在 PowerShell 中的位元組陣列
- 在 PowerShell 中將數據轉換為字節數組
- 在 PowerShell 5+ 中將數據轉換為字節數組
- 在 PowerShell 7+ 中將數據轉換為字節數組
- 在 PowerShell 中將字節數組轉換為字符串
- 在 PowerShell 中將字符串轉換為字節數組
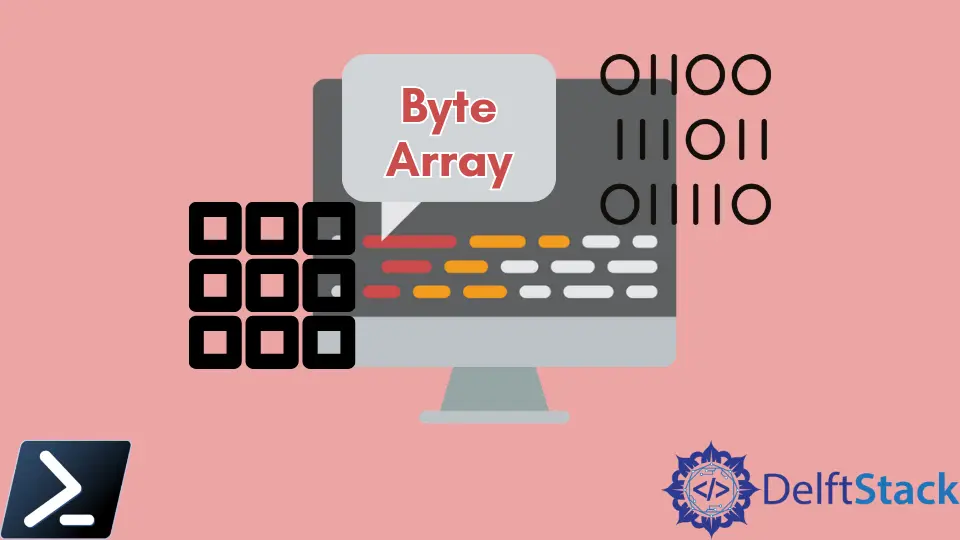
本文旨在演示如何使用 PowerShell 腳本 API 將各種形式的數據轉換為字節數組。
在 PowerShell 中將數據轉換為字節數組
在自動化任務和處理數據時,數據可能需要以特定方式進行處理,以提取有用信息或以有意義的方式修改數據。在 PowerShell 中處理這種數據有時可能變得不便,尤其是在另一種數據類型中。
為了使處理更輕鬆或減少計算次數,在某些情況下,將數據轉換為字節數組並在其上執行操作而不是原始數據可能是理想的。
考慮以下代碼:
$data = Get-Content "a.exe"
Get-Item "a.exe" | Format-List -Property * -Force
Write-Host "File Datatype: "$data.GetType().Name
這將產生以下輸出:
< Output redacted >
.
.
.
.
BaseName : a
Target :
LinkType :
Length : 400644
.
.
.
Extension : .exe
Name : a.exe
Exists : True
.
.
.
.
.
Attributes : Archive
File Datatype: Object[]
從上面的輸出中,我們打開的文件是一個 Windows 可執行文件 (exe
)。在使用 Get-Item
和 Format-List
cmdlet 查看其屬性後,可以獲得關於該文件的有意義的見解,特別是大小,這在迭代過程中可以證明非常有用。
這種情況的一個不利方面是,使用 Get-Content
cmdlet 獲取的文件數據返回的是 Object[]
。
讓我們看看如何將其轉換為 Byte[]
以便進行場景相關的操作。
在 PowerShell 5+ 中將數據轉換為字節數組
考慮以下代碼:
$file = "a.exe"
[byte[]]$data = Get-Content $file -Encoding Byte
Write-Host "File Datatype: "$data.GetType().Name
for ($i = 0; $i -lt 10; $i++) { Write-Host $data[$i] }
這將產生以下輸出:
File Datatype: Byte[]
Byte No 0 : 77
Byte No 1 : 90
Byte No 2 : 144
Byte No 3 : 0
Byte No 4 : 3
Byte No 5 : 0
Byte No 6 : 0
Byte No 7 : 0
Byte No 8 : 4
Byte No 9 : 0
Byte No 10 : 0
使用類型轉換和 -Encoding
參數,可以直接將文件讀取為字節數組。我們可以使用 GetType()
方法來驗證結果確實是字節數組,並訪問其名為 Name
的屬性。
為了進一步驗證數據是否確實正確轉換,我們可以寫一個小的 for
循環,並打印出文件的一些字節。
在 PowerShell 7+ 中將數據轉換為字節數組
考慮以下代碼:
[byte[]]$data = Get-Content "a.exe" -AsByteStream
Write-Host $data.GetType().Name
這將產生以下輸出:
textByte[]
大多數語法在版本之間是相同的。唯一的區別是 -Encoding Byte
被替換為 -AsByteStream
。
在 PowerShell 中將字節數組轉換為字符串
將任何給定的字節數組轉換為字符串是容易的。考慮以下代碼:
$array = @(0x54, 0x68, 0x65, 0x20, 0x6f, 0x62, 0x6a, 0x65, 0x63, 0x74, 0x20, 0x6f, 0x66, 0x20, 0x6c, 0x69, 0x66, 0x65, 0x20, 0x69, 0x73, 0x20, 0x6e, 0x6f, 0x74, 0x20, 0x74, 0x6f, 0x20, 0x62, 0x65, 0x20, 0x6f, 0x6e, 0x20, 0x74, 0x68, 0x65, 0x20, 0x73, 0x69, 0x64, 0x65, 0x20, 0x6f, 0x66, 0x20, 0x74, 0x68, 0x65, 0x20, 0x6d, 0x61, 0x6a, 0x6f, 0x72, 0x69, 0x74, 0x79, 0x2c, 0x20, 0x62, 0x75, 0x74, 0x20, 0x74, 0x6f, 0x20, 0x65, 0x73, 0x63, 0x61, 0x70, 0x65, 0x20, 0x66, 0x69, 0x6e, 0x64, 0x69, 0x6e, 0x67, 0x20, 0x6f, 0x6e, 0x65, 0x73, 0x65, 0x6c, 0x66, 0x20, 0x69, 0x6e, 0x20, 0x74, 0x68, 0x65, 0x20, 0x72, 0x61, 0x6e, 0x6b, 0x73, 0x20, 0x6f, 0x66, 0x20, 0x74, 0x68, 0x65, 0x20, 0x69, 0x6e, 0x73, 0x61, 0x6e, 0x65, 0x2e)
$string = [System.Text.Encoding]::UTF8.GetString($array)
$string
這將產生以下輸出:
The object of life is not to be on the side of the majority but to escape finding oneself in the ranks of the insane.
使用 UTF8.GetString
方法,我們可以將任何 UTF8 編碼的字節數組轉換回其字符串表示形式。請注意文本的編碼,因為在其他編碼(例如 ASCII)上使用此方法可能會產生異常結果。
在 PowerShell 中將字符串轉換為字節數組
與字節數組可以轉換為其字符串表示形式的方式類似,字符串也可以轉換為其字節表示形式。
考慮以下代碼:
$string = "Never esteem anything as of advantage to you that will make you break your word or lose your self-respect."
$bytes = [System.Text.Encoding]::Unicode.GetBytes($string)
Write-Host "First 10 Bytes of String are: "
for ($i = 0; $i -lt 10; $i++) { Write-Host $bytes[$i] }
這將產生以下輸出:
First 10 Bytes of String are:
78
0
101
0
118
0
101
0
114
0
在這個特定場景中,可以使用 Unicode.GetBytes
獲取 Unicode 字符串的字節。確保字符串是 Unicode;否則,轉換可能會導致重要數據的丟失。
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn