在 Java 中顯示影象
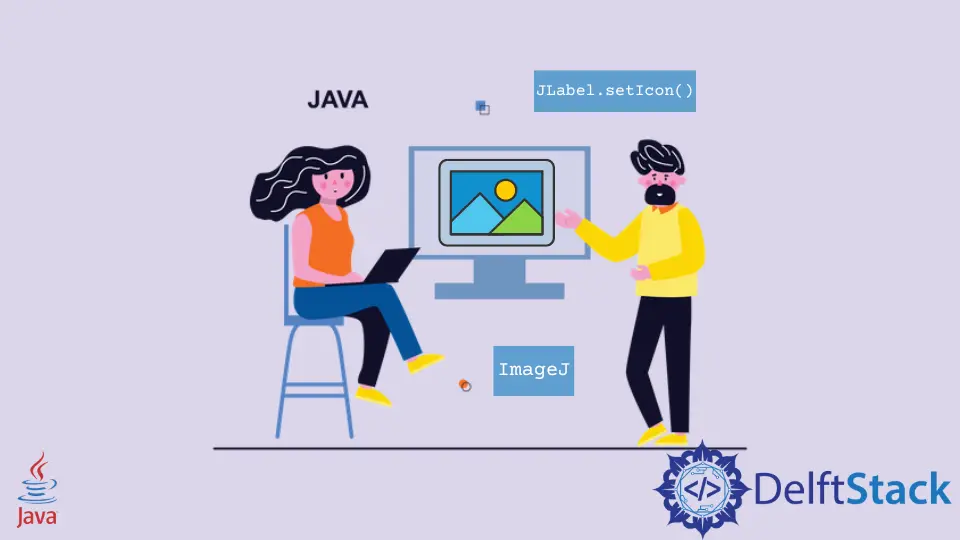
我們可以在 Java 中使用多種方式顯示影象。下面我們將看到如何使用兩種方法在 Java 中顯示影象。
在 Java 中使用 JLabel.setIcon()
顯示影象
在第一個示例中,我們使用 Swing 庫的 JLabel
類。JLabel
擴充套件了 JComponent
,我們可以將此元件附加到 JFrame
。為了讀取影象檔案,我們使用 File
類並傳遞影象的路徑。接下來,我們使用 ImageIO.read()
將影象轉換為 BufferedImage
物件。現在我們建立一個要顯示在 JLabel
中的圖示。
為了顯示標籤圖示,我們需要一個帶有 FlowLayout
和 500 x 500 大小的 JFrame
物件。大小可以根據我們的需要進行調整。現在我們建立一個 JLabel
物件並使用 JLabel.setIcon()
函式設定它的圖示。然後我們將 jLabel
元件新增到 jFrame
並將框架的可見性設定為 true。
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.*;
public class DisplayImage {
public static void main(String[] args) throws IOException {
File file = new File("C:\\Users\\User1\\Pictures\\Camera Roll\\java.png");
BufferedImage bufferedImage = ImageIO.read(file);
ImageIcon imageIcon = new ImageIcon(bufferedImage);
JFrame jFrame = new JFrame();
jFrame.setLayout(new FlowLayout());
jFrame.setSize(500, 500);
JLabel jLabel = new JLabel();
jLabel.setIcon(imageIcon);
jFrame.add(jLabel);
jFrame.setVisible(true);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
輸出:
在 Java 中使用 ImageJ
庫顯示影象
在這個程式中,我們使用了一個名為 ImageJ
的強大的影象處理庫。要使用它,我們首先將 maven 倉庫和依賴項匯入 pom.xml
。
<repositories>
<repository>
<id>scijava.public</id>
<url>https://maven.scijava.org/content/groups/public</url>
</repository>
</repositories>
<dependency>
<groupId>net.imagej</groupId>
<artifactId>ij</artifactId>
<version>1.53j</version>
</dependency>
我們的目標是顯示影象,而 ImageJ
使我們變得簡單。以下是我們首先從類 IJ
呼叫靜態函式 openImage()
並將其中影象的路徑作為引數傳遞的程式碼。請注意,我們只寫了影象名稱及其副檔名,因為我們的影象在同一目錄中。
IJ.openImage()
返回一個 ImagePlus
物件。現在我們使用 imagePlus
物件呼叫 show()
方法。我們可以看到輸出顯示了一個幀中的影象。
import ij.IJ;
import ij.ImagePlus;
public class DisplayImage {
public static void main(String[] args) {
ImagePlus imagePlus = IJ.openImage("mountains.jpeg");
imagePlus.show();
}
}
輸出:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn相關文章 - Java JLabel
- 在 Java Swing 中更改 JLabel 文字
- 在 Swing 中將 JLabel 居中
- 在 Java 中使用 setFont
- Java 中的 JFileChooser 示例
- 在 Java 中將 ActionListener 新增到 JButton