在 PHP 中使用 SoapClient 的示例
- 從 PHP 中的 Web 服務獲取函式和型別
- PHP SOAP 示例 1:溫度轉換
- PHP SOAP 示例 2:打個招呼
- PHP SOAP 示例 3:簡單算術
- PHP SOAP 示例 4:國家資訊
-
PHP SOAP 示例 5:使用
NuSOAP
進行溫度轉換 -
額外示例:使用
cURL
的 SOAP 呼叫
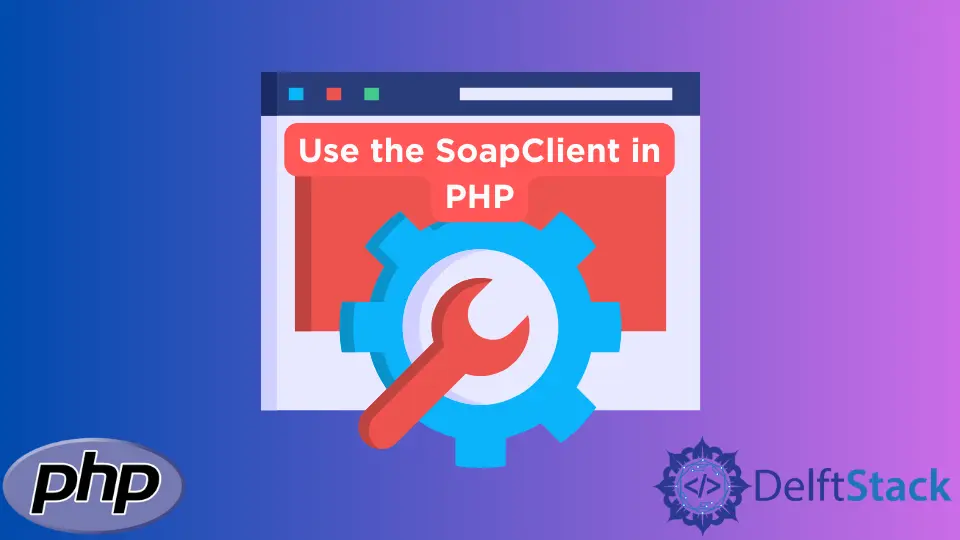
本文介紹了使用 PHP SoapClient
處理 WSDL 檔案的六個示例。在此之前,我們將解釋如何從 Web 服務中獲取函式和型別。
如果你想使用 Web 服務,這是必須的。
從 PHP 中的 Web 服務獲取函式和型別
在使用 Web 服務之前,你必須做的第一件事是瞭解它的功能和型別。那是因為服務函式將從服務返回資料。
型別是你可能提供給函式的引數的資料型別。我們說可能
是因為,稍後你會發現,並非所有服務函式都需要引數。
在 PHP 中,你可以使用以下內容從服務中獲取函式和型別。將服務 URL 替換為另一個,PHP 將返回函式的名稱和型別。
你應該連線到網際網路才能使程式碼正常工作。
<?php
$client = new SoapClient("http://www.dneonline.com/calculator.asmx?WSDL");
echo "<b>The functions are:</b> <br/>";
echo "<pre>";
var_dump($client->__getFunctions());
echo "</pre>";
echo "<b>The types are:</b> <br />";
echo "<pre>";
var_dump($client->__getTypes());
echo "</pre>";
?>
輸出(以下是摘錄):
<b>The functions are:</b> <br/><pre>array(8) {
[0]=>
string(32) "AddResponse Add(Add $parameters)"
[1]=>
string(47) "SubtractResponse Subtract(Subtract $parameters)"
[2]=>
string(47) "MultiplyResponse Multiply(Multiply $parameters)"
[3]=>
string(41) "DivideResponse Divide(Divide $parameters)"
[4]=>
string(32) "AddResponse Add(Add $parameters)"
[5]=>
string(47) "SubtractResponse Subtract(Subtract $parameters)"
[6]=>
string(47) "MultiplyResponse Multiply(Multiply $parameters)"
[7]=>
string(41) "DivideResponse Divide(Divide $parameters)"
}
</pre><b>The types are:</b> <br /><pre>array(8) {
[0]=>
string(36) "struct Add {
int intA;
int intB;
}"
[1]=>
string(38) "struct AddResponse {
int AddResult;
}"
[2]=>
string(41) "struct Subtract {
int intA;
int intB;
}"
..........
筆記:
- 在
types
下,你將找到為函式定義的引數的資料型別。 - 在程式碼中為函式提供引數時,資料型別必須相同。
- 如果你在型別定義中看到類似
int intA
的內容,則引數必須是名為intA
的整數。
現在你知道如何獲取服務功能和型別,你可以開始使用服務了。
PHP SOAP 示例 1:溫度轉換
W3schools 提供了一個溫度轉換器 WSDL 檔案。在 PHP 中,你可以連線到此檔案以執行溫度轉換。
為此(以及使用 SoapClient
的其他 SOAP 操作)採取的步驟如下:
-
建立一個新的
SoapClient
物件。 -
定義你要使用的資料。此資料應採用服務功能可以使用的格式。
-
呼叫服務的函式。
-
顯示結果。
我們在下面的程式碼中實現了這些步驟。
<?php
// Create the client object and pass in the
// URL of the SOAP server.
$soap_client = new SoapClient('https://www.w3schools.com/xml/tempconvert.asmx?WSDL');
// Create an associative array of the data
// that you'll pass into one of the functions
// of the client.
$degrees_in_celsius = array('Celsius' => '25');
// Use a function of the client for the
// conversion.
$convert_to_fahrenheit = $soap_client->CelsiusToFahrenheit($degrees_in_celsius);
// Show the result using var_dump. Other PHP
// functions like echo will not work.
echo "<pre>";
var_dump($convert_to_fahrenheit);
echo "</pre>";
// Repeat the same process. This time, use the
// FahrenheitToCelsius function to convert a
// temperature from Fahrenheit to Celsius.
$degrees_in_fahrenheit = array('Fahrenheit' => '25');
$convert_to_celsius = $soap_client->FahrenheitToCelsius($degrees_in_fahrenheit);
echo "<pre>";
var_dump($convert_to_celsius);
echo "</pre>";
?>
輸出:
<pre>object(stdClass)#2 (1) {
["CelsiusToFahrenheitResult"]=>
string(2) "77"
}
</pre><pre>object(stdClass)#3 (1) {
["FahrenheitToCelsiusResult"]=>
string(17) "-3.88888888888889"
}
</pre>
PHP SOAP 示例 2:打個招呼
我們的第二個示例是使用 learnwebservices.com
的打招呼
訊息。你所要做的就是使用 SoapClient
啟動對服務的 SOAP 呼叫。
之後,呼叫它的 SayHello
方法並傳入你想要的名稱。然後你可以訪問 Message
方法來顯示訊息。
訊息應該是 Hello your_desired_name!
。例如,如果 your_desired_name
是 Martinez
,你將獲得 Hello Martinez!
的輸出。
這就是我們在下一個程式碼中所做的。
<?php
$soap_client = new SoapClient('https://apps.learnwebservices.com/services/hello?wsdl');
// Call a function of the soap client.
$say_hello = $soap_client->SayHello(['Name' => 'Martinez']);
// Print the message from the client.
echo $say_hello->Message;
?>
輸出:
Hello Martinez!
PHP SOAP 示例 3:簡單算術
在這個例子中,我們使用一個允許你執行簡單算術的 Web 服務。這種算術包括加法、除法、乘法和減法。
但是,我們只會展示如何使用服務進行新增和分割。你應該嘗試其餘的,因為它會讓你更好地瞭解服務。
此服務的算術功能要求數字有名稱。這些名稱是 intA
和 intB
,因此我們將這些名稱分配給關聯陣列中的數字以方便使用。
之後,我們將此陣列傳遞給服務的 Add
和 Divide
函式。下面的程式碼顯示了所有這些在行動和計算結果。
<?php
// Connect to a SOAP client that allows you
// to do basic calculations.
$soap_client = new SoapClient("http://www.dneonline.com/calculator.asmx?WSDL");
// Define data that'll use with the client. This
// time the client requires that arguments that
// you'll pass in has the name intA and intB.
// Therefore, we use that name in our array.
$numbers_for_the_client = array("intA" => 222, "intB" => 110);
$add_the_numbers = $soap_client->Add($numbers_for_the_client);
$divide_the_numbers = $soap_client->Divide($numbers_for_the_client);
// Show the results of the addition and
// division of numbers.
echo "<pre>";
var_dump($add_the_numbers);
var_dump($divide_the_numbers);
echo "</pre>";
?>
輸出:
<pre>object(stdClass)#2 (1) {
["AddResult"]=>
int(332)
}
object(stdClass)#3 (1) {
["DivideResult"]=>
int(2)
}
</pre>
PHP SOAP 示例 4:國家資訊
如果你想獲取有關某個國家/地區的某些詳細資訊,此示例中的服務適合你。該服務有許多功能可以返回一個國家的詳細資訊。
其中一些函式是 ListOfCountryNameByCodes
和 ListOfContinentsByName
。下面,我們使用 ListOfCountryNameByCodes
列印世界上的國家。
<?php
// A soap client that allows you to view information
// about countries in the world
$soap_client = new SoapClient("http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso?WSDL");
// Use a client function to print country names.
$list_countries_names_by_code = $soap_client->ListOfCountryNamesByCode();
/*
Here is another function you can try out.
$list_of_continents_by_name = $client->ListOfContinentsByName();
*/
// Show the results.
echo "<pre>";
var_dump($list_countries_names_by_code);
echo "</pre>";
?>
輸出(以下是摘錄):
<pre>object(stdClass)#2 (1) {
["ListOfCountryNamesByCodeResult"]=>
object(stdClass)#3 (1) {
["tCountryCodeAndName"]=>
array(246) {
[0]=>
object(stdClass)#4 (2) {
["sISOCode"]=>
string(2) "AD"
["sName"]=>
string(7) "Andorra"
}
[1]=>
object(stdClass)#5 (2) {
["sISOCode"]=>
string(2) "AE"
["sName"]=>
string(20) "United Arab Emirates"
}
[2]=>
object(stdClass)#6 (2) {
["sISOCode"]=>
string(2) "AF"
["sName"]=>
string(11) "Afghanistan"
}
....
PHP SOAP 示例 5:使用 NuSOAP
進行溫度轉換
NuSOAP
是一個 PHP 工具,它允許你將 SOAP 連線到 Web 服務。操作模式類似於使用 SoapClient
,但有以下區別。
nusoap_client
需要服務地址和wsdl
作為其引數。- 你將處理的資料必須是 XML。
要使用 NuSOAP
,請從 SourceForge
下載它並將其放到你當前的工作目錄中。然後使用以下程式碼進行溫度轉換。
你將觀察到步驟是相同的,除了前面詳述的例外。還有一件事,程式碼在 PHP5
中工作,它在 PHP8
中返回 bool
。
<?php
// This PHP script use NUSOAP for SOAP connection
// and it works in PHP5 ONLY.
require_once('nusoap.php');
// Specify the WSDL file and initiate a new
// NUSOAP client.
$wsdl = "https://www.w3schools.com/xml/tempconvert.asmx?WSDL";
$nusoap_client = new nusoap_client($wsdl, 'wsdl');
// Call on the required method of the client.
$function_from_client = "CelsiusToFahrenheit";
// We'll save the result of the entire operation
// in an array.
$result = array();
// Temperature for conversion.
