如何在 PHP 中解析 JSON 檔案
Minahil Noor
2021年4月29日
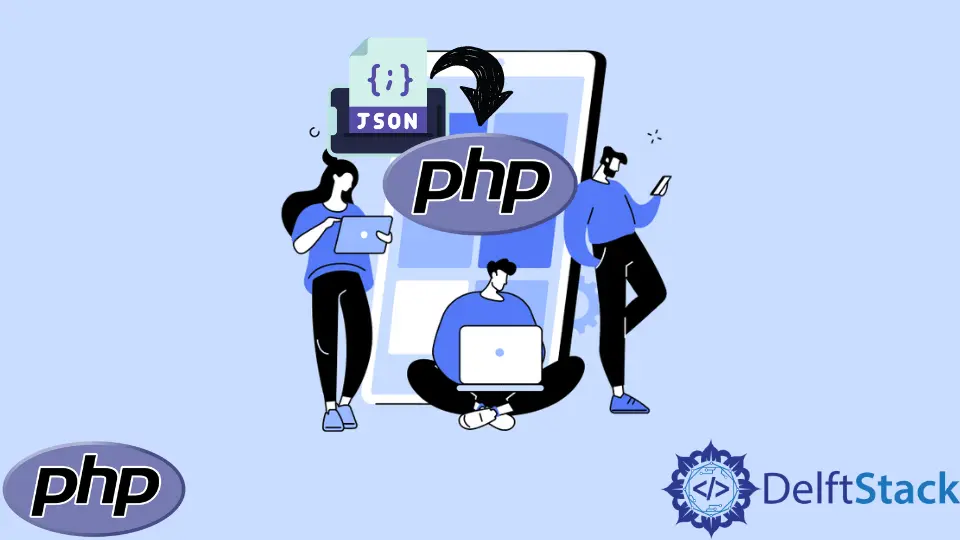
在本文中,我們將介紹在 PHP 中解析 JSON
檔案的方法。
- 使用
file_get_contents()
函式
示例程式碼中使用的 JSON
檔案的內容如下。
[
{
"id": "01",
"name": "Olivia Mason",
"designation": "System Architect"
},
{
"id": "02",
"name": "Jennifer Laurence",
"designation": "Senior Programmer"
},
{
"id": "03",
"name": "Medona Oliver",
"designation": "Office Manager"
}
]
使用 file_get_contents()
函式解析 PHP 中的 JSON 檔案
內建函式 file_get_contents()
用於讀取檔案並將其儲存為字串。通過使用此函式,我們可以將 JSON
檔案解析為字串。使用此函式的正確語法如下。
file_get_contents($pathOfFile, $searchPath, $customContext, $startingPoint, $length);
此函式接受五個引數。這些引數的詳細資訊如下。
引數 | 描述 | |
---|---|---|
$pathOfFile |
強制性的 | 它指定檔案的路徑 |
$searchPath |
可選的 | 它指定搜尋檔案的路徑 |
$customContext |
可選的 | 它用於指定自定義上下文 |
$startingPoint |
可選的 | 它指定讀取檔案的起點 |
$length |
可選的 | 它是要讀取的檔案的最大長度(以位元組為單位) |
以下程式顯示瞭如何解析 JSON
檔案。
<?php
$JsonParser = file_get_contents("myfile.json");
var_dump($JsonParser);
?>
函式 file_get_contents()
僅解析儲存在 JSON 檔案中的 JSON 資料。我們無法直接使用此資料。
輸出:
string(328) "[
{
"id": "01",
"name": "Olivia Mason",
"designation": "System Architect"
},
{
"id": "02",
"name": "Jennifer Laurence",
"designation": "Senior Programmer"
},
{
"id": "03",
"name": "Medona Oliver",
"designation": "Office Manager"
}
]"
為了使這些資料有用,我們可以使用 json_decode()
將 JSON 字串轉換為陣列。在以下程式中使用此函式。
<?php
$Json = file_get_contents("myfile.json");
// Converts to an array
$myarray = json_decode($Json, true);
var_dump($myarray); // prints array
?>
輸出:
array(3) {
[0]=>
array(3) {
["id"]=>
string(2) "01"
["name"]=>
string(12) "Olivia Mason"
["designation"]=>
string(16) "System Architect"
}
[1]=>
array(3) {
["id"]=>
string(2) "02"
["name"]=>
string(17) "Jennifer Laurence"
["designation"]=>
string(17) "Senior Programmer"
}
[2]=>
array(3) {
["id"]=>
string(2) "03"
["name"]=>
string(13) "Medona Oliver"
["designation"]=>
string(14) "Office Manager"
}
}