如何將圖另存為影象檔案而不在 Matplotlib 中顯示
Suraj Joshi
2023年1月30日
Matplotlib
Matplotlib Save Plots
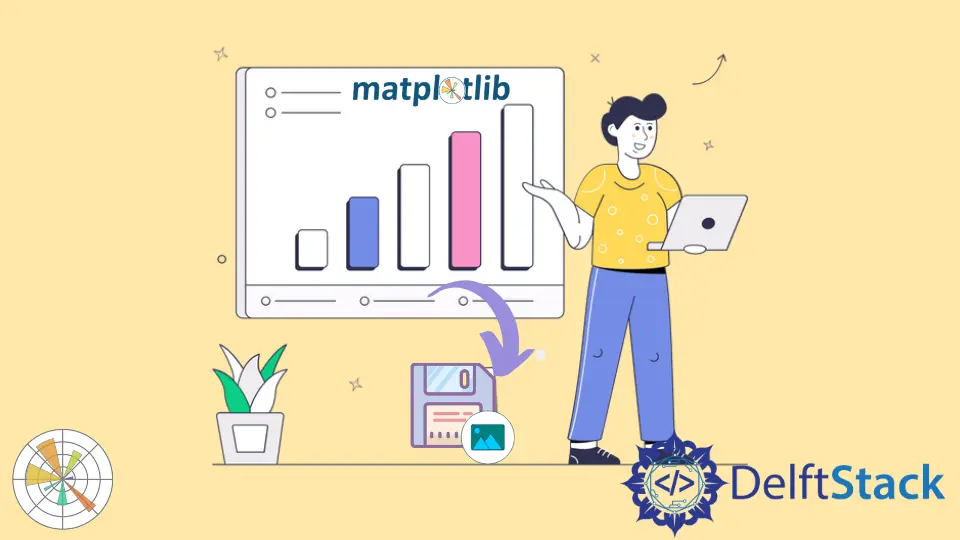
我們可以簡單地使用 savefig()
和 imsave()
方法儲存從 Matplotlib 生成的圖。如果我們處於互動模式,則可能會顯示該圖。為了避免顯示繪圖,我們使用 close()
和 ioff()
方法。
Matplotlib 中使用 savefig()
方法儲存影象
我們可以使用 matplotlib.pyplot.savefig()
儲存從 Matplotlib 生成的圖。我們可以在 savefig()
中指定需要儲存繪圖的路徑和格式。
語法:
matplotlib.pyplot.savefig(
fname,
dpi=None,
facecolor="w",
edgecolor="w",
orientation="portrait",
papertype=None,
format=None,
transparent=False,
bbox_inches=None,
pad_inches=0.1,
frameon=None,
metadata=None,
)
在這裡,fname
代表相對於當前目錄的路徑的檔名。
Matplotlib 中儲存圖形而不以互動模式顯示
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.savefig("Plot generated using Matplotlib.png")
這會將生成的圖以 Plot generated using Matplotlib.png
的名稱儲存在當前工作目錄中。
我們還可以將圖儲存為其他格式,例如 png,jpg,svg,pdf 等。同樣,我們可以使用 figsave()
方法的不同引數來定製影象。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.savefig("Customed Plot.pdf", dpi=300, bbox_inches="tight")
這會將圖另存為工作目錄中的 Customed Plot.pdf
。在這裡,dpi=300
表示儲存的影象中每英寸 300 個點,而 bbox_inches='tight'
表示輸出影象周圍沒有邊框。
Matplotlib 中儲存圖形而不以非互動模式顯示
但是,如果我們處於互動模式,則始終顯示該圖。為了避免這種情況,我們使用 close()
和 ioff()
方法強行關閉圖形視窗以防止圖形顯示。由於使用了 ion()
方法,因此設定了互動模式。
避免使用 close()
方法顯示
matplotlib.pyplot.close
可用於關閉圖形視窗。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
fig = plt.figure()
plt.ion()
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.close(fig)
plt.savefig("Plot generated using Matplotlib.png")
避免使用 ioff()
方法顯示
我們可以使用 matplotlib.pyplot.ioff()
方法關閉互動模式。這樣可以防止顯示圖形。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
fig = plt.figure()
plt.ion()
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.ioff()
plt.savefig("Plot generated using Matplotlib.png")
使用 matplotlib.pyplot.imsave()
方法儲存影象
我們可以使用 matplotlib.pyplot.imsave()
將陣列另存為 Matplotlib 中的影象檔案。
import numpy as np
import matplotlib.pyplot as plt
image = np.random.randn(100, 100)
plt.imsave("new_1.png", image)
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn