在 Javascript 中切換多個案例
-
在 Javascript 中使用 Fall Through 模型使用多個案例的
切換
-
使用 JavaScript 中的數學表示式處理
Switch
中的多個案例 -
使用 Javascript 中的字串表示式處理
Switch
中的多個case
-
使用 Javascript 中的邏輯表示式處理
Switch
中的多個案例
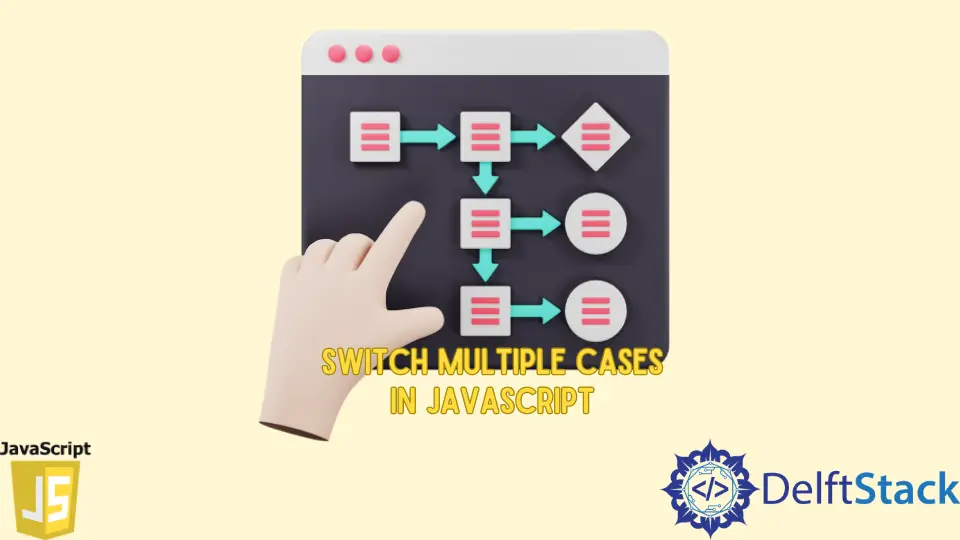
Switch case 的效能比 if-else
階梯高得多。它們有一個從變數值的直接對映,在 switch(variable)
塊中,到它們對應的值,在 case: value
塊中。我們熟悉單值對映的常見 switch-case
用法,但它可以支援多種情況嗎?讓我們看看在 javascript 中,我們可以在 switch-case
塊中有多個 case 的幾種方法。
在 Javascript 中使用 Fall Through 模型使用多個案例的切換
Fall through
模型是在 JavaScript 中使用多個 case
塊支援 switch
的第一步。在這裡,我們通過多種情況捕獲切換變數的多個值。我們將 break
關鍵字應用於一組有效的情況。例如,如果我們想根據過去的月份記錄一年的季度名稱,我們將根據季度對月份指數進行分組。參考以下程式碼。
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
}
function findQuarter(dateString) {
var monthIndex = new Date(dateString).getMonth();
switch (monthIndex) {
case 0:
case 1:
case 2:
console.log('First Quarter');
break;
case 3:
case 4:
case 5:
console.log('Second Quarter');
break;
case 6:
case 7:
case 8:
console.log('Third Quarter');
break;
case 9:
case 10:
case 11:
console.log('Fourth Quarter');
break;
}
}
輸出:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
findQuarter()
函式接受格式為 yyyy-mm-dd
的日期字串。然後它通過首先將其轉換為 javascript 日期物件,然後從日期物件中獲取月份索引,從 dateString
引數中提取月份索引。getMonth()
是一個 javascript 函式,它應用於 Date
物件並返回從 0
開始的月份索引。我們在這裡使用多個案例,一個堆疊在另一個下面以捕獲輸出的多個值。
例如,對於從 1 月到 3 月的月份,根據 getMonth()
函式返回的輸出,相應的月份索引的範圍是 0 到 2。我們在沒有 break
關鍵字的情況下將所有情況從 0
堆疊到 2
。因此,他們會絆倒並在遇到 break
時打破 switch-case
,該 break
恰好在 case 2:
行中,它記錄了值 First Quarter
。這組程式碼看起來很笨重。我們可以按如下方式重寫相同的內容以減少行數。
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
}
function findQuarter(dateString) {
var monthIndex = new Date(dateString).getMonth();
switch (monthIndex) {
case 0:
case 1:
case 2:
console.log('First Quarter');
break;
case 3:
case 4:
case 5:
console.log('Second Quarter');
break;
case 6:
case 7:
case 8:
console.log('Third Quarter');
break;
case 9:
case 10:
case 11:
console.log('Fourth Quarter');
break;
}
}
輸出:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
請注意,這裡的輸出保持不變。這只是編寫 Fall through
switch-case
模型的另一種方式。它使程式碼現在看起來更乾淨並節省空間。
使用 JavaScript 中的數學表示式處理 Switch
中的多個案例
我們正在按照前面部分中提到的示例處理一個月的數值。我們可以在 case
塊中使用 expressions
嗎?絕對是的!在解釋功能之前,讓我們參考以下程式碼。
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
findQuarter('2021-2-30');
}
function findQuarter(dateString) {
var monthIndex = new Date(dateString).getMonth();
switch (monthIndex) {
case ( (monthIndex < 2) ? monthIndex : -1 ):
console.log('First Quarter');
break;
case ( ((monthIndex > 2) && (monthIndex < 5)) ? monthIndex : -1):
console.log('Second Quarter');
break;
case ( ((monthIndex > 5) && (monthIndex < 8)) ? monthIndex : -1):
console.log('Third Quarter');
break;
case ( ((monthIndex > 8) && (monthIndex < 11)) ? monthIndex : -1):
console.log('Fourth Quarter');
break;
default:
console.log('Invalid date');
break;
}
}
輸出:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