$temperature = 86;
// Specify the temperature as an XML file
$temperature_as_xml= '<CelsiusToFahrenheit xmlns="https://www.w3schools.com/xml/">
<Celsius>' . $temperature . '</Celsius>
</CelsiusToFahrenheit>';
// If the function exists in the client, initiate
// the conversion.
if (isset($function_from_client)) {
$result['response'] = $nusoap_client->call($function_from_client, $temperature_as_xml);
}
// Show the results
echo "<pre>" . $temperature . ' Celsius => ' . $result['response']['CelsiusToFahrenheitResult'] . ' Fahrenheit' . "</pre>";
?>
輸出:
<pre>86 Celsius => 186.8 Fahrenheit</pre>
額外示例:使用 cURL
的 SOAP 呼叫
你可以使用 cURL
對 Web 服務進行 SOAP 呼叫。我們將再次使用 W3Schools 溫度轉換服務。
同時,使用 cURL
,你必須在使用服務時執行以下操作。
- 資料應該在
Soap Envelope
中。 - 你必須將內容標頭髮送到瀏覽器。
- 你必須定義
cURL
連線選項。
我們在下面的程式碼中完成了所有這些以及更多工作;程式碼註釋詳細說明了每一步發生了什麼。
<?php
// This script allows you to use w3schools
// convert via curl.
$webservice_address = "https://www.w3schools.com/xml/tempconvert.asmx";
// The number you'll like to convert, in this case
// we'll like to convert a temperature in celsius
// fahrenheit.
$temperature = 56;
// Pass the temperature as an XML file.
// The XML format is based on the official
// format for the SOAP client by w3schools.
// You can find more at:
$temperature_as_xml = '<?xml version="1.0" encoding="utf-8"?>
<soap12:Envelope
xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="https://www.w3.org/2001/XMLSchema"
xmlns:soap12="https://www.w3.org/2003/05/soap-envelope">
<soap12:Body>
<CelsiusToFahrenheit xmlns="https://www.w3schools.com/xml/">
<Celsius>' . $temperature . '</Celsius>
</CelsiusToFahrenheit>
</soap12:Body>
</soap12:Envelope>';
// Define content headers
$content_headers = array(
'Content-Type: text/xml; charset=utf-8',
'Content-Length: '.strlen($temperature_as_xml)
);
// Initiate the CURL connection and define
// the connection options.
$init_curl_connection = curl_init($webservice_address);
curl_setopt($init_curl_connection, CURLOPT_POST, true);
curl_setopt($init_curl_connection, CURLOPT_HTTPHEADER, $content_headers);
curl_setopt($init_curl_connection, CURLOPT_POSTFIELDS, $temperature_as_xml);
curl_setopt($init_curl_connection, CURLOPT_RETURNTRANSFER, true);
curl_setopt($init_curl_connection, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($init_curl_connection, CURLOPT_SSL_VERIFYPEER, false);
// Use the curl_exec function to get the
// received data.
$recieved_data = curl_exec($init_curl_connection);
// Save the connection result in another variable.
// This will allow us to use it in an "if" statement
// without causing ambiguity.
$connection_result = $recieved_data;
// Check if there is an error.
if ($connection_result === FALSE) {
printf("CURL error (#%d): %s<br>\n", curl_errno($init_curl_connection),
htmlspecialchars(curl_error($init_curl_connection)));
}
// Close the CURL connection
curl_close ($init_curl_connection);
// Show the received data.
echo $temperature . ' Celsius => ' . $recieved_data . ' Fahrenheit';
?>
輸出(在瀏覽器中):
56 Celsius => 132.8 Fahrenheit
輸出(詳細版本):
Celsius => <?xml version="1.0" encoding="utf-8"?>
<soap:Envelope xmlns:soap="https://www.w3.org/2003/05/soap-envelope" xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="https://www.w3.org/2001/XMLSchema">
<soap:Body>
<CelsiusToFahrenheitResponse xmlns="https://www.w3schools.com/xml/">
<CelsiusToFahrenheitResult>132.8</CelsiusToFahrenheitResult>
</CelsiusToFahrenheitResponse><
/soap:Body></soap:Envelope>Fahrenheit
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn