如何在 PowerShell 中創建並拋出新異常
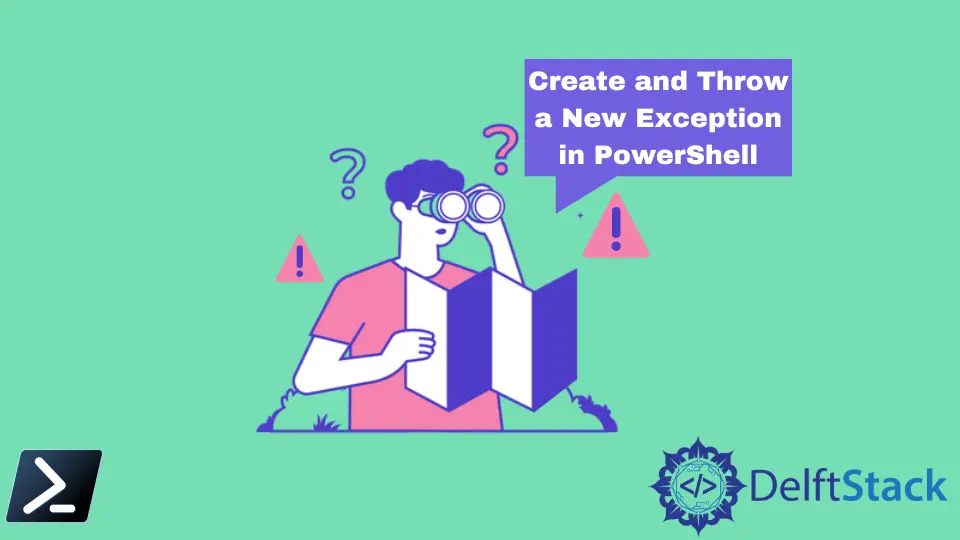
異常處理是編寫穩健和可靠的 PowerShell 腳本的關鍵方面。通過創建和拋出異常,您可以有效地傳達在腳本執行過程中可能發生的錯誤和問題。
雖然 PowerShell 提供了幾種類型的內建異常,但在某些情況下,您需要創建和拋出自定義異常,以根據腳本的特定需求量身定制錯誤處理。
在本文中,我們將探討在 PowerShell 中創建和拋出自定義異常的過程,並提供詳細的示例和輸出。
創建自定義異常類
在深入自定義異常之前,讓我們簡要回顧一下編程中異常的概念。
異常是打斷程序正常流程的意外事件或錯誤。當發生異常時,控制權將轉移到指定的異常處理代碼塊,允許進行優雅的錯誤管理。
PowerShell 提供了多種內建異常類型,例如 System.Management.Automation.CmdletInvocationException
、System.IO.IOException
和 System.Exception
,以處理常見錯誤。然而,當您遇到特定錯誤情況或想向用戶提供清晰且具資訊性的錯誤消息時,創建自定義異常就非常有益。
要在 PowerShell 中創建自定義異常,您首先需要定義一個繼承自 [System.Exception]
基類的新異常類。然後,您可以添加屬性和方法來自定義異常的行為。
讓我們通過一個實際的示例來說明這一點:
class MyCustomException : [System.Exception] {
MyCustomException() {
}
MyCustomException([string] $message) {
$this.Message = $message
}
[string] GetMessage() {
return "Custom Exception: $($this.Message)"
}
}
在這個示例中,我們創建了一個名為 MyCustomException
的自定義異常類。MyCustomException
類繼承自 [System.Exception]
基類。
然後,定義了兩個構造函數:一個不帶參數的默認構造函數和另一個接受自定義錯誤消息的構造函數。
最後,我們包括了一個 GetMessage
方法來返回格式化的錯誤消息。此方法在處理異常時提供具體細節時非常有用。
使用 throw
語句來拋出自定義異常
一旦您定義了自定義異常類,就可以使用 throw
語句在腳本中拋出它。為此,創建自定義異常的實例並傳遞相關的錯誤消息。
以下是一個演示如何拋出自定義異常的示例:
try {
# Simulate code that may cause an error
$result = 1 / 0
}
catch {
$errorMessage = "An error occurred: $($_.Exception.Message)"
throw [MyCustomException]::new($errorMessage)
}
在 try
塊中,我們故意通過嘗試除以零來創建一個錯誤。
在 catch
塊中,我們捕獲錯誤,創建一個自定義錯誤消息,然後拋出一個包含此錯誤消息的 MyCustomException
實例。
處理自定義異常
處理自定義異常與處理 Built-in 異常在 PowerShell 中是相似的。您可以使用 try
、catch
和 finally
塊來優雅地管理錯誤。
以下是一個演示捕獲和處理自定義異常的示例:
try {
# Simulate code that may cause an error
$result = 1 / 0
}
catch [MyCustomException] {
Write-Host "Custom Exception Caught: $($_.Exception.Message)"
}
catch {
Write-Host "Other Exception Caught: $($_.Exception.Message)"
}
在這段代碼中,我們在 try
塊中嘗試除以零。第一個 catch
塊專門捕獲 MyCustomException
並顯示一條消息,指示捕獲到了自定義異常,並顯示我們提供的自定義錯誤消息。
如果發生任何其他異常,第二個 catch
塊作為通用異常處理程序將捕獲它並顯示其他消息。
讓我們執行該腳本並查看輸出:
Custom Exception Caught: An error occurred: Attempted to divide by zero.
在這個示例中,我們故意通過嘗試除以零來觸發一個錯誤。MyCustomException
類的 catch
塊捕獲了錯誤並顯示一條消息,說明 Custom Exception Caught
。
此外,它提供了自定義錯誤消息:An error occurred: Attempted to divide by zero
。如果發生其他異常,它們將被通用 catch
塊捕獲,但這在本示例中不適用。
這裡是完整的 PowerShell 代碼,用於創建和拋出自定義異常,以及如何處理它的示例:
class MyCustomException : [System.Exception] {
MyCustomException() {
}
MyCustomException([string] $message) {
$this.Message = $message
}
[string] GetMessage() {
return "Custom Exception: $($this.Message)"
}
}
try {
# Simulate code that may cause an error (division by zero)
$result = 1 / 0
}
catch {
$errorMessage = "An error occurred: $($_.Exception.Message)"
throw [MyCustomException]::new($errorMessage)
}
try {
# Simulate code that may cause another error (e.g., accessing a non-existent variable)
$nonExistentVariable = $undefinedVariable
}
catch [MyCustomException] {
Write-Host "Custom Exception Caught: $($_.Exception.Message)"
}
catch {
Write-Host "Other Exception Caught: $($_.Exception.Message)"
}
此代碼將輸出異常處理的結果,區分自定義異常和其他異常。
結論
在 PowerShell 中創建和拋出自定義異常使您能夠提供更具資訊性的錯誤消息,並增強腳本的可靠性和可維護性。這些自定義異常幫助您根據腳本特定需求量身定制錯誤處理,確保用戶體驗更加順暢,提高故障排除能力。