如何在 Java 中讀取 XML 檔案
Mohammad Irfan
2023年10月12日
Java
Java File
- 樣本 XML 檔案
-
使用 Java 中的
DocumentBuilderFactory
讀取 XML 檔案 - 讀取 Java POJO 中的 XML 檔案
-
在 Java 中使用
jdom2
讀取 XML 檔案 -
在 Java 中使用
XPath
讀取 XML 檔案 -
在 Java 中使用
DOM4J
讀取 XML 檔案
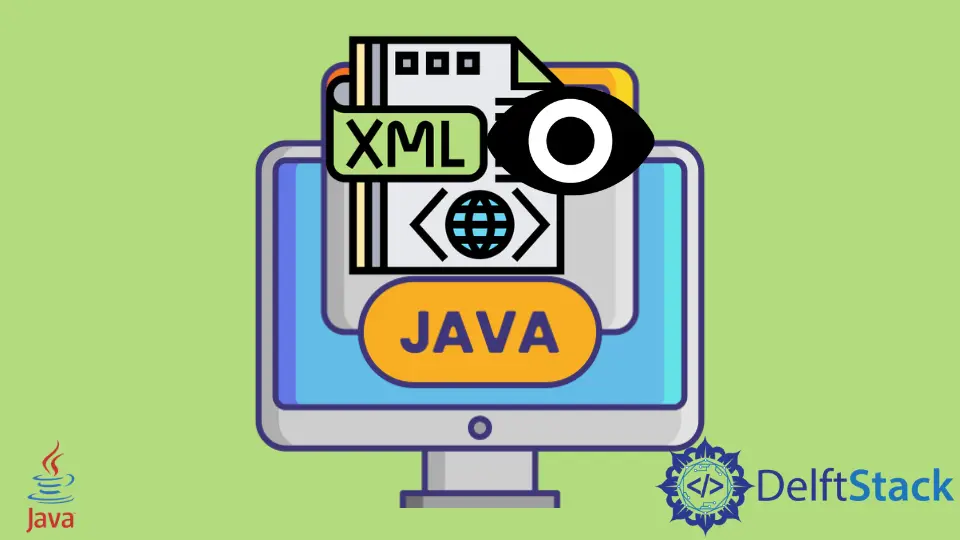
在本文中,我們將學習如何通過使用諸如 DOM Parser
,XML Parser
,jdom2
,dom4j
之類的某些庫將 XML 檔案解析為 Java 應用程式。XML 代表 Extensible Markup Language
,用於在應用程式中傳遞資料。
樣本 XML 檔案
這是本文將用來讀取的一個 XML 檔案示例。
<?xml version="1.0"?>
<company>
<employee id="1001">
<firstname>Tony</firstname>
<lastname>Black</lastname>
<salary>100000</salary>
</employee>
<employee id="2001">
<firstname>Amy</firstname>
<lastname>Green</lastname>
<salary>200000</salary>
</employee>
</company>
使用 Java 中的 DocumentBuilderFactory
讀取 XML 檔案
我們使用 DocumentBuilder
為構建器建立一個例項,然後使用 parse()
方法解析 XML 檔案。getElementsByTagName()
方法獲取 XML 的每個節點,然後使用 for
迴圈對節點的每個子節點進行迭代。請參見下面的示例。
import java.io.File;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class SimpleTesting {
public static void main(String[] args) throws ParserConfigurationException, SAXException {
try {
File file = new File("company.xml");
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document document = db.parse(file);
document.getDocumentElement().normalize();
System.out.println("Root Element :" + document.getDocumentElement().getNodeName());
NodeList nList = document.getElementsByTagName("employee");
System.out.println("----------------------------");
for (int temp = 0; temp < nList.getLength(); temp++) {
Node nNode = nList.item(temp);
System.out.println("\nCurrent Element :" + nNode.getNodeName());
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) nNode;
System.out.println("Employee id : " + eElement.getAttribute("id"));
System.out.println("First Name : "
+ eElement.getElementsByTagName("firstname").item(0).getTextContent());
System.out.println(
"Last Name : " + eElement.getElementsByTagName("lastname").item(0).getTextContent());
System.out.println(
"Salary : " + eElement.getElementsByTagName("salary").item(0).getTextContent());
}
}
} catch (IOException e) {
System.out.println(e);
}
}
}
輸出:
Root Element :company
----------------------------
Current Element :employee
Employee id : 1001
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
Employee id : 2001
First Name : Amy
Last Name : Green
Salary : 200000
讀取 Java POJO 中的 XML 檔案
如果要將 XML 資料轉換為 Java 相容型別,則可以使用 Java POJO 讀取資料。在這裡,我們使用 ArrayList
型別的 Employee 通過 add()
方法新增每個節點,然後使用 for
迴圈迭代每個物件。請參見以下示例。
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(new File("employee.xml"));
List<Employee> employees = new ArrayList<>();
NodeList nodeList = document.getDocumentElement().getChildNodes();
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node.getNodeType() == Node.ELEMENT_NODE) {
Element elem = (Element) node;
String firstname =
elem.getElementsByTagName("firstname").item(0).getChildNodes().item(0).getNodeValue();
String lastname =
elem.getElementsByTagName("lastname").item(0).getChildNodes().item(0).getNodeValue();
Double salary = Double.parseDouble(
elem.getElementsByTagName("salary").item(0).getChildNodes().item(0).getNodeValue());
employees.add(new Employee(firstname, lastname, salary));
}
}
for (Employee empl : employees) System.out.println(empl.toString());
}
}
class Employee {
private String Firstname;
private String Lastname;
private double salary;
public Employee(String Firstname, String Lastname, double salary) {
this.Firstname = Firstname;
this.Lastname = Lastname;
this.salary = salary;
}
@Override
public String toString() {
return "[" + Firstname + ", " + Lastname + ", " + salary + "]";
}
}
輸出:
[Tony, Black, 100000.0]
[Amy, Green, 200000.0]
在 Java 中使用 jdom2
讀取 XML 檔案
jdom2 是一個使用 Java 類提供對 DOM 解析的支援的庫。我們使用了 SAXBuilder
類和 build()
方法來將資料獲取到 Document
中,然後使用 getRootElement()
