如何在 Go 中將字串轉換為整數型別
Suraj Joshi
2023年1月30日
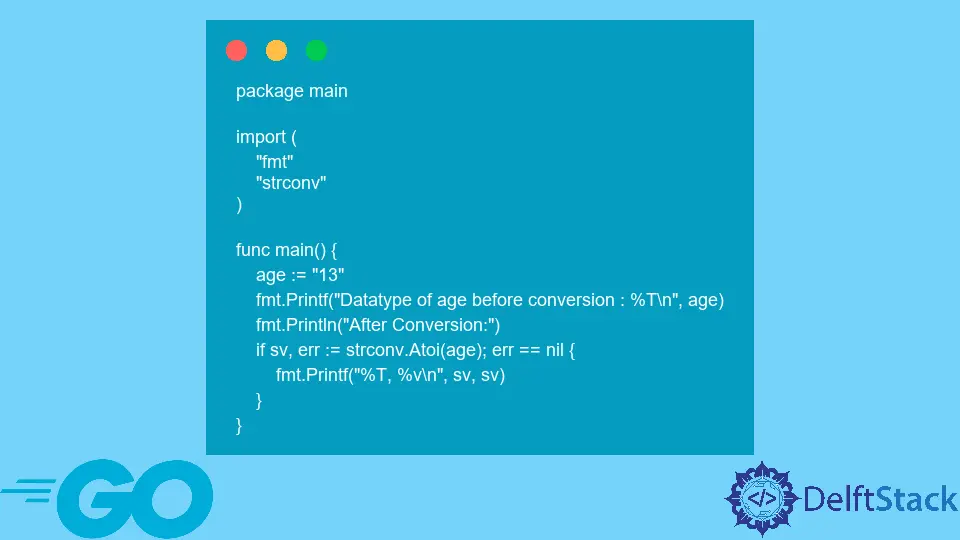
Go 是靜態型別的語言,如果我們將變數定義為整型,則它只能為整型。我們不能在不轉換變數的字串資料型別的情況下為其分配字串。Go 有一個名為 strconv
的內建包,它提供了三個函式 Atoi()
,Sscanf()
和 ParseInt()
將 Go 字串轉換為整型 int
。
來自 strconv
包的 Atoi()
函式
strconv
包實現了基本資料型別的字串表示之間的轉換。要將字串轉換為整數,我們使用來自 strconv
包的 Atoi()
函式。Atoi
代表 ASCII to integer
。
package main
import (
"fmt"
"strconv"
)
func main() {
age := "13"
fmt.Printf("Datatype of age before conversion : %T\n", age)
fmt.Println("After Conversion:")
if sv, err := strconv.Atoi(age); err == nil {
fmt.Printf("%T, %v\n", sv, sv)
}
}
輸出:
Datatype of age before conversion : string
After Conversion:
int, 13
如果輸入字串不是整數格式,則該函式返回零。
package main
import (
"fmt"
"strconv"
)
func main() {
age := "abc"
fmt.Printf("Datatype of age before conversion : %T\n", age)
fmt.Println("After Conversion:")
sv, _ := strconv.Atoi(age)
fmt.Printf("%T, %v\n", sv, sv)
}
輸出:
Datatype of age before conversion : string
After Conversion:
int, 0
來自 strconv
包的 ParseInt()
函式
strconv.ParseInt(s, base, bitSize)
方法以給定的 base (0,2 到 36)和位大小(0 到 64)解釋輸入字串 s
並返回一個對應的整數。Atoi(s)
等同於 ParseInt(s, 10, 0)
。
package main
import (
"fmt"
"reflect"
"strconv"
)
func main() {
x := "123"
fmt.Println("Value of x before conversion: ", x)
fmt.Println("Datatype of x before conversion:", reflect.TypeOf(x))
intStr, _ := strconv.ParseInt(x, 10, 64)
fmt.Println("Value after conversion: ", intStr)
fmt.Println("Datatype after conversion:", reflect.TypeOf(intStr))
}
輸出:
Value of x before conversion: 123
Datatype of x before conversion: string
Value after conversion: 123
Datatype after conversion: int64
fmt.Sscanf
方法
fmt.Sscanf()
非常適合解析包含數字的自定義字串。Sscanf()
函式掃描引數字串,將連續的以空格分隔的值儲存到格式定義的連續引數中,並返回成功解析的專案數。
package main
import (
"fmt"
)
func main() {
s := "id:00234"
var i int
if _, err := fmt.Sscanf(s, "id:%5d", &i); err == nil {
fmt.Println(i)
}
}
輸出:
234
方法比較
strconv.ParseInt()
是所有方法中最快的,strconv.Atoi()
比 strconv.ParseInt()
要慢一點,而 fmt.Sscanf()
是除最靈活方法之外最慢的方法。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn