Pandas DataFrame DataFrame.interpolate()函式
-
pandas.DataFrame.interpolate()
語法 -
示例程式碼:用
DataFrame.interpolate()
方法對DataFrame
中所有NaN
值進行內插 -
示例程式碼:
DataFrame.interpolate()
方法用method
引數 -
示例程式碼:Pandas
DataFrame.interpolate()
方法使用axis
引數沿row
軸進行插值 -
示例程式碼:
DataFrame.interpolate()
方法帶limit
引數 -
示例程式碼:
DataFrame.interpolate()
方法帶limit_direction
引數的方法 -
用
DataFrame.interpolate()
方法對時間序列資料進行內插
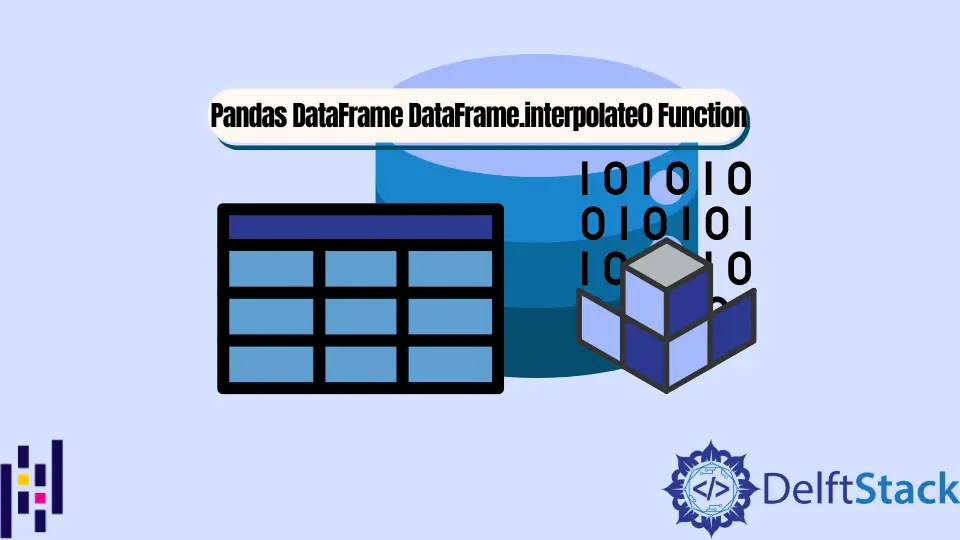
Python Pandas DataFrame.interpolate()
函式使用插值技術在 DataFrame 中填充 NaN
值。
pandas.DataFrame.interpolate()
語法
DataFrame.interpolate(
method="linear",
axis=0,
limit=None,
inplace=False,
limit_direction="forward",
limit_area=None,
downcast=None,
**kwargs
)
引數
method |
linear , time , index , values , nearest , zero , slinear , quadratic , cubic , barycentric , krogh , polynomial , spline , piecewise_polynomial , from_derivatives , pchip , akima 或 None 。用於插值 NaN 的方法。 |
axis |
沿行(axis=0 )或列(axis=1 )插補缺失的數值 |
limit |
要內插的最大連續 NaN 數 |
inplace |
布林型。如果 True ,就地修改呼叫方 DataFrame 。 |
limit_direction |
forward , backward 或 both 。當指定 limit 時,將沿 NaNs 的 Direction 進行插值。 |
limit_area |
None , inside 或 outside 。當指定 limit 時,對插值的限制。 |
downcast |
字典。指定向下轉換資料型別 |
**kwargs |
插值函式的關鍵字 |
返回值
如果 inplace
為 True
,則使用給定的 method
對所有 NaN
值進行內插的 DataFrame
;否則為 None
。
示例程式碼:用 DataFrame.interpolate()
方法對 DataFrame
中所有 NaN
值進行內插
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, 8, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate()
print("Interploated DataFrame:")
print(filled_df)
輸出:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 8.0
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.0 4.0
1 2.0 6.0
2 3.0 8.0
3 3.0 5.5
4 3.0 3.0
它使用 linear
插值方法對 DataFrame
中的所有 NaN
值進行內插。
該方法與 pandas.DataFrame.fillna()
相比更加智慧,後者使用一個固定的值來替換 DataFrame.
中的所有 NaN
值。
示例程式碼:DataFrame.interpolate()
方法用 method
引數
我們也可以在 DataFrame.interpolate()
函式中設定 method
引數值,用不同的插值技術對 DataFrame
中的 NaN
值進行插值。
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, 8, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate(method='polynomial', order=2)
print("Interploated DataFrame:")
print(filled_df)
輸出:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 8.0
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.000000 4.000
1 2.000000 7.125
2 3.000000 8.000
3 3.368421 6.625
4 3.000000 3.000
該方法使用二階多項式插值方法對 DataFrame
中的所有 NaN
值進行插值。
這裡,order=2
是 polynomial
函式的關鍵字引數。
示例程式碼:Pandas DataFrame.interpolate()
方法使用 axis
引數沿 row
軸進行插值
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, 8, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate(axis=1)
print("Interploated DataFrame:")
print(filled_df)
輸出:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 8.0
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.0 4.0
1 2.0 2.0
2 3.0 8.0
3 NaN NaN
4 3.0 3.0
這裡,我們設定 axis=1
,以沿行軸插值 NaN
值。在第 2 行,NaN
值被沿第 2 行線性內插替換。
但是,在第 4 行中,由於第 4 行中的兩個值都是 NaN
,所以即使在內插後,NaN
值仍然存在。
示例程式碼:DataFrame.interpolate()
方法帶 limit
引數
DataFrame.interpolate()
方法中的 limit
引數限制了該方法所要填充的連續 NaN
值的最大數量。
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, None, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate( limit = 1)
print("Interploated DataFrame:")
print(filled_df)
輸出:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 NaN
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.0 4.00
1 2.0 3.75
2 3.0 NaN
3 3.0 NaN
4 3.0 3.00
在這裡,當一列中的一個 NaN
值從上到下被填滿後,同一列中下一個連續的 NaN
值將保持不變。
示例程式碼:DataFrame.interpolate()
方法帶 limit_direction
引數的方法
DataFrame.interpolate()
方法中的 limit-direction
引數控制沿著特定軸的方向,在這個方向上進行數值插值。
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, None, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate(limit_direction ='backward', limit = 1)
print("Interploated DataFrame:")
print(filled_df)
輸出:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 NaN
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.0 4.00
1 2.0 NaN
2 3.0 NaN
3 3.0 3.25
4 3.0 3.00
在這裡,當一列中的 NaN
從底部填入後,同一列中下一個連續的 NaN
值將保持不變。
用 DataFrame.interpolate()
方法對時間序列資料進行內插
import pandas as pd
dates=['April-10', 'April-11', 'April-12', 'April-13']
fruits=['Apple', 'Papaya', 'Banana', 'Mango']
prices=[3, None, 2, 4]
df = pd.DataFrame({'Date':dates ,
'Fruit':fruits ,
'Price': prices})
print(df)
df.interpolate(inplace=True)
print("Interploated DataFrame:")
print(df)
輸出:
Date Fruit Price
0 April-10 Apple 3.0
1 April-11 Papaya NaN
2 April-12 Banana 2.0
3 April-13 Mango 4.0
Interploated DataFrame:
Date Fruit Price
0 April-10 Apple 3.0
1 April-11 Papaya 2.5
2 April-12 Banana 2.0
3 April-13 Mango 4.0
由於 inplace=True
,在呼叫 interpolate()
函式後,原 DataFrame
被修改。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn