如何在 C++ 中把字串轉換為 Char 陣列
Jinku Hu
2023年10月12日
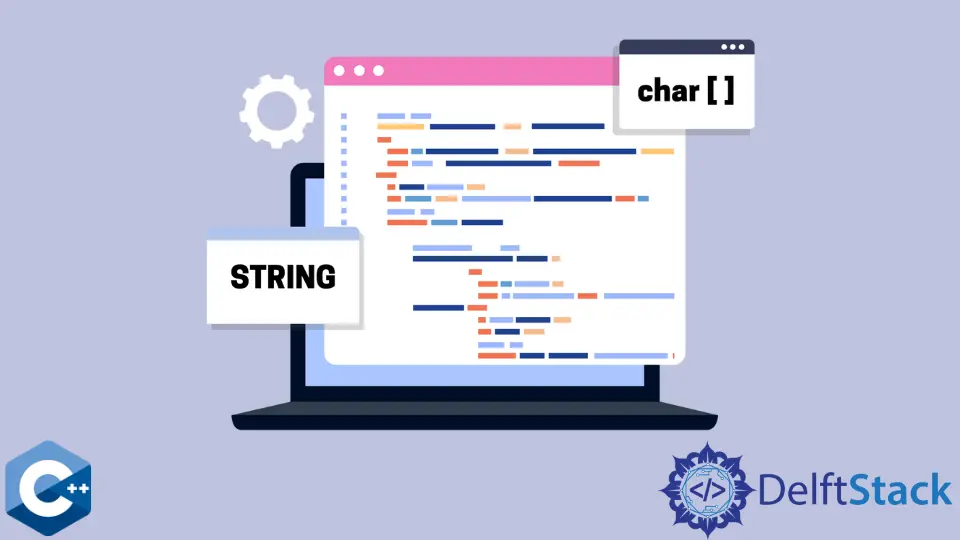
本文介紹了將字串資料轉換為 char
陣列的多種方法。
使用 std::basic_string::c_str
方法將字串轉換為 char 陣列
這個版本是解決上述問題的 C++ 方法。它使用了 string
類內建的方法 c_str
,該方法返回一個指向以 null
終止的 char 陣列的指標。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*";
auto c_string = tmp_string.c_str();
cout << c_string << endl;
return EXIT_SUCCESS;
}
輸出:
This will be converted to char*
如果你打算修改儲存在 c_str()
方法返回的指標處的資料,首先,你必須用 memmove
函式把這個範圍複製到不同的位置,然後對 char 字串進行如下操作。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*";
char *c_string_copy = new char[tmp_string.length() + 1];
memmove(c_string_copy, tmp_string.c_str(), tmp_string.length());
/* do operations on c_string_copy here */
cout << c_string_copy;
delete[] c_string_copy;
return EXIT_SUCCESS;
}
注意,你可以使用各種函式複製 c_string
資料,例如:[memcpy
]。memcpy
, memccpy
, mempcpy
, strcpy
或 strncpy
, 但請記住閱讀手冊頁面並仔細考慮其邊緣情況/錯誤。
使用 std::vector
容器將字串轉換為 Char 陣列
另一種方法是將字串中的字元儲存到 vector
容器中,然後使用其強大的內建方法安全地運算元據。
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string tmp_string = "This will be converted to char*\n";
vector<char> vec_str(tmp_string.begin(), tmp_string.end());
std::copy(vec_str.begin(), vec_str.end(),
std::ostream_iterator<char>(cout, ""));
return EXIT_SUCCESS;
}
使用指標操作操作將字串轉換為字元陣列
在這個版本中,我們定義了一個名為 tmp_ptr
的 char
指標,並將 tmp_string
中第一個字元的地址分配給它。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*\n";
string mod_string = "I've been overwritten";
char *tmp_ptr = &tmp_string[0];
cout << tmp_ptr;
return EXIT_SUCCESS;
}
但請記住,修改 tmp_ptr
中的資料也會改變 tmp_string
的值,你可以參考下面的程式碼示例。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*\n";
string mod_string = "I've been overwritten";
char *tmp_ptr = &tmp_string[0];
memmove(tmp_ptr, mod_string.c_str(), mod_string.length());
cout << tmp_string;
return EXIT_SUCCESS;
}
作者: Jinku Hu
相關文章 - C++ String
- C++ 中字串和字元的比較
- 從 C++ 中的字串中刪除最後一個字元
- 從 C++ 中的字串中獲取最後一個字元
- C++ 中字串的 sizeof 運算子和 strlen 函式的區別
- C++ 中的 std::string::erase 函式
- 在 C++ 中列印字串的所有排列