在 PowerShell 中否定條件
Rohan Timalsina
2022年5月16日
PowerShell
PowerShell Condition
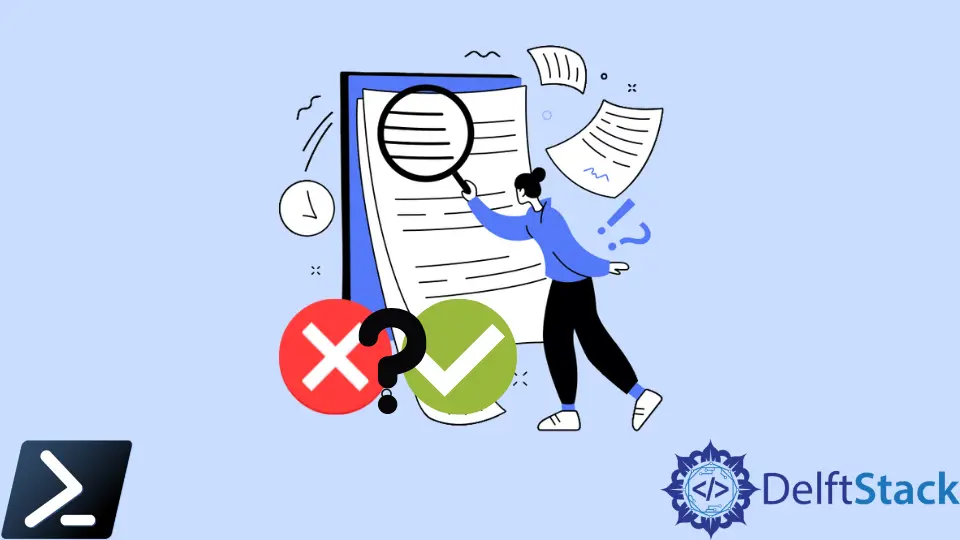
PowerShell 有不同的決策語句來執行程式碼,就像其他程式語言一樣。你可以在 PowerShell 指令碼中使用條件進行決策。指令碼根據這些決定執行不同的操作。如果條件為 true
,它將執行一個命令,如果條件為 false
,它將執行另一個命令。
PowerShell 中最常用的語句之一是 If
語句。它具有三種型別:if
語句、if-else
語句和巢狀 if
語句。PowerShell 還使用 switch
語句作為條件語句。
這是 if
語句的一個簡單示例。
if(5 -lt 7){
Write-Host "5 is less than 7"
}
如果 5 小於 7,則執行 Write-Host
命令。
輸出:
5 is less than 7
邏輯運算子連線 PowerShell 中的條件語句,允許你測試多個條件。PowerShell 支援 -and
、-or
、-xor
、-not
和 !
邏輯運算子。本教程將教你否定 PowerShell 中的條件。
使用 -not
運算子否定 PowerShell 中的條件
-not
是一個邏輯運算子,用於否定 PowerShell 中的語句。你可以使用 -not
運算子來否定 PowerShell 中的條件。
if (-not (5 -lt 7)){
Write-Host "5 is less than 7"
}
這一次,它不列印任何輸出,因為使用 -not
運算子時條件變為否定。它說 5 is less than 7
,這是錯誤的。
現在,讓我們用 -not
運算子測試另一個條件 5 大於 7
。
if (-not (5 -gt 7)){
Write-Host "5 is less than 7"
}
它列印輸出,因為 5 不大於 7
為真。
輸出:
5 is less than 7
在 PowerShell 中採用 !
運算子否定條件
你也可以使用!
運算子來否定 PowerShell 中的條件。它與 -not
運算子相同。
我們有兩個變數,$a
和 $b
,其值如下。
$a=3; $b=9
在這裡,條件變為 $a 不小於 $b
與 !
運算子。如果條件為 true
,則執行第一個命令,如果條件為 false
,則執行第二個命令。
if (! ($a -lt $b)){
Write-Host "$a is greater than $b"
}
else{
Write-Host "$a is less than $b"
}
輸出:
3 is less than 9
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Rohan Timalsina