在 PowerShell 中查詢子字串的位置
- PowerShell 中的字串和子字串
-
在 PowerShell 中使用
IndexOf
方法查詢子字串的位置 -
在 PowerShell 中使用
LastIndexOf
方法查詢子字串的位置 -
在 PowerShell 中使用
Select-String
Cmdlet 查詢子字串的位置
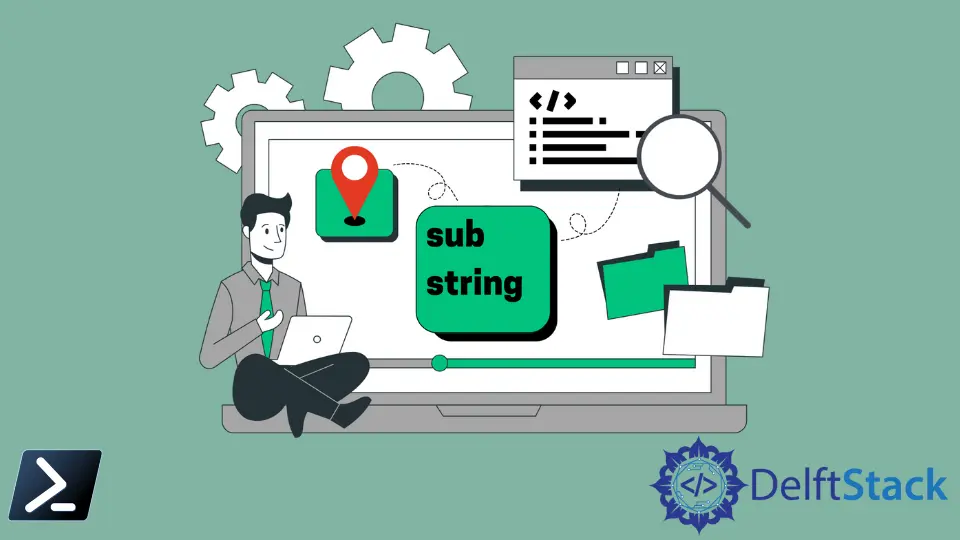
本教程將介紹在 PowerShell 中查詢子字串位置的不同方法。
PowerShell 中的字串和子字串
字串是 PowerShell 中使用的常見資料型別。它是表示文字的字元序列。
你可以使用單引號或雙引號在 PowerShell 中定義字串。例如,ABC
或 ABC
。
子字串是字串中的任何特定文字。如果 ABC
是一個字串,B
是它的子字串。
有時,你可能需要查詢字串的某個部分或文字檔案中子字串的位置。IndexOf
方法和 LastIndexOf
主要報告指定子字串在 PowerShell 中的索引位置。
在 PowerShell 中使用 IndexOf
方法查詢子字串的位置
IndexOf
方法允許你在字串物件中查詢指定字串的第一次出現。
在此方法中,你可以指定字串、字串物件中的起始搜尋位置、要搜尋的字元數以及用於搜尋的規則型別。
下面的示例查詢給定字串中第一次出現子字串 to
的位置。
"Welcome to PowerShell Tutorial".IndexOf("to")
輸出:
8
IndexOf
方法從零開始計算索引,列印輸出 8
。
在下面的程式碼中,9
被指定為字串中的起始搜尋位置。
"Welcome to PowerShell Tutorial".IndexOf("to", 9)
當你指定開始搜尋位置時,該位置之前的字元將被忽略,因此它會在索引 9
之後查詢子字串 to
第一次出現的位置。
輸出:
24
如果指定的字串不匹配,則返回值 -1
。
例如,在給定字串中搜尋子字串 py
會列印結果 -1
。
"Welcome to PowerShell Tutorial".IndexOf("py")
輸出:
-1
在 PowerShell 中使用 LastIndexOf
方法查詢子字串的位置
你還可以使用 LastIndexOf
方法在 PowerShell 中查詢子字串的索引。LastIndexOf
方法查詢字串中某個字元最後一次出現的位置。
以下命令查詢給定字串中最後一次出現的子字串 to
的索引。
"Welcome to PowerShell Tutorial".LastIndexOf("to")
如圖所示,它列印子字串 to
最後出現的位置。
輸出:
24
讓我們看另一個帶有字串變數 $hello
的示例。
$hello="HelloHelloHello"
如果你使用 LastIndexOf
方法在 $hello
中查詢子字串 He
,它會返回 10
,因為它是出現 He
的最後一個索引。
$hello.LastIndexOf("He")
輸出:
10
如果子字串不匹配,它也返回 -1
。
例如:
$hello.LastIndexOf("Hi")
輸出:
-1
在 PowerShell 中使用 Select-String
Cmdlet 查詢子字串的位置
Select-String
cmdlet 在字串和檔案中搜尋文字。它類似於 UNIX 中的 grep
。
預設情況下,它會在每一行中找到第一個匹配項並顯示包含該匹配項的所有文字。
$hello | Select-String "He"
輸出:
HelloHelloHello
Select-String
的 Matches
屬性查詢指定字串與輸入字串或檔案之間的匹配。匹配子字串的位置儲存在 Index
屬性中。
下面的示例展示瞭如何使用 Matches.Index
屬性來查詢字串中子字串的位置。
("HelloHelloHello" | Select-String "He").Matches.Index
它只列印索引 0
,因為 Select-String
在每行文字的第一個匹配之後停止。
輸出:
0
你可以使用 -AllMatches
引數在每一行中搜尋多個匹配項。
("HelloHelloHello" | Select-String "He" -AllMatches).Matches.Index
如圖所示,它列印指定子字串的所有位置。
輸出:
0
5
10
以下示例在文字檔案 test.txt
中查詢指定子字串的所有位置。
(Get-Content test.txt | Select-String "the").Matches.Index
輸出:
10
47
17
此方法是查詢字串中所有字元位置的最佳選擇。
我們希望本文能幫助你瞭解如何在 PowerShell 中查詢子字串的索引位置。