在 Go 中檢查檔案是否存在
Jay Singh
2022年4月22日
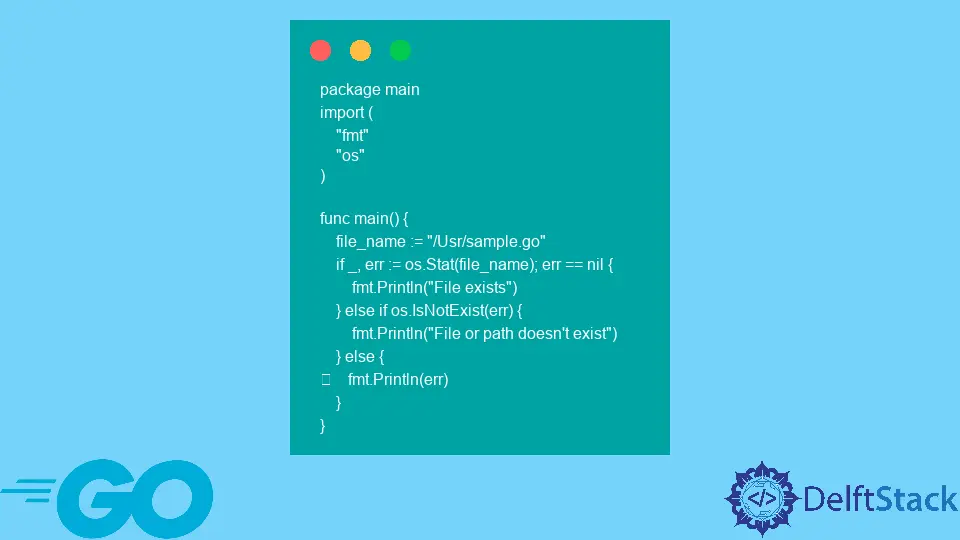
本文將討論在 Go 中檢查檔案是否存在。
在 Go 中使用 IsNotExist()
和 Stat()
檢查檔案是否存在
我們使用 Go 程式語言中 os
包中的 IsNotExist()
和 Stat()
方法來確定檔案是否存在。
Stat()
函式返回一個包含檔案資訊的物件。如果檔案不存在,它會產生一個錯誤物件。
下面是使用 IsNotExist()
和 Stat()
的程式碼示例。
示例 1:
package main
import (
"fmt"
"os"
)
// function to check if file exists
func doesFileExist(fileName string) {
_, error := os.Stat(fileName)
// check if error is "file not exists"
if os.IsNotExist(error) {
fmt.Printf("%v file does not exist\n", fileName)
} else {
fmt.Printf("%v file exist\n", fileName)
}
}
func main() {
// check if demo.txt exists
doesFileExist("demo.txt")
// check if demo.csv exists
doesFileExist("demo.csv")
}
輸出:
demo.txt file exist
demo.csv file does not exist
示例 2:
package main
import (
"fmt"
"os"
)
func main() {
file_name := "/Usr/sample.go"
if _, err := os.Stat(file_name); err == nil {
fmt.Println("File exists")
} else if os.IsNotExist(err) {
fmt.Println("File or path doesn't exist")
} else {
fmt.Println(err)
}
}
輸出:
File or path doesn't exist