PowerShell 中的返回值
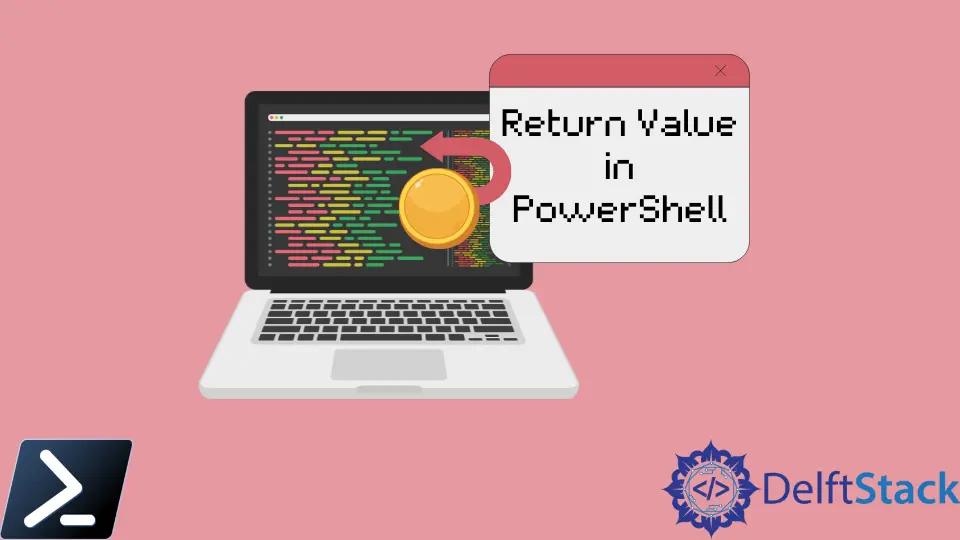
通常,return
關鍵字退出函式、指令碼或指令碼塊。因此,例如,我們可以使用它在特定點離開作用域、返回值或指示作用域的末端已經到達。
但是,在 Windows PowerShell 中,使用 return
關鍵字可能會有些混亂,因為你的指令碼可能會列印出你可能沒有預料到的輸出。
本文將討論 return
關鍵字的工作原理以及如何在 Windows PowerShell 中正確使用它們。
在 PowerShell 中使用 return
關鍵字
下面的指令碼塊是 return
關鍵字語法的基本形式。
return <expression>
return
關鍵字可以單獨出現,也可以在其後出現值或表示式。單獨的 return
關鍵字會將命令列返回到其先前的呼叫點。
return
return $a
return (1 + $a)
如果在特定點滿足條件,下面的示例使用 return
關鍵字退出函式。在此示例中,奇數不相乘,因為 return 語句先於該語句執行。
function MultiplyOnlyEven
{
param($num)
if ($num % 2) { return "$num is not even" }
$num * 2
return
}
1..10 | ForEach-Object {MultiplyOnlyEven -Num $_}
輸出:
1 is not even
4
3 is not even
8
5 is not even
12
7 is not even
16
9 is not even
20
從更本機程式設計的角度來看,Windows PowerShell 具有令人困惑的返回語義。我們需要考慮兩個主要思想:
- 所有輸出都被捕獲並返回。
- return 關鍵字表示一個邏輯退出點。
話雖如此,以下幾個指令碼塊將返回 $a
變數的值。
使用表示式返回關鍵字:
$a = "Hello World"
return $a
返回不帶表示式的關鍵字:
$a = "Hello World"
$a
return
在第二個指令碼塊中也不需要 return
關鍵字,因為在命令列中呼叫變數將顯式返回該變數。
PowerShell 管道中的返回值
當你從指令碼塊或函式返回值時,Windows PowerShell 會自動彈出成員並通過管道一次一個地推送它們。此用例背後的原因是由於 Windows PowerShell 的一次處理。
下面的函式演示了這個想法將返回一個數字陣列。
function Test-Return
{
$array = 1,2,3
return $array
}
Test-Return | Measure-Object | Select-Object Count
輸出:
Count
-----
3
使用 Test-Return
cmdlet 時,以下函式的輸出通過管道傳送到 Measure-Object
cmdlet。該 cmdlet 將計算管道中物件的數量,並且在執行時,返回的計數為 3。
要使指令碼塊或函式僅將單個物件返回到管道,請使用以下方法之一:
在 PowerShell 中使用一元陣列表示式
使用一元表示式可以將你的返回值作為單個物件傳送到管道中,如以下示例所示。
function Test-Return
{
$array = 1,2,3
return (,$array)
}
Test-Return | Measure-Object | Select-Object Count
輸出:
Count
-----
1
在 PowerShell 中使用 Write-Output
和 NoEnumerate
引數
我們還可以將 Write-Output
cmdlet 與 -NoEnumerate
引數一起使用。下面的示例使用 Measure-Object
cmdlet 對通過 return
關鍵字從示例函式傳送到管道的物件進行計數。
示例程式碼:
function Test-Return
{
$array = 1,2,3
return Write-Output -NoEnumerate $array
}
Test-Return | Measure-Object | Select-Object Count
輸出:
Count
-----
1
在 PowerShell 版本 5 中引入了另一種強制管道僅返回單個物件的方法,我們將在本文的下一節中討論。
在 PowerShell 5 中定義類
使用 Windows PowerShell 5.0 版,我們現在可以建立和定義我們的自定義類。將你的函式更改為一個類,return
關鍵字將只返回緊接在它之前的單個物件。
示例程式碼:
class test_class {
[int]return_what() {
Write-Output "Hello, World!"
return 1000
}
}
$tc = New-Object -TypeName test_class
$tc.return_what()
輸出:
1000
如果上面的類是一個函式,它將返回管道中所有儲存的值。
輸出:
Hello World!
1000
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn