如何在 C# 函式中傳遞一個方法作為引數
Minahil Noor
2024年2月16日
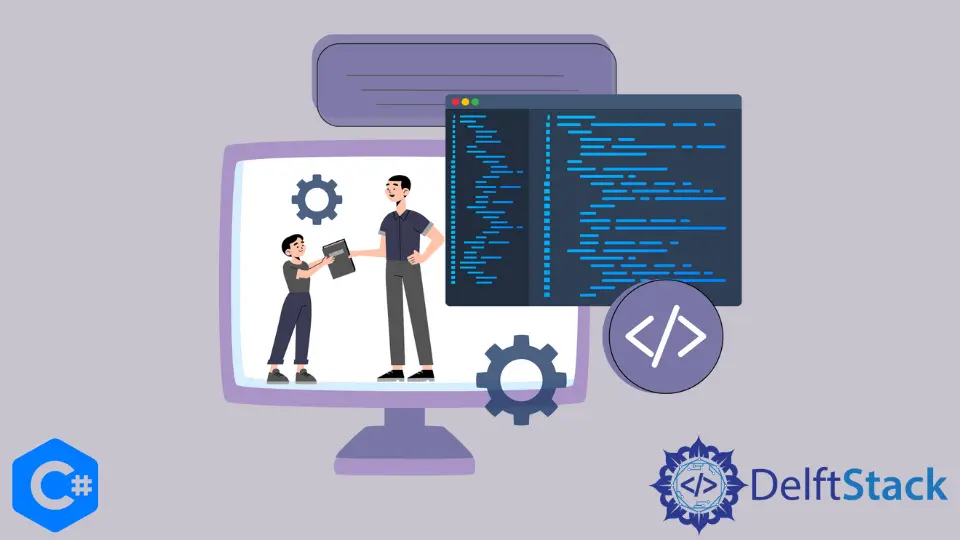
本文將介紹在 C# 函式中傳遞一個方法作為引數的不同方法。
- 使用
Func
委託 - 使用
Action
代表
在 C# 中使用 Func
代表傳遞一個方法作為引數
我們將使用內建的 Func
delegate 來傳遞一個方法作為引數。delegate
的作用就像一個函式指標。使用這個委託的正確語法如下。
public delegate returnType Func<in inputType, out returnType>(InputType arg);
內建的委派 Func
有 N 個引數。它的引數詳情如下。
引數 | 說明 | |
---|---|---|
inputType |
強制 | 它是這個委託的輸入值的型別,可以有 N 個輸入。 |
returnType |
強制 | 它是返回值的型別。該委託的最後一個值被視為是返回型別。 |
下面的程式顯示了我們如何使用 Func
委託來傳遞一個方法作為引數。
public class MethodasParameter {
public int Method1(string input) {
return 0;
}
public int Method2(string input) {
return 1;
}
public bool RunMethod(Func<string, int> MethodName) {
int i = MethodName("This is a string");
return true;
}
public bool Test() {
return RunMethod(Method1);
}
}
在 C# 中使用 Action
委派來傳遞一個方法作為引數
我們也可以使用內建的委託 Action
來傳遞一個方法作為引數。使用這個委託的正確語法如下。
public delegate void Action<in T>(T obj);
內建的委託人 Action
可以有 16 個引數作為輸入。它的詳細引數如下。
引數 | 說明 | |
---|---|---|
T |
強制 | 它是這個委託的輸入值的型別。可以有 16 個輸入值。 |
下面的程式顯示了我們如何使用 Action
委託來傳遞一個方法作為引數。
public class MethodasParameter {
public int Method1(string input) {
return 0;
}
public int Method2(string input) {
return 1;
}
public bool RunTheMethod(Action myMethodName) {
myMethodName();
return true;
}
RunTheMethod(() => Method1("MyString1"));
}