在 C++ 中從陣列中刪除元素
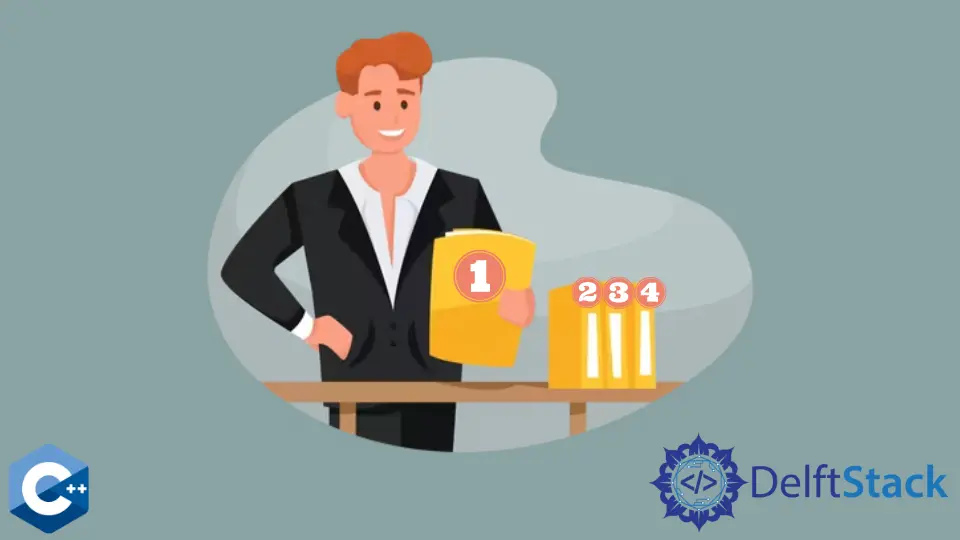
本文將介紹幾種在 C++ 中如何從陣列中刪除元素的方法。
從 C++ 中使用 std::to_array
和 std::remove
函式從陣列中刪除元素
陣列可以在 C++ 中定義為定長或動態陣列,並且它們都需要使用不同的方法來刪除元素。在此示例中,我們考慮內建的 C 樣式固定陣列,因為這些陣列通常由數字程式操縱以提高效率。
我們將宣告一個 int
陣列,並刪除元素值 2
,該元素值在該陣列中出現兩次。std::remove
是演算法庫的一部分,它刪除指定範圍內給定元素的所有例項。
儘管起初,我們使用 std::to_array
函式將 arr
物件轉換為 std::array
容器,以便安全地與 std::remove
方法一起使用。後一種演算法返回範圍的新末端的迭代器,這意味著所得的陣列
物件仍包含 10
個元素,我們需要將其複製到新位置。由於原始物件是 C 樣式的陣列,因此我們為 8 個元素的 int
陣列分配了新的動態記憶體,並使用了 std::memmove
函式從 array
物件中複製了內容。請注意,我們使用 std::distance
函式計算了 8
值。
#include <array>
#include <cstring>
#include <iostream>
#include <iterator>
using std::array;
using std::cout;
using std::endl;
using std::remove;
int main() {
int arr[10] = {1, 1, 1, 2, 2, 6, 7, 8, 9, 10};
int elem_to_remove = 2;
cout << "| ";
for (const auto &item : arr) {
cout << item << " | ";
}
cout << endl;
auto tmp = std::to_array(arr);
auto len =
std::distance(tmp.begin(), (tmp.begin(), tmp.end(), elem_to_remove));
auto new_arr = new int[len];
std::memmove(new_arr, tmp.data(), len * sizeof(int));
cout << "| ";
for (int i = 0; i < len; ++i) {
cout << new_arr[i] << " | ";
}
cout << endl;
delete[] new_arr;
return EXIT_SUCCESS;
}
輸出:
| 1 | 1 | 1 | 2 | 2 | 6 | 7 | 8 | 9 | 10 |
| 1 | 1 | 1 | 6 | 7 | 8 | 9 | 10 |
使用 std::erase
和 std::remove
函式從 C++ 中的陣列中刪除元素
當給定陣列的型別為 std::vector
時,會發生此問題的另一種情況。這次,我們有了動態陣列特性,使用內建函式進行元素操作更加靈活。
在下面的示例程式碼中,我們將使用擦除-刪除
慣用語並刪除範圍中給定元素的所有出現。請注意,std::erase
需要兩個迭代器來表示要刪除的範圍。因此,它需要 std::remove
演算法的返回值來指定起點。請注意,如果僅呼叫 std::remove
方法,則 arr2
物件的元素將是 - {1, 1, 1, 6, 7, 8, 9, 10, 9, 10}
。
#include <iostream>
#include <iterator>
#include <vector>
using std::cout;
using std::endl;
using std::remove;
using std::vector;
int main() {
vector<int> arr2 = {1, 1, 1, 2, 2, 6, 7, 8, 9, 10};
int elem_to_remove = 2;
cout << "| ";
for (const auto &item : arr2) {
cout << item << " | ";
}
cout << endl;
arr2.erase(std::remove(arr2.begin(), arr2.end(), elem_to_remove), arr2.end());
cout << "| ";
for (const auto &item : arr2) {
cout << item << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
輸出:
| 1 | 1 | 1 | 2 | 2 | 6 | 7 | 8 | 9 | 10 |
| 1 | 1 | 1 | 6 | 7 | 8 | 9 | 10 |
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu