如何在 PowerShell 中使用 Read-Host 設置預設值
-
PowerShell 中
Read-Host
命令概述 -
使用條件語句在 PowerShell 中的
Read-Host
命令設置預設值 -
使用函數在 PowerShell 中的
Read-Host
命令設置預設值 -
使用 Do-While 循環在 PowerShell 中的
Read-Host
命令設置預設值 - 結論
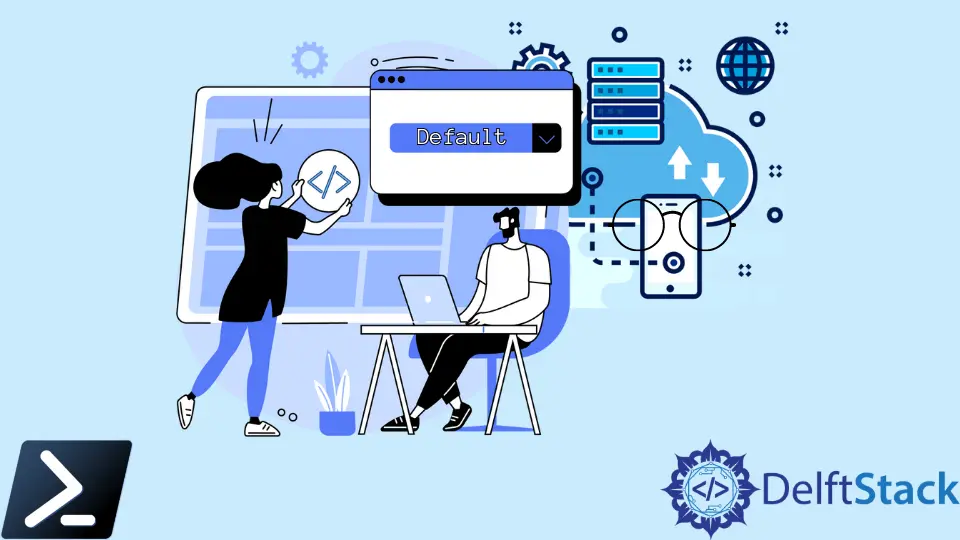
PowerShell 是一個強大的腳本語言,提供了多種與用戶互動的方式。一種常見的方法是使用 Read-Host
來提示用戶輸入。
然而,在某些情況下,提供預設值是有益的。在本指南中,我們將探討在 PowerShell 中使用 Read-Host
結合預設值的不同方法。
PowerShell 中 Read-Host
命令概述
Read-Host
是 PowerShell 中一個基本的 cmdlet,允許腳本通過提示用戶輸入來與其互動。這是一個用於在腳本執行期間收集信息的多功能工具。這個 cmdlet 在需要動態或特定用戶信息的情況下特別有用。
語法:
Read-Host [-Prompt] <String> [-AsSecureString] [-Credential <PSCredential>] [-ErrorAction <ActionPreference>] [-Timeout <Int32>] [-OutVariable <String>] [-OutBuffer <Int32>] [-InputObject <PSObject>] [<CommonParameters>]
在這裡,關鍵參數包括:
-Prompt
:指定將顯示給用戶的消息。-AsSecureString
:請求作為安全字符串(密碼)輸入。-Credential
:提示輸入用戶名和密碼。返回一個PSCredential
對象。-ErrorAction
:定義如果出現錯誤時要採取的行動。-Timeout
:設置 cmdlet 等待輸入的時間限制。-OutVariable
:將結果存儲在變量中。-OutBuffer
:決定在輸出之前緩衝的對象數量。-InputObject
:允許從管道輸入。
Read-Host
命令的基本用法
$userInput = Read-Host "Please enter your name"
在此示例中,用戶將收到消息“請輸入您的姓名”的提示。他們輸入的值將存儲在變量 $userInput
中。
安全字符串輸入
$securePassword = Read-Host "Please enter your password" -AsSecureString
此命令提示用戶輸入密碼,並將輸入存儲為安全字符串在變量 $securePassword
中。
超時功能
$userInput = Read-Host "Please enter your choice" -Timeout 10
此命令提示用戶輸入,並將等待最多 10
秒。如果在該時間內未提供輸入,變量 $userInput
將為空。
你可以使用條件語句、函數、循環或 try-catch 區塊與 Read-Host
結合來納入預設值。
使用條件語句在 PowerShell 中的 Read-Host
命令設置預設值
創建一個名為 defaultName
的變量,並賦予其字符串值 John Doe
。如果用戶選擇不提供輸入,則將使用此值。
$defaultName = "John Doe"
定義一個新變量 userName
,它將產生一條消息,要求用戶輸入並利用 Read-Host
命令執行此操作。
$userName = Read-Host ("Please enter your name (default is {0})" -f $defaultName)
以下代碼實施一個條件以檢查用戶是否提供了任何輸入。它檢查變量 $userName
是否為 null 或空字符串。
如果用戶提供了某些輸入(不是空字符串),則會將 $defaultName
的值更新為用戶的輸入。
if (-not $userName) { $userName = $defaultName }
完整代碼:
$defaultName = "John Doe"
$userName = Read-Host ("Please enter your name (default is {0})" -f $defaultName)
if (-not $userName) { $userName = $defaultName }
Write-Host "Hello, $($userName)!"
