JavaScript 中的萬用字元字串比較
- JavaScript 中的正規表示式
-
使用
test()
方法作為 JavaScript 中的RegExp
物件 -
在 JavaScript 中使用
substring()
方法 -
在 JavaScript 中使用
substr()
函式 -
JavaScript 中
substring()
和substr()
的區別 - JavaScript 中的萬用字元字串比較
-
在 JavaScript 中使用
escapeRegex
函式 - まとめ
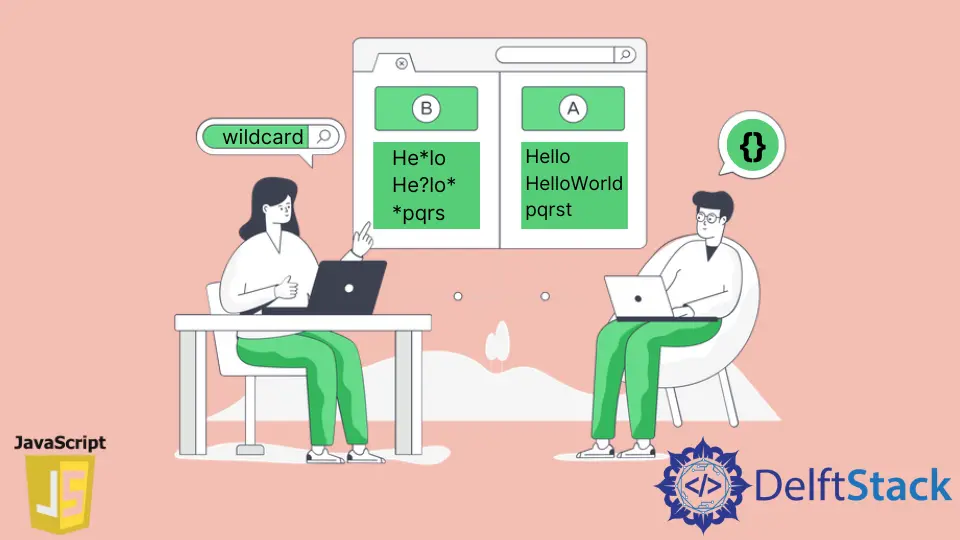
這篇文章是關於 JavaScript 的正規表示式,不同的字串方法,以及 JavaScript 中的萬用字元字串比較。這些問題被解決為:
- 什麼是 JavaScript 中的正規表示式。
substr
和substring
如何與正規表示式一起使用。- JavaScript 中如何使用萬用字元方法進行字串比較。
JavaScript 中的正規表示式
正規表示式是由一系列字元組成的搜尋模式。此搜尋模式可以定義你在搜尋文字中的資料時要查詢的內容。
正規表示式可能像單個字元一樣簡單,也可能像複雜模式一樣複雜。所有文字搜尋和文字替換操作都可以使用正規表示式來執行。
/BlocTAK/x;
在程式碼行中,BlocTAK
是用於搜尋的模式,x
是確保搜尋不區分大小寫的修飾符。
使用 test()
方法作為 JavaScript 中的 RegExp
物件
RegExp 表示式方法是 test()
方法。它在字串中查詢模式並根據結果返回 true 或 false。
程式碼 - HTML:
<html>
<body>
<p>Search for "H" in the next paragraph:</p>
<p id="5">Hello World</p>
<p id="data"></p>
</body>
</html>
它也可以使用 <script>
標籤新增到 HTML 的標題中。
程式碼 - JavaScript:
let text = document.getElementById('5').innerHTML;
const pattern = /e/;
document.getElementById('data').innerHTML = pattern.test(text);
在 JavaScript 中使用 substring()
方法
函式 substring()
從字串中提取字元並返回兩個索引(位置)之間的子字串。substring()
函式從字串的開頭到結尾刪除字元(不包括在內)。
substring()
方法不會改變原始字串。如果開始大於結束,則切換引數,並且當開始或結束值小於零時,它們被認為是 0
。
程式碼 - HTML:
<html>
<body>
<p>substring() extracts a part of a string:</p>
<p id="demo"></p>
</body>
</html>
程式碼 - JavaScript:
let text = 'Hello world!';
let result = text.substring(7, 5);
document.getElementById('demo').innerHTML = result;
在 JavaScript 中使用 substr()
函式
substr()
函式接受一個字串段並提取它。substr()
函式接受給定數量的字元並返回定義數量的字元。
substr()
不會更改原始字串。使用負開始位置從字串的末尾刪除字元。
程式碼 - HTML:
<html>
<body>
<p>substr() extracts a part of a string:</p>
<p id="data"></p>
</body>
</html>
程式碼 - JavaScript:
let text = 'Hello world!';
let result = text.substr(7, 5);
document.getElementById('data').innerHTML = result;
JavaScript 中 substring()
和 substr()
的區別
你應該小心不要混淆 substring()
和 substr()
方法,因為它們之間存在細微差別。substring()
有兩個引數,開始和結束索引。
相比之下,substr()
有兩個引數,起始索引和返回字串中包含的字元數。
程式碼:
let text = 'BlocTAK'
console.log(text.substring(2, 5)) // => "ocT"
console.log(text.substr(2, 3)) // => "ocT"
JavaScript 中的萬用字元字串比較
單個字元,例如星號 (*
),是一個萬用字元,可以讀取為多個文字字元或空字串。它經常用於檔案搜尋,因為它消除了輸入完整名稱的需要。
使用文字和萬用字元模式實現萬用字元模式匹配方法,以檢視萬用字元模式是否與文字匹配。匹配應該一直貫穿文字。
這 ?
