使用 PowerShell 檢查字串的開頭
-
使用
-Like
邏輯運算子使用 PowerShell 檢查字串的開頭 -
使用
-cLike
邏輯運算子使用 PowerShell 檢查字串的開頭 -
在 PowerShell 中使用
StartsWith()
函式來檢查字串的開頭
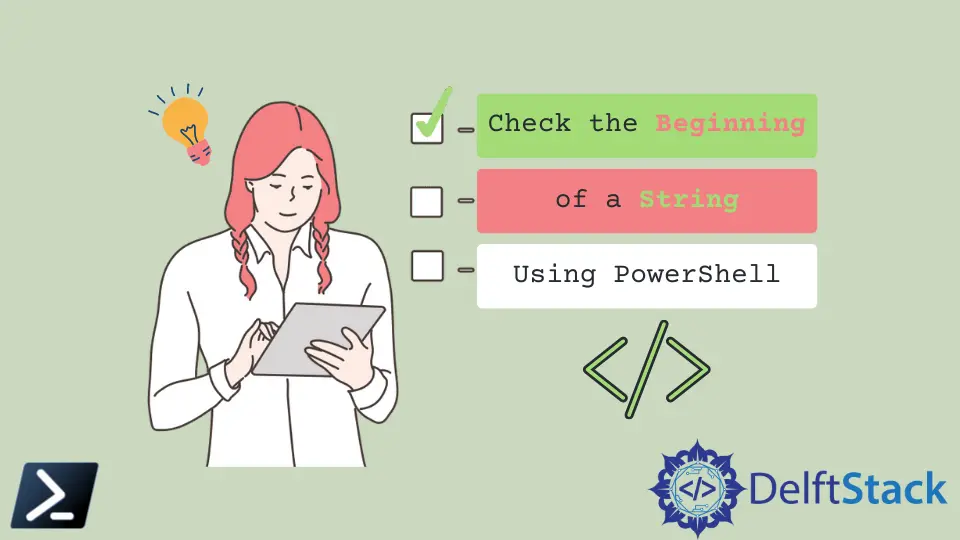
在某些情況下,我們可能會遇到需要檢查字串變數是否以字元或字串開頭的用例。
檢查字串是否以特定字元或字串開頭是編寫指令碼時的常見做法,並且在使用 Windows PowerShell 編寫時也相當容易。
本文將演示如何使用 Windows PowerShell 中的不同方法檢查字串變數的開頭。
我們可以使用帶有萬用字元的 Windows PowerShell -like
運算子來檢查字串的開頭是否區分大小寫和不區分大小寫。但在我們討論它的語法之前,我們可能需要澄清什麼是萬用字元。
萬用字元是用於匹配多個字元的系統模式。它們在 cmdlet 中指定特定模式以過濾結果或僅返回萬用字元結果。Windows PowerShell 中有多種型別的萬用字元可用。它們被表示為*
、?
、[m-n]
和 [abc]
。
在此特定用例中,我們將使用星號 (*
) 萬用字元。星號萬用字元表示匹配模式必須包含零個或多個字元。因此,例如,字串 ba*
可能對應於 bat
、bath
、bars
、basil
、basilica
或簡單的 ba。
使用 -Like
邏輯運算子使用 PowerShell 檢查字串的開頭
以下方法使用 -like
運算子檢查字串是否以另一個字串開頭。預設情況下,-Like
運算子忽略區分大小寫的語句。但是,如前所述,如果我們使用邏輯運算子,則必須與星號萬用字元配合使用。
示例程式碼:
$strVal = 'Hello World'
if($strVal -like 'hello*') {
Write-Host "Your string starts with hello."
} else {
Write-Host "Your string doesn't start with hello."
}
輸出:
Your string starts with hello.
使用 -cLike
邏輯運算子使用 PowerShell 檢查字串的開頭
我們可以使用 -cLike
運算子來執行區分大小寫的比較。
$strVal = 'Hello World!'
if($strVal -clike 'h*') {
Write-Host "Your string starts with lowercase h."
} else {
Write-Host "Your string starts with uppercase H."
}
輸出:
Your string starts with uppercase H.
在 PowerShell 中使用 StartsWith()
函式來檢查字串的開頭
我們還可以使用 .NET 框架的字串擴充套件函式 StartsWith()
來檢查字串是否以一組字元開頭。
以下方法檢查一個字串是否以另一個字串開頭。
$strVal ='Hello World!'
if($strVal.StartsWith('Hello')) {
Write-Host 'Your string starts with hello.'
} else {
Write-Host 'Your string doesn't start with hello.'
}
StartsWith
函式還接受另一個引數,我們可以使用它來檢查區分大小寫的字元。這個引數是 CurrentCultureIgnoreCase
。如果要執行區分大小寫的比較,請使用以下方法。
$strVal ='Hello world'
if($strVal.StartsWith('hello','CurrentCultureIgnoreCase')) {
Write-Host 'True'
} else {
Write-Host 'False'
}
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn