
loc[]
方法來遍歷 Python 中的 DataFrame 行
loc[]
方法用於一次訪問一行。當我們在遍歷 DataFrame 的迴圈中使用 loc[]
方法時,我們可以遍歷 DataFrame 的行。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for i in range(len(df)):
print(
"Total income in "
+ df.loc[i, "Date"]
+ " is:"
+ str(df.loc[i, "Income_1"] + df.loc[i, "Income_2"])
)
輸出:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
在這裡,range(len(df))
生成一個範圍物件以遍歷 DataFrame 中的整個行。
在 Python 中用 iloc[]
方法遍歷 DataFrame 行
Pandas DataFrame 的 iloc 屬性也非常類似於 loc 屬性。loc 和 iloc 之間的唯一區別是,在 loc 中,我們必須指定要訪問的行或列的名稱,而在 iloc 中,我們要指定要訪問的行或列的索引。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for i in range(len(df)):
print(
"Total income in " + df.iloc[i, 0] + " is:" + str(df.iloc[i, 1] + df.iloc[i, 2])
)
輸出:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
這裡的索引 0
代表 DataFrame 的第一列,即 Date
,索引 1
代表 Income_1
列,索引 2
代表 Income_2
列。
pandas.DataFrame.iterrows()
遍歷 Pandas 行
pandas.DataFrame.iterrows()
返回的索引該行以及該行的整個資料為系列。因此,我們可以使用此函式在 Pandas DataFrame 中的行上進行迭代。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for index, row in df.iterrows():
print(
"Total income in "
+ row["Date"]
+ " is:"
+ str(row["Income_1"] + row["Income_2"])
)
輸出:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
pandas.DataFrame.itertuples 遍歷 Pandas 行
pandas.DataFrame.itertuples
返回一個物件,以使用第一個欄位作為索引,其餘欄位作為列值。因此,我們還可以使用此函式在 Pandas DataFrame 中的行上進行迭代。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for row in df.itertuples():
print("Total income in " + row.Date + " is:" + str(row.Income_1 + row.Income_2))
輸出:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
##pandas.DataFrame.apply
遍歷 Pandas 行
pandas.DataFrame.apply
返回一個 DataFrame
沿 DataFrame 的給定軸應用給定函式的結果。
語法:
DataFrame.apply(self, func, axis=0, raw=False, result_type=None, args=(), **kwds)
其中,func
代表要應用的函式,而 axis
代表應用函式的軸。我們可以使用 axis = 1
或 axis ='columns'
將函式應用於每一行。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
print(
df.apply(
lambda row: "Total income in "
+ row["Date"]
+ " is:"
+ str(row["Income_1"] + row["Income_2"]),
axis=1,
)
)
輸出:
0 Total income in April-10 is:30
1 Total income in April-11 is:50
2 Total income in April-12 is:20
3 Total income in April-13 is:20
4 Total income in April-14 is:50
5 Total income in April-16 is:25
dtype: object
此處,lambda
關鍵字用於定義應用於每行的行內函數。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn相關文章 - Pandas DataFrame
- 如何將 Pandas DataFrame 列標題獲取為列表
- 如何刪除 Pandas DataFrame 列
- 如何在 Pandas 中將 DataFrame 列轉換為日期時間
- 如何在 Pandas DataFrame 中將浮點數轉換為整數
- 如何按一列的值對 Pandas DataFrame 進行排序
- 如何用 group-by 和 sum 獲得 Pandas 總和