在 Windows PowerShell 中逐行讀取檔案
Marion Paul Kenneth Mendoza
2023年1月30日
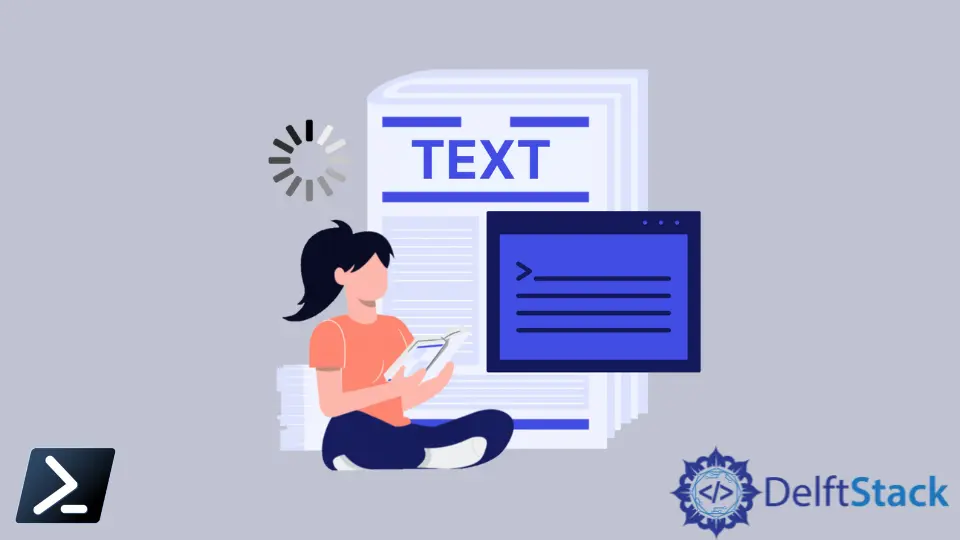
在 Windows PowerShell 中,我們可以使用 Get-Content
cmdlet 從檔案中讀取檔案。但是,該 cmdlet 會立即將整個檔案內容載入到記憶體中,這會導致大檔案失敗或凍結。
為了解決這個問題,我們可以做的是我們可以逐行讀取檔案,本文將向你展示如何。
在開始之前,最好建立一個包含多行的示例文字檔案。例如,我建立了一個包含以下行的 file.txt
。
file.txt
:
one
two
three
four
five
six
seven
eight
nine
ten
根據微軟的說法,正規表示式是一種用於匹配文字的模式。它可以由文字字元、運算子和其他結構組成。本文將嘗試不同的指令碼,其中涉及一個稱為正規表示式的標準變數,用 $regex
表示。
改進 Get-Content
Cmdlet 語法
我們最初討論過 Get-Content
cmdlet 在我們載入大檔案時存在缺陷,因為它會將檔案的內容一次全部載入到記憶體中。但是,我們可以通過使用 foreach
迴圈逐行載入來改進它。
示例程式碼:
foreach($line in Get-Content .\file.txt) {
if($line -match $regex){
Write-Output $line
}
}
使用 switch
載入特定行
我們可以使用 $regex
變數通過建立自定義 $regex
值並在 switch case 函式中匹配它來輸入檔案中的特定行。
示例程式碼:
$regex = '^t'
switch -regex -file file.txt {
$regex { "line is $_" }
}
輸出:
line is two
line is three
line is ten
在這個例子中,我們定義了一個自定義值 $regex
,它只匹配並顯示以字元 t
開頭的行。
使用 PowerShell 中的 [System.IO.File]
類逐行讀取檔案
如果你有權訪問 .NET 庫,則可以使用 [System.IO.File]
類從檔案中讀取內容。它是 Get-Content
的絕佳替代品,因為你不需要正規表示式來逐行處理檔案。
示例程式碼:
foreach($line in [System.IO.File]::ReadLines("C:\yourpathhere\file.txt"))
{
Write-Output $line
}
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn