在 Windows PowerShell 中獲取命令列引數
- 在 PowerShell 指令碼中定義引數
- PowerShell 指令碼中的命名引數
- 為 PowerShell 指令碼中的引數分配預設值
- PowerShell 指令碼中的切換引數
- PowerShell 指令碼中的強制引數
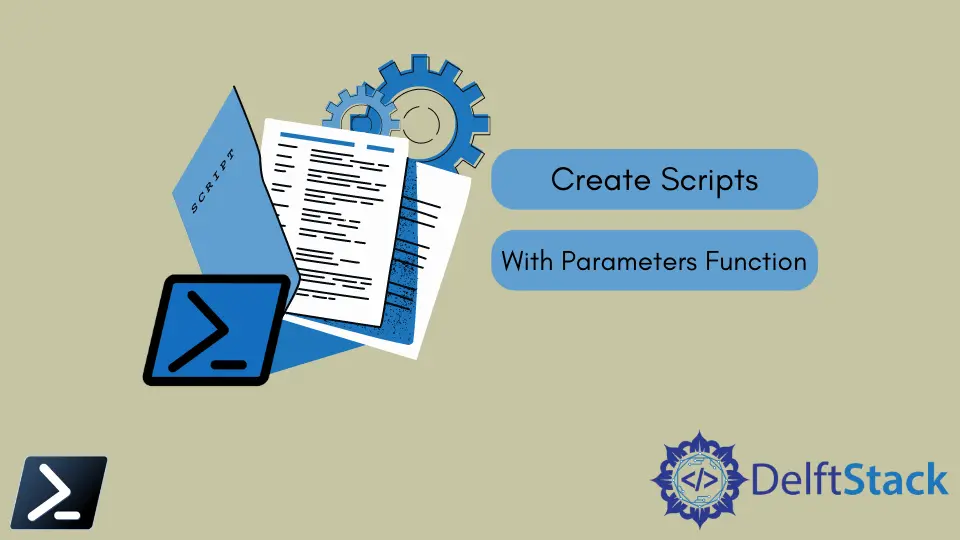
我們使用稱為 param()
的 Windows PowerShell 引數函式處理引數。Windows PowerShell 引數函式是任何指令碼的基本元件。一個引數是開發人員如何讓指令碼使用者在執行時提供輸入。
在本文中,你將學習如何使用引數函式建立指令碼、使用它們以及構建引數的一些最佳實踐。
在 PowerShell 指令碼中定義引數
管理員可以使用引數函式 param()
為指令碼和函式建立引數。一個函式包含一個或多個由變數定義的引數。
Hello_World.ps1
:
param(
$message
)
但是,為了確保引數只接受你需要的輸入型別,最佳實踐規定使用引數塊 [Parameter()]
為引數分配資料型別,並在變數前用方括號 []
括住資料型別。
Hello_World.ps1
:
param(
[Parameter()]
[String]$message
)
在上面的示例 Hello_World.ps1
中,變數 message
僅在給定值具有資料型別 String
時才接受傳遞的值。
PowerShell 指令碼中的命名引數
在指令碼中使用引數函式的一種方法是通過引數名稱——這種方法稱為命名引數。通過命名引數呼叫指令碼或函式時,我們使用變數名作為引數的全名。
在這個例子中,我們建立了一個 Hello_World.ps1
並在引數函式中定義了變數。請記住,我們可以在引數函式中放置一個或多個變數。
Hello_World.ps1
:
param(
[Parameter()]
[String]$message,
[String]$emotion
)
Write-Output $message
Write-Output "I am $emotion"
然後,我們可以在執行 .ps1
檔案時使用命名引數作為引數。
.\Hello_World.ps1 -message 'Hello World!' -emotion 'happy'
輸出:
Hello World!
I am happy
為 PowerShell 指令碼中的引數分配預設值
我們可以通過在指令碼中給引數一個值來為引數預分配一個值。然後,在不從執行行傳遞值的情況下執行指令碼將採用指令碼內定義的變數的預設值。
param(
[Parameter()]
[String]$message = "Hello World",
[String]$emotion = "happy"
)
Write-Output $message
Write-Output "I am $emotion"
.\Hello_World.ps1
輸出:
Hello World!
I am happy
PowerShell 指令碼中的切換引數
我們可以使用的另一個引數是 [switch]
資料型別定義的 switch 引數。switch 引數用於二進位制或布林值以指示 true
或 false
。
Hello_World.ps1
:
param(
[Parameter()]
[String]$message,
[String]$emotion,
[Switch]$display
)
if($display){
Write-Output $message
Write-Output "I am $emotion"
}else{
Write-Output "User denied confirmation."
}
我們可以使用下面的語法使用 script 引數呼叫我們的指令碼。
.\Hello_World.ps1 -message 'Hello World!' -emotion 'happy' -display:$false
輸出:
User denied confirmation.
PowerShell 指令碼中的強制引數
執行指令碼時必須使用一個或多個引數是很常見的。我們可以通過在引數塊 [Parameter()]
中新增 Mandatory
屬性來使引數成為強制性引數。
Hello_World.ps1:
param(
[Parameter(Mandatory)]
[String]$message,
[String]$emotion,
[Switch]$display
)
if($display){
Write-Output $message
Write-Output "I am $emotion"
}else{
Write-Output "User denied confirmation."
}
執行 Hello_World.ps1
時,Windows PowerShell 將不允許指令碼執行並提示你輸入值。
.\Hello_World.ps1
輸出:
cmdlet hello_world.ps1 at command pipeline position 1
Supply values for the following parameters:
message:
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn