如何在 PHP 中獲取字串的最後一個字元
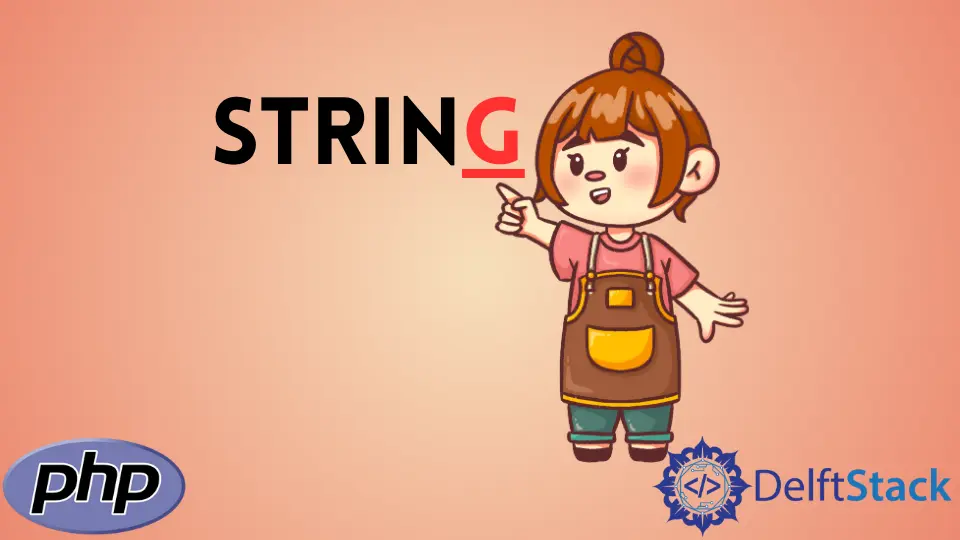
在本文中,我們將介紹獲取字串的最後一個字元的方法。
- 使用
substr()
函式 - 通過直接字串訪問
- 使用
for
迴圈
使用 substr()
函式獲取 PHP 中字串的最後一個字元
PHP 中內建的 substr()
函式可以獲取字串的子字串。此函式具有三個引數,其中一個是可選引數。使用此函式的正確語法如下
substr($stringName, $startingPosition, $lengthOfSubstring);
$stringName
是輸入字串。$startngPosition
是子字串的起始索引。$lengthOfSubstring
是子字串的長度。變數 $stringName
和 $startingPosition
是必需引數,而 $lengthOfSubstring
是可選引數。當省略 $lengthOfSubstring
時,子字串將從 $startngPosition
開始,直到輸入字串的末尾。
<?php
$string = 'This is a string';
$lastChar = substr($string, -1);
echo "The last char of the string is $lastChar.";
?>
如果 $startingPosition
具有正值,則子字串從字串的左側開始,即字串的開頭。如果 $startingPosition
是負數的話,則子字串從字串的右側開始,即字串的結尾。
為了獲得字串的最後一個字元,這裡我們將 -1
作為 $startingPosition
的值。-1
表示字串的最後一個字元。
輸出:
The last char of the string is g.
使用直接字串訪問獲取 PHP 中字串的最後一個字元
字串是一個字元陣列。我們可以通過考慮字串的最後一個字元來獲取字串的最後一個字元。
<?php
$string = "This is a string";
$lengthOfString = strlen($string);
$lastCharPosition = $lengthOfString-1;
$lastChar = $string[$lastCharPosition];
echo "The last char of the string is $lastChar.";
?>
字串的最後一個字元的索引比字串的長度小 1,因為索引從 0 開始。$lengthOfString
使用 strlen()
函式。$lastCharPosition
存放著字串的最後一個字元的索引。輸出是通過傳遞 $lastCharPosition
作為字元陣列的索引之後的最後一個字元 char
。
輸出:
The last char of the string is g.
從 PHP 7.1.0 進行負字串索引訪問
從 PHP 7.1.0 開始,字串的負索引也開始支援。因此,我們可以使用-1 作為索引來獲取 PHP 中字串的最後一個字元。
示例程式碼:
<?php
$string = "This is a string";
$lastChar = $string[-1];
echo "The last char of the string is $lastChar.";
?>
輸出:
The last char of the string is g.
使用 for
迴圈獲取 PHP 中字串的最後一個字元
我們可以通過將字串的最後一個 char 儲存到新的字串中,然後使用 for
迴圈獲取最後一個 char。我們將遍歷字串併到達最後一個字元。
<?php
$string = "This is a string";
$lastCharPosition = strlen($string) - 1;
for ($x = $lastCharPosition; $x < strlen($string); $x++) {
$newString = $string[$x];
}
echo "The last char of the string is $newString.";
?>
我們將使用 strlen()
函式獲得最後一個字元的索引,並在 for
迴圈中將其分配給 $x
。
輸出:
The last char of the string is g.