如何在 PHP 中從字串中提取數字
Minahil Noor
2023年1月30日
-
在 PHP 中使用
preg_match_all()
函式從一個字串中提取數字 -
在 PHP 中使用
filter_var()
函式從字串中提取數字 -
在 PHP 中使用
preg_replace()
函式從字串中提取數字
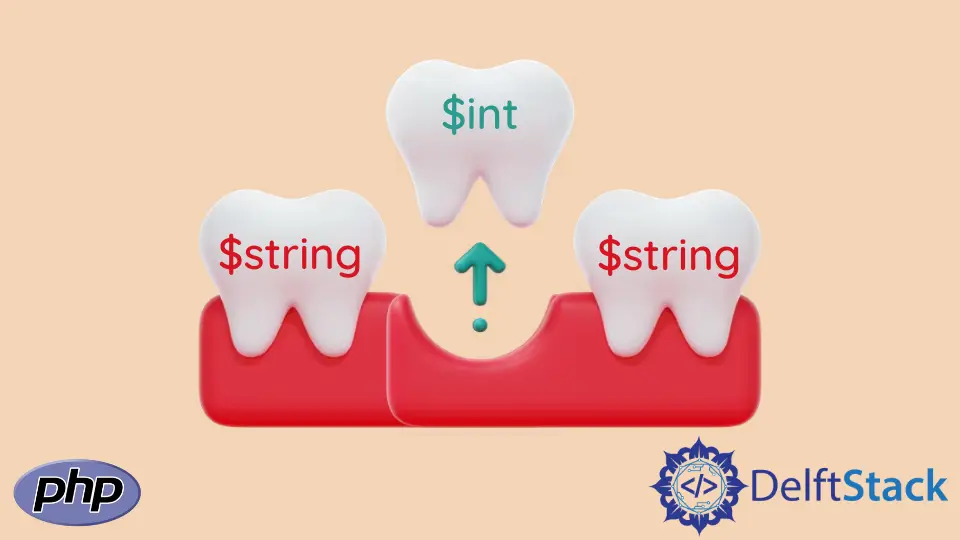
在本文中,我們將介紹在 PHP 中從字串中提取數字的方法。
- 使用
preg_match_all()
函式的方法 - 使用
filter_variable()
函式 - 使用
preg_replace()
函式
在 PHP 中使用 preg_match_all()
函式從一個字串中提取數字
我們可以使用內建函式 preg_match_all()
從字串中提取數字。這個函式從一個字串中全域性搜尋指定的模式。使用該函式的正確語法如下。
preg_match_all($pattern, $inputString, $matches, $flag, $offset);
內建函式 preg_match_all()
有五個引數。其詳細引數如下
引數 | 說明 | |
---|---|---|
$pattern |
強制 | 它是我們要在給定的 string 中檢查的模式 |
$inputString |
強制 | 它是我們要搜尋給定模式的字串 |
$matches |
可選 | 如果給定了這個引數,那麼函式將匹配過程的結果儲存在其中。 |
$flags |
可選 | 該引數有兩個選項。PREG_OFFSET_CAPTURE 和 PREG_UNMATCHED_AS_NULL 。你可以在這裡閱讀它的描述。 |
$offset |
可選 | 它告訴函式從哪裡開始匹配過程。通常情況下,搜尋從頭開始。 |
這個函式返回一個 Boolean
變數。如果給定的模式存在,它就返回 true。
下面的程式顯示了我們如何使用 preg_match_all()
函式從給定的 string
中提取數字。
<?php
$string = 'Sarah has 4 dolls and 6 bunnies.';
preg_match_all('!\d+!', $string, $matches);
print_r($matches);
?>
我們使用 !\d+!
模式從字串中提取數字。
輸出:
Array
(
[0] => Array
(
[0] => 4
[1] => 6
)
)
在 PHP 中使用 filter_var()
函式從字串中提取數字
我們還可以使用 filter_var()
函式從字串
中提取數字。使用這個函式的正確語法如下。
filter_var($variableName, $filterName, $options)
函式 filter_var()
接受三個引數。其詳細引數如下
引數 | 說明 | |
---|---|---|
$variableName |
強制 | 它是要過濾的變數 |
$filterName |
強制 | 它是應用於變數的過濾器的名稱,預設情況下是 FILTER_DEFAULT 。預設情況下,它是 FILTER_DEFAULT 。 |
$options |
強制 | 這個引數告訴了我們要使用的選項,我們使用了 FILTER_SANITIZE_NUMBER_INT 過濾器。 |
我們使用了 FILTER_SANITIZE_NUMBER_INT
過濾器。從字串中提取數字的程式如下。
<?php
$string = 'Sarah has 4 dolls and 6 bunnies.';
$int = (int) filter_var($string, FILTER_SANITIZE_NUMBER_INT);
echo("The extracted numbers are: $int \n");
?>
輸出:
The extracted numbers are: 46
在 PHP 中使用 preg_replace()
函式從字串中提取數字
在 PHP 中,我們也可以使用 preg_replace()
函式從字串中提取數字。使用這個函式的正確語法如下。
preg_replace($regexPattern, $replacementVar, $original, $limit, $count)
函式 preg_replace()
接受五個引數。其詳細引數如下
引數 | 說明 | |
---|---|---|
$regexPattern |
強制 | 它是我們將在原始的字串或陣列中搜尋的模式 |
$replacementVar |
強制 | 它是字串或數,我們用它來替代搜尋值。 |
$original |
強制 | 它是一個字串或陣列,我們要從其中找到值並替換它。 |
$limit |
可選 | 這個引數限制了替換的數量 |
$count |
可選 | 這個引數告訴我們對原始字串或陣列的總替換次數 |
我們將使用模式 /[^0-9]/
來查詢字串中的數字。從字串中提取數字的程式如下。
<?php
$string = 'Sarah has 4 dolls and 6 bunnies.';
$outputString = preg_replace('/[^0-9]/', '', $string);
echo("The extracted numbers are: $outputString \n");
?>
輸出:
The extracted numbers are: 46