和 *
可用於萬用字元模式。
程式碼:
function match(first, second) {
if (first.length == 0 && second.length == 0) return true;
if (first.length > 1 && first[0] == '*' && second.length == 0) return false;
if ((first.length > 1 && first[0] == '?') ||
(first.length != 0 && second.length != 0 && first[0] == second[0]))
return match(first.substring(1), second.substring(1));
if (first.length > 0 && first[0] == '*')
return match(first.substring(1), second) ||
match(first, second.substring(1));
return false;
}
function test(first, second) {
if (match(first, second))
document.write(
'Yes' +
'<br>');
else
document.write(
'No' +
'<br>');
}
test('He*lo', 'Hello'); // Yes
test('He?lo*', 'HelloWorld'); // Yes
test('*pqrs', 'pqrst'); // No because 't' is not in first
test('abc*bcd', 'abcdhghgbcd'); // Yes
test('abc*c?d', 'abcd'); // No because second must have 2 instances of 'c'
在移至 Pattern and Text 中的下一個字元之前,萬用字元模式存在三種情況。
?
字元匹配任何單個字元。*
字元匹配任何字元序列。- 字元不是萬用字元。如果 Text 中的當前字元與 Pattern 中的當前字元匹配,我們將轉到 Pattern 和 Text 中的下一個字元。
上面的程式碼是一個字串匹配的例子,其中給出了一個包含萬用字元的字串,使用前兩種情況。
在 JavaScript 中使用 escapeRegex
函式
使用 escapeRegex
函式轉義正規表示式中具有任何特殊含義的任何字元。
程式碼:
function matchRuleShort(str, rule) {
var escapeRegex = (str) => str.replace(/([.*+?^=!:${}()|\[\]\/\\])/g, '\\$1');
return new RegExp('^' + rule.split('*').map(escapeRegex).join('.*') + '$')
.test(str);
}
function matchRuleExpl(str, rule) {
var escapeRegex = (str) => str.replace(/([.*+?^=!:${}()|\[\]\/\\])/g, '\\$1');
rule = rule.split('*').map(escapeRegex).join('.*');
rule = '^' + rule + '$'
var regex = new RegExp(rule);
return regex.test(str);
}
alert(
'1. ' + matchRuleShort('bird123', 'bird*') + '\n' +
'2. ' + matchRuleShort('123bird', '*bird') + '\n' +
'3. ' + matchRuleShort('123bird123', '*bird*') + '\n' +
'4. ' + matchRuleShort('bird123bird', 'bird*bird') + '\n' +
'5. ' + matchRuleShort('123bird123bird123', '*bird*bird*') + '\n' +
'6. ' + matchRuleShort('s[pe]c 3 re$ex 6 cha^rs', 's[pe]c*re$ex*cha^rs') +
'\n' +
'7. ' + matchRuleShort('should not match', 'should noo*oot match') + '\n');
escapeRegex
轉義正規表示式中包含任何特殊含義的任何字元,對於任何字串 grep(regexpEscape(string), string)
,它始終為 true
。
まとめ
在本文中,我們介紹了正規表示式及其物件的概念。然後我們關注字串方法 substr
和 substring
及其區別。
此外,我們在 JavaScript 中將這些字串方法 substr()
和 substring()
與萬用字元方法一起使用。最後,我們在 JavaScript 中使用 regexp
物件 true()
和 regexpEscape
函式使用萬用字元字串比較。