如何在 PHP 中將字串轉換為數字
Minahil Noor
2023年1月30日
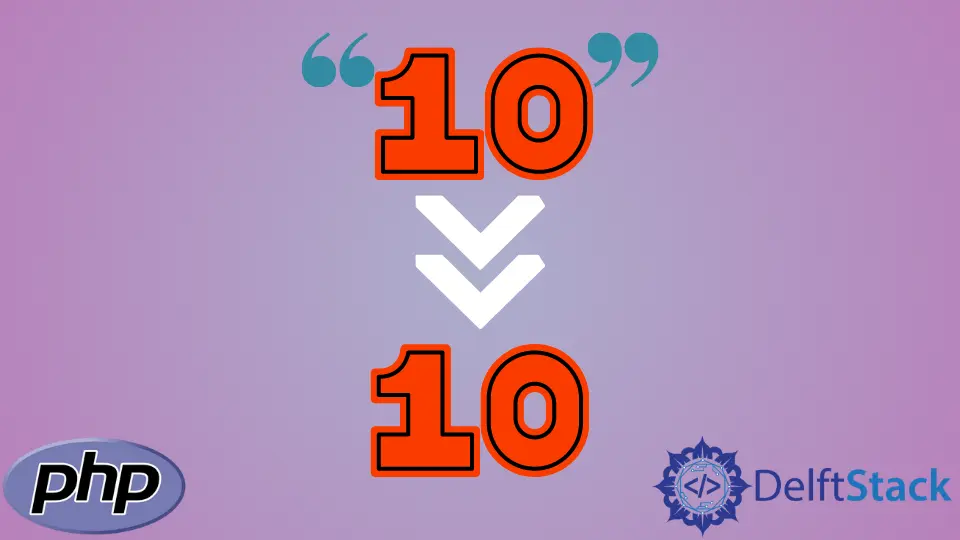
在本文中,我們將介紹在 PHP 中將字串轉換為數字的方法。
- 使用顯式型別轉換
- 使用
intval()
/floatval()
函式 - 使用
settype()
函式
在 PHP 中使用顯式型別轉換將字串轉換為數字
顯式型別轉換將任何型別的變數轉換為所需型別的變數。它將變數轉換為原始資料型別。將變數顯式型別轉換為 int
或 float
的正確語法如下
$variableName = (int)$stringName
$variableName = (float)$stringName
變數 $variableName
是整數變數,用於儲存 number
的值。變數 $stringName
是數字字串。我們還可以將非數字字串轉換為數字。
<?php
$variable = "10";
$integer = (int)$variable;
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "8.1";
$float = (float)$variable;
echo "The variable $variable has converted to a number and its value is $float.";
?>
輸出:
The variable 10 has converted to a number and its value is 10.
The variable 8.1 has converted to a number and its value is 8.1.
對於非數字字串,我們將得到 0 作為輸出。
<?php
$variable = "abc";
$integer = (int)$variable;
echo "The variable has converted to a number and its value is $integer.";
?>
輸出:
The variable has converted to a number and its value is 0.
在 PHP 中使用 intval()
函式將字串轉換為數字
intval()
函式將字串轉換為整型 int
。
floatval()
函式將字串轉換為浮點數 float
。
這些函式接受的引數是一個字串,返回值分別是一個整數或一個浮點數。
intval( $StringName );
變數 StringName
是字串型別。
<?php
$variable = "53";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
輸出:
The variable 53 has converted to a number and its value is 53.
The variable 25.3 has converted to a number and its value is 25.3.
如果給定的字串是數字和字串表示形式的混合,例如 2020Time
,則 intval()
將返回 2020
。但是,如果給定的字串不是以數字開頭,則它將返回 0
。floatval()
方法也是如此。
示例程式碼:
<?php
$variable = "2020Time";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "Time2020";
$integer = intval($variable);
echo "The variable $variable has converted to a number and its value is $integer.";
echo "\n";
$variable = "25.3Time";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
echo "\n";
$variable = "Time25.3";
$float = floatval($variable);
echo "The variable $variable has converted to a number and its value is $float.";
?>
輸出:
The variable 2020Time has converted to a number and its value is 2020.
The variable Time2020 has converted to a number and its value is 0.
The variable 25.3Time has converted to a number and its value is 25.3.
The variable Time25.3 has converted to a number and its value is 0.
在 PHP 中使用 settype()
函式將字串轉換為數字
settype()
函式將任何資料型別的變數轉換為特定資料型別。它接受兩個引數,變數和型別名稱。
settype($variableName, "typeName");
$variableName
是我們要轉換為數字的任何資料型別的變數。"typeName"
是我們要為 $variableName
變數使用的資料型別的名稱。
<?php
$variable = "45";
settype($variable, "integer");
echo "The variable has converted to a number and its value is $variable.";
?>
settype()
函式將 $variable
的型別從字串設定為整型 integer
。它僅適用於原始資料型別。
輸出:
The variable has converted to a number and its value is 45.
相關文章 - PHP String
- 如何在 PHP 中刪除字串中的所有空格
- 如何在 PHP 中將 DateTime 轉換為字串
- 如何在 PHP 中將字串轉換為日期和日期時間
- 如何在 PHP 中將整數轉換為字串
- 如何在 PHP 中將陣列轉換為字串