輸出:
在下一種方法中,我們將接收用戶輸入的過程放置在檢查用戶是否輸入了任何值的條件內。這使我們能夠更好地追踪用戶是否提供了任何數據。
如果用戶沒有任何行動,我們使用預設值 John Doe
。但是,如果用戶提供輸入,我們將使用存儲在 userName
變量中的值。
代碼:
$defaultName = "John Doe"
if (($userName = Read-Host "Press enter to accept default value $defaultName") -eq '') { $defaultName } else { $userName }
輸出:
最後一種方法驗證了用戶是否輸入了信息,如果有,則將用戶輸入的值直接賦給名為 defaultName
的變量。
代碼:
$defaultName = "John Doe"
if ($userName = Read-Host "Value [$defaultName]") { $defaultName = $userName }
輸出:
這樣,它就不必使用 else
條件。
使用函數在 PowerShell 中的 Read-Host
命令設置預設值
函數封裝了一組可以在腳本中重複使用的指令。我們將設計一個名為 Read-HostWithDefault
的函數,該函數將納入預設值。
function Read-HostWithDefault {
param (
[string]$prompt,
[string]$default
)
$userInput = Read-Host "$prompt (default is $default)"
$userInput = if ($userInput -ne "") { $userInput } else { $default }
return $userInput
}
解釋:
function Read-HostWithDefault { ... }
:這定義了一個名為Read-HostWithDefault
的函數。param ( [string]$prompt, [string]$default )
:這指定了函數期望的參數。在這個案例中,它是提示消息和一個預設值。$userInput = Read-Host "$prompt (default is $default)"
:這一行提示用戶輸入,顯示提示和預設值。輸入將存儲在變量$userInput
中。$userInput = if ($userInput -ne "") { $userInput } else { $default }
:這一行檢查$userInput
是否不是空字符串($userInput -ne ""
)。如果此條件為真(意味著用戶提供了輸入),則$userInput
保留其值。如果為假(意味著用戶沒有提供輸入),則將$default
指派給$userInput
。return $userInput
:最後,函數返回結果值。
現在,讓我們看看如何在實際場景中使用這個函數:
$defaultName = "John Doe"
$name = Read-HostWithDefault -prompt "Enter your name" -default $defaultName
Write-Host "Hello, $name!"
解釋:
$defaultName = "John Doe"
:在這裡,我們為用戶的姓名設置了預設值。$name = Read-HostWithDefault -prompt "Enter your name" -default $defaultName
:這一行提示用戶輸入其姓名,顯示所提供的提示消息和預設值。函數Read-HostWithDefault
通過適當的參數被調用。Write-Host "Hello, $name!"
:這會生成一個問候語,該問候語可以是用戶提供的,也可以是預設值。
輸出:
使用 Do-While 循環在 PowerShell 中的 Read-Host
命令設置預設值
do-while
循環是 PowerShell 中的一個控制結構,它重複執行一段代碼塊,只要指定的條件為真。這在確保至少執行某個操作一次時特別有用。
do-while
循環的基本語法是:
do {
# Code to be executed
} while (condition)
do
語句中的代碼塊將繼續執行,只要 while
關鍵字後面的條件為真。
讓我們考慮一個示例,我們希望提示用戶輸入其姓名,但如果他們不輸入任何內容,則提供預設值 "John Doe"
:
$defaultName = "John Doe"
do {
$userName = Read-Host "Please enter your name (default is $defaultName)"
$userName = if ($userName) { $userName } else { $defaultName }
} while (-not $userName)
Write-Host "Hello, $userName!"
解釋:
$defaultName = "John Doe"
:這一行設置了如果用戶不提供任何輸入時要使用的預設值。do { ... } while (-not $userName)
:這啟動了一個do-while
循環。在do
語句中的代碼塊至少會執行一次。條件(-not $userName)
確保循環在$userName
未提供的情況下繼續。$userName = Read-Host "Please enter your name (default is $defaultName)"
:這提示用戶輸入其姓名,並在消息中顯示預設值。$userName = if ($userName) { $userName } else { $defaultName }
:這一行使用if-else
語句來確定$userName
的值。如果$userName
有值(即它不是空或 null),則保持該值。否則,它將預設名稱$defaultName
指派給$userName
。-not $userName
:這個條件檢查$userName
是否為空或未提供。如果它是空的,則循環將繼續,提示用戶再次輸入。- 一旦用戶提供輸入,條件
-not $userName
變為假,循環終止。
輸出:
結論
在本綜合指南中,我們探討了在 PowerShell 中使用 Read-Host
命令設置預設值的各種方法。每種方法根據您的腳本的具體要求提供了不同的優勢。
- 條件語句:此方法允許對用戶輸入進行簡單檢查,並在處理不同場景時提供靈活性。
- 函數:通過封裝邏輯在函數中,您可以創建具有無縫集成預設值的可重用代碼。
- Do-While 循環:這種方法確保至少執行某個操作一次,非常適合需要用戶輸入的場景。
通過理解和應用這些技術,您可以增強 PowerShell 腳本的用戶體驗和韌性。記住選擇最符合您的具體腳本需求的方法。
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn