PHP 中如何檢查字串是否以指定字串開頭
Minahil Noor
2023年1月30日
PHP
PHP String
-
使用
substr()
函式檢查字串是否以 PHP 中的指定字串開頭 -
使用
strpos()
函式檢查字串是否以 PHP 中的指定字串開頭 -
使用
strncmp()
函式檢查字串是否以 PHP 中的指定字串開頭
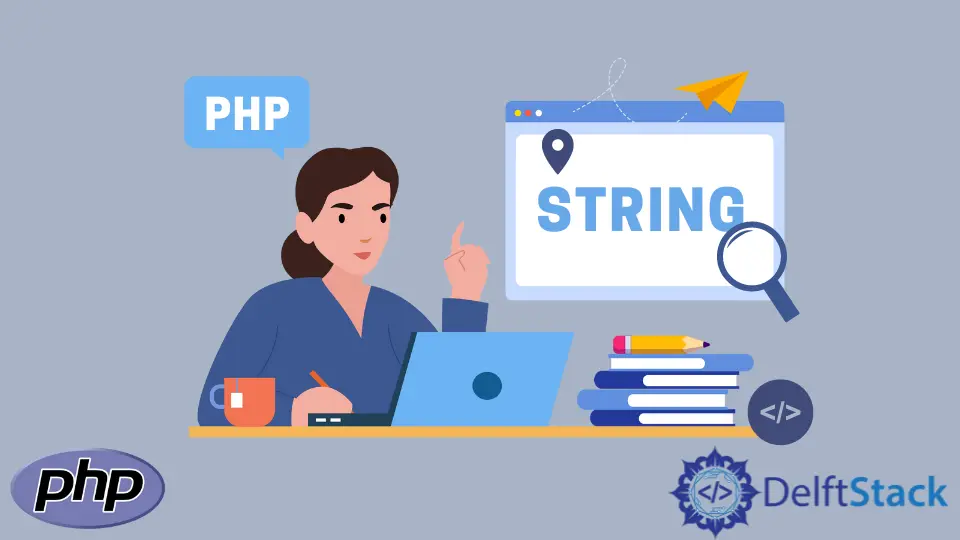
在本文中,我們將介紹檢查 PHP 中字串是否以指定的字串開頭的方法。
- 使用
substr()
函式 - 使用
strpos()
函式 - 使用
strncmp()
函式
使用 substr()
函式檢查字串是否以 PHP 中的指定字串開頭
內建函式 substr()
用於訪問子字串。傳遞字串作為輸入,並返回我們要訪問的子字串。我們可以使用這個函式來檢查 string
是否以特定的字串開頭。使用此函式的正確語法如下
substr($string, $startPosition, $lengthOfSubstring);
該功能具有三個引數。其引數的詳細資訊如下。
引數 | 描述 | |
---|---|---|
$string |
強制的 | 我們希望訪問其子字串的原始字串 |
$startPosition |
強制的 | 整數變數。它告訴我們子字串開始的位置。如果為正,則我們的子字串從字串的左側開始,即從開頭開始。如果為負,則我們的子字串從末尾開始。 |
$lengthOfSubstring |
可選的 | 整數變數。它從開始位置告訴字串的總長度。如果省略,則返回從字串的開始位置到結尾的子字串。如果為負,則根據其值從末尾刪除該字串。如果為零,則返回一個空字串。 |
<?php
$string = "Mr. Peter";
if(substr($string, 0, 3) === "Mr."){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
在上面的程式碼中,我們要檢查我們的字串是否以 Mr.
開頭。
substr($string, 0, 3)
0
是子字串的起始索引,換句話說,子字串從給定字串的第一個字元開始。
3 表示返回的子字串的長度為 3。
如果字串的開頭與 Mr.
相同,則它將顯示字串以所需的子字串開頭。
輸出:
The string starts with the desired substring.
使用 strpos()
函式檢查字串是否以 PHP 中的指定字串開頭
函式 strpos()
返回給定字串中子字串首次出現的位置。我們可以用它來檢查字串是否以指定的字串開頭。
如果返回值為 0
,則意味著給定的字串以指定的子字串開頭。否則,字串不能以檢查的子字串開頭。
strpos()
是區分大小寫的函式。使用此函式的正確語法如下。
strpos($string, $searchString, $startPosition);
它具有三個引數。其引數的詳細資訊如下。
引數 | 描述 | |
---|---|---|
$string |
強制的 | 這是我們希望找到其子字串的字串 |
$searchString |
強制的 | 它是將在字串中搜尋的子字串 |
$startPosition |
可選的 | 它是字串從搜尋開始的位置 |
<?php
$string = "Mr. Peter";
if(strpos( $string, "Mr." ) === 0){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
在這裡,我們通過查詢第一次出現的先生來檢查字串是否以 Mr.
開頭。
輸出:
The string starts with the desired substring.
使用 strncmp()
函式檢查字串是否以 PHP 中的指定字串開頭
內建函式 strncmp()
比較兩個給定的字串。此函式也區分大小寫。使用此函式的正確語法如下。
strncmp($string1, $string2, $length);
它具有三個引數。其引數的詳細資訊如下。
引數 | 描述 | |
---|---|---|
$string1 |
強制的 | 這是要比較的第一個字串 |
$string2 |
強制的 | 這是要比較的第二個字串 |
$length |
強制的 | 它是要比較的字串的長度 |
如果兩個字串相等,則返回零。這是區分大小寫的功能。
<?php
$string = "Mr. Peter";
if(strncmp($string, "Mr.", 3) === 0){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
在這裡,比較兩個字串。要比較的字串的長度為 3
。
輸出:
The string starts with the desired substring.
strncmp 的不區分大小寫的版本是 strncasecmp
。它比較給定兩個字串的前 n 個字元,而不管它們的大小寫如何。
<?php
$string = "mr. Peter";
if(strncasecmp($string, "Mr.", 3) === 0){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
輸出:
The string starts with the desired substring.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe