在 C++ 中查詢字串的長度
Jinku Hu
2023年10月12日
C++
C++ String
-
使用
length
函式在 C++ 中查詢字串的長度 -
使用
size
函式在 C++ 中查詢字串的長度 -
使用
while
迴圈在 C++ 中查詢字串的長度 -
使用
std::strlen
函式在 C++ 中查詢字串的長度
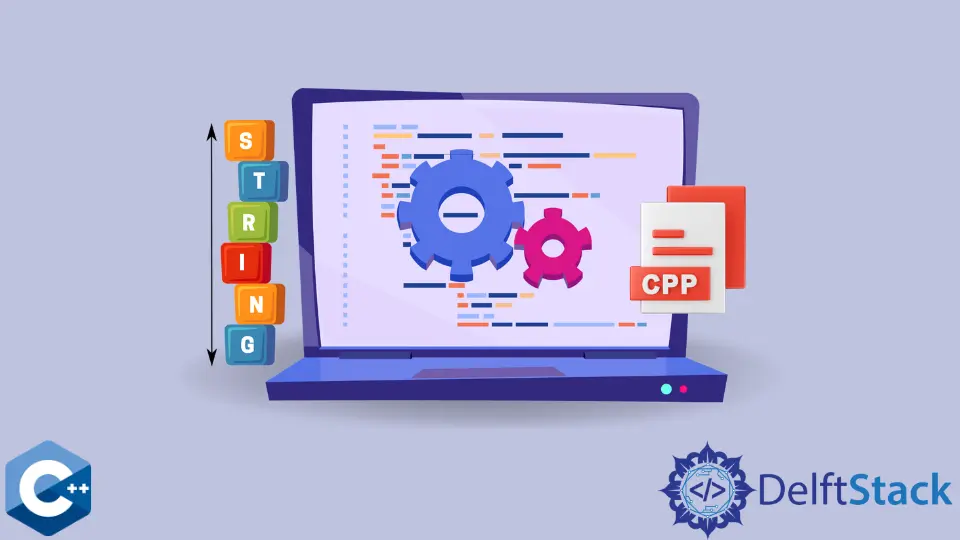
本文將介紹幾種如何在 C++ 中查詢字串長度的方法。
使用 length
函式在 C++ 中查詢字串的長度
C++ 標準庫提供了 std::basic_string
類,以擴充套件類似於 char
的序列,並實現了用於儲存和處理此類資料的通用結構。雖然,大多數人對 std::string
型別更熟悉,後者本身就是 std::basic_string<char>
的型別別名。std::string
提供了 length
內建函式來檢索儲存的 char
序列的長度。
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << str1.length() << endl;
exit(EXIT_SUCCESS);
}
輸出:
string: this is random string oiwaoj
length: 28
使用 size
函式在 C++ 中查詢字串的長度
std::string
類中包含的另一個內建函式是 size
,其行為與以前的方法相似。它不帶引數,並返回字串物件中 char
元素的數量。
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << str1.size() << endl;
exit(EXIT_SUCCESS);
}
輸出:
string: this is random string oiwaoj
length: 28
使用 while
迴圈在 C++ 中查詢字串的長度
或者,可以實現自己的函式來計算字串的長度。在這種情況下,我們利用 while
迴圈將字串作為 char
序列遍歷,並在每次迭代時將計數器加 1。注意,該函式將 char*
作為引數,並呼叫了 c_str
方法以在主函式中檢索此指標。當取消引用的指標值等於 0
時,迴圈停止,並且以 null 終止的字串實現保證了這一點。
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
size_t lengthOfString(const char *s) {
size_t size = 0;
while (*s) {
size += 1;
s += 1;
}
return size;
}
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << lengthOfString(str1.c_str()) << endl;
exit(EXIT_SUCCESS);
}
輸出:
string: this is random string oiwaoj
length: 28
使用 std::strlen
函式在 C++ 中查詢字串的長度
最後,可以使用老式的 C 字串庫函式 strlen
,該函式將單個 const char*
引數作為我們自定義的函式-lengthOfString
。當在不以空位元組結尾的 char
序列上呼叫時,這後兩種方法可能會出錯,因為它可能在遍歷期間訪問超出範圍的記憶體。
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << std::strlen(str1.c_str()) << endl;
exit(EXIT_SUCCESS);
}
輸出:
string: this is random string oiwaoj
length: 28
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu