在 Java 中讀取 JSON 檔案
Siddharth Swami
2023年10月12日
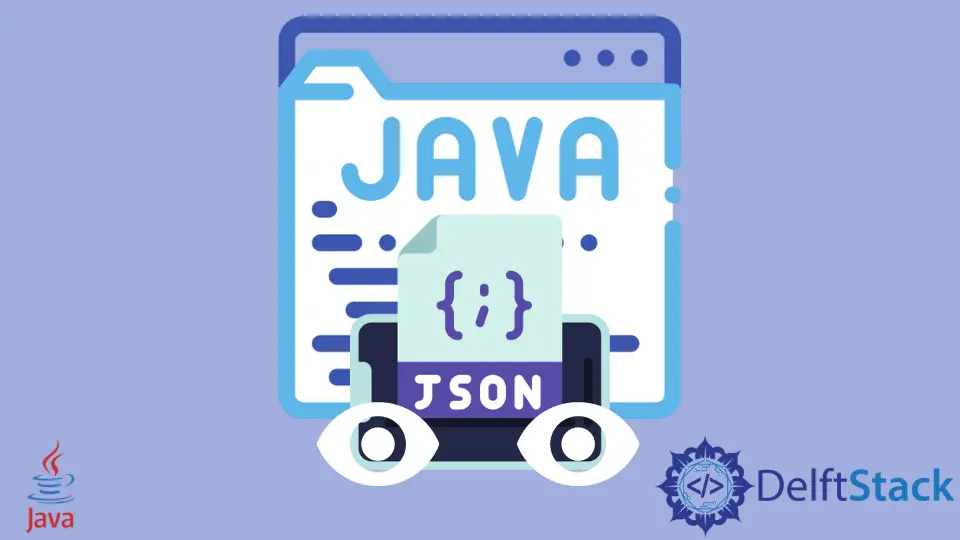
JavaScript Object Notation 是一種輕量級的基於文字的語言,用於儲存和傳輸資料。物件和陣列是由 JSON 檔案表示的兩種資料型別。
本教程演示瞭如何在 Java 中讀取 JSON 檔案。
要讀取 JSON 檔案,我們將使用 FileReader()
函式來啟動一個 FileReader
物件並讀取給定的 JSON 檔案。
在我們的示例中,我們將讀取以下檔案。
{
"firstName": "Ram",
"lastName": "Sharma",
"age": 26
},
"phoneNumbers": [
{
"type": "home",
"phone-number": "212 888-2365"
}
]
}
為了解析這個檔案的內容,我們將使用 json.simple
java 庫。我們需要從 java.simple
庫中匯入兩個類,org.json.simple.JSONArray
和 org.json.simple.JSONObject
類。JSONArray
幫助我們以陣列的形式讀取元素,而 JSONObject
幫助我們讀取 JSON 文字中存在的物件。
在下面的程式碼中,我們讀取了系統上已經存在的 JSON 檔案,然後我們將列印檔案中的名字和姓氏。
例如,
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import org.json.simple.parser.*;
public class JSONsimple {
public static void main(String[] args) throws Exception {
// parsing file "JSONExample.json"
Object ob = new JSONParser().parse(new FileReader("JSONFile.json"));
// typecasting ob to JSONObject
JSONObject js = (JSONObject) ob;
String firstName = (String) js.get("firstName");
String lastName = (String) js.get("lastName");
System.out.println("First name is: " + firstName);
System.out.println("Last name is: " + lastName);
}
}
輸出:
First name is: Ram
Last name is: Sharma
我們可以在上面的例子中看到我們從 JSON 檔案中讀取的名字和姓氏。
這裡我們使用了 Java 中 org.json.simple.parser.*
類中的 JSONParser().parse()
,它解析檔案中的 JSON 文字。此處的 js.get()
方法從檔案中獲取名字和姓氏。
我們可以使用不同的方法解析 JSON 內容,但使用 FileReader()
讀取主檔案。
我們也可以直接從字串解析 JSON。還有其他方法可以解析 JSON 字串。
下面的程式碼演示了 org.json
方法。在這裡,我們將複製並貼上靜態字串 json
中的完整檔案。然後我們將建立一個物件,該物件將用於從檔案中讀取 JSON 物件和陣列。
import org.json.JSONArray;
import org.json.JSONObject;
public class JSON2 {
static String json = "{\"contacDetails\": {\n" + // JSON text in the file is written here
" \"firstName\": \"Ram\",\n"
+ " \"lastName\": \"Sharma\"\n"
+ " },\n"
+ " \"phoneNumbers\": [\n"
+ " {\n"
+ " \"type\": \"home\",\n"
+ " \"phone-number\": \"212 888-2365\",\n"
+ " }\n"
+ " ]"
+ "}";
public static void main(String[] args) {
// Make a object
JSONObject ob = new JSONObject(json);
// getting first and last name
String firstName = ob.getJSONObject("contacDetails").getString("firstName");
String lastName = ob.getJSONObject("contacDetails").getString("lastName");
System.out.println("FirstName " + firstName);
System.out.println("LastName " + lastName);
// loop for printing the array as phoneNumber is stored as array.
JSONArray arr = obj.getJSONArray("phoneNumbers");
for (int i = 0; i < arr.length(); i++) {
String post_id = arr.getJSONObject(i).getString("phone-number");
System.out.println(post_id);
}
}
}
輸出:
FirstName Ram
LastName Sharma
212 888-2365