如果我們在 switch case 中使用表示式,它們必須返回一個數字值,否則為 -1
。在上面的程式碼片段中,我們在 case
塊中新增了數字表示式而不是它們的實際值。這些表示式計算月份的相應季度並記錄季度名稱。在這裡,我們在 switch case
中包含了 default
子句來處理 Invalid date
。如果 date
中的 month
有效(在 1
到 12
的範圍內),JavaScript 中的 getMonth()
方法返回一個值(介於 0
和 11
之間),否則它將返回 NaN
。default
塊將捕獲此類無效日期場景。
使用 Javascript 中的字串表示式處理 Switch
中的多個 case
我們還可以在 case
塊中使用字串表示式。這些表示式在執行時計算,執行 case
子句。對於我們的月份示例,讓我們嘗試切換月份名稱。為此,我們將在日期物件上應用 toLocaleDateString()
函式。在函式的 options 引數中,我們給出 { month: 'long' }
。toLocaleDateString('en-US', { month: 'long' })
的輸出現在將是月份名稱,即完整的月份名稱。然後我們切換 toLocaleDateString('en-US', { month: 'long' })
函式的完整月份名稱輸出。
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
findQuarter('2021-21-30');
}
function findQuarter(dateString) {
var monthName =
new Date(dateString).toLocaleDateString('en-US', {month: 'long'});
switch (true) {
case['January', 'February', 'March'].includes(monthName):
console.log('First Quarter');
break;
case['April', 'May', 'June'].includes(monthName):
console.log('Second Quarter');
break;
case['July', 'August', 'September'].includes(monthName):
console.log('Third Quarter');
break;
case['October', 'November', 'December'].includes(monthName):
console.log('Fourth Quarter');
break;
default:
console.log('Invalid date');
break;
}
}
輸出:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
Invalid date
在上面的程式碼中,我們使用了 switch(true)
語句。在 case
子句中,我們有字串比較。我們為每個季度建立了一個臨時的月份陣列,並將它們新增到每個案例
中。然後我們檢查 monthName
在季度陣列中是否可用。如果找到,它將記錄季度的名稱。如果日期無效,toLocaleDateString('en-US', { month: 'long' })
函式將輸出 Invalid Date
。這個場景被 default
塊捕獲,因為 Invalid Date
字串將與儲存季度月份名稱的預定義陣列不匹配。因此,我們在這裡順利處理無效日期的情況。
.indexOf()
代替 .includes()
或 find()
函式來支援多個瀏覽器。Internet Explorer 不支援 .includes()
和 find()
函式。使用 Javascript 中的邏輯表示式處理 Switch
中的多個案例
正如我們在數學和字串表示式中看到的那樣,我們還可以在 switch-case
塊中的 multiple cases
中使用邏輯運算子。在月份示例中,我們將使用邏輯 OR
運算子 ||
用於確定月份名稱是否在特定季度中。讓我們參考以下程式碼。
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
findQuarter('2021-21-30');
}
function findQuarter(dateString) {
var monthName =
new Date(dateString).toLocaleDateString('en-US', {month: 'long'});
switch (true) {
case (
monthName === 'January' || monthName === 'February' ||
monthName === 'March'):
console.log('First Quarter');
break;
case (monthName === 'April' || monthName === 'May' || monthName === 'June'):
console.log('Second Quarter');
break;
case (
monthName === 'July' || monthName === 'August' ||
monthName === 'September'):
console.log('Third Quarter');
break;
case (
monthName === 'October' || monthName === 'November' ||
monthName === 'December'):
console.log('Fourth Quarter');
break;
default:
console.log('Invalid date');
break;
}
}
輸出:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
Invalid date
在程式碼中,我們檢查 monthName
值是否等於一個季度的月份。因此,我們安慰季度的名稱。在這裡,我們也處理無效日期的情況,因為它不滿足 case
子句中編碼的任何條件,並且會落入 default
塊,然後將 Invalid date
列印到瀏覽器控制檯。