方法來獲取元素。請參見下面的示例。
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import org.jdom2.Document;
import org.jdom2.Element;
import org.jdom2.JDOMException;
import org.jdom2.input.SAXBuilder;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("/employee.xml");
SAXBuilder saxBuilder = new SAXBuilder();
Document document = saxBuilder.build(inputFile);
System.out.println("Root element :" + document.getRootElement().getName());
Element classElement = document.getRootElement();
List<Element> studentList = classElement.getChildren();
System.out.println("----------------------------");
for (int temp = 0; temp < studentList.size(); temp++) {
Element student = studentList.get(temp);
System.out.println("\nCurrent Element :" + student.getName());
System.out.println("First Name : " + student.getChild("firstname").getText());
System.out.println("Last Name : " + student.getChild("lastname").getText());
System.out.println("Salary : " + student.getChild("salary").getText());
}
} catch (JDOMException e) {
e.printStackTrace();
} catch (IOException ioe) {
ioe.printStackTrace();
}
}
}
輸出:
Root element :company
----------------------------
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000
在 Java 中使用 XPath
讀取 XML 檔案
在這裡,我們使用 Java 中的 XPath
庫來解析 XML 檔案。XPathFactory
類用於將所有節點編譯為 NodeList
,然後通過 for 迴圈迭代每個子節點。請參見下面的示例。
import java.io.File;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathExpressionException;
import javax.xml.xpath.XPathFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("/employee.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder;
dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inputFile);
doc.getDocumentElement().normalize();
XPath xPath = XPathFactory.newInstance().newXPath();
String expression = "/company/employee";
NodeList nodeList =
(NodeList) xPath.compile(expression).evaluate(doc, XPathConstants.NODESET);
for (int i = 0; i < nodeList.getLength(); i++) {
Node nNode = nodeList.item(i);
System.out.println("\nCurrent Element :" + nNode.getNodeName());
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) nNode;
System.out.println("First Name : "
+ eElement.getElementsByTagName("firstname").item(0).getTextContent());
System.out.println(
"Last Name : " + eElement.getElementsByTagName("lastname").item(0).getTextContent());
System.out.println(
"Salary : " + eElement.getElementsByTagName("salary").item(0).getTextContent());
}
}
} catch (ParserConfigurationException e) {
System.out.println(e);
} catch (SAXException e) {
System.out.println(e);
} catch (IOException e) {
System.out.println(e);
} catch (XPathExpressionException e) {
System.out.println(e);
}
}
}
輸出:
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000
在 Java 中使用 DOM4J
讀取 XML 檔案
Dom4j
是另一個可以解析 Java 中 XML 檔案的庫。SAXReader 類的 read()
方法用於將節點讀入文件。請參見下面的示例。
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.Node;
import org.dom4j.io.SAXReader;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("employee.xml");
SAXReader reader = new SAXReader();
Document document = reader.read(inputFile);
System.out.println("Root element :" + document.getRootElement().getName());
Element classElement = document.getRootElement();
List<Node> nodes = document.selectNodes("company/employee");
System.out.println("----------------------------");
for (Node node : nodes) {
System.out.println("\nCurrent Element :" + node.getName());
System.out.println("First Name : " + node.selectSingleNode("firstname").getText());
System.out.println("Last Name : " + node.selectSingleNode("lastname").getText());
System.out.println("Salary : " + node.selectSingleNode("salary").getText());
}
} catch (DocumentException e) {
e.printStackTrace();
}
}
}
輸出:
Root element :company
----------------------------
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe