Python Numpy.mean() - 算術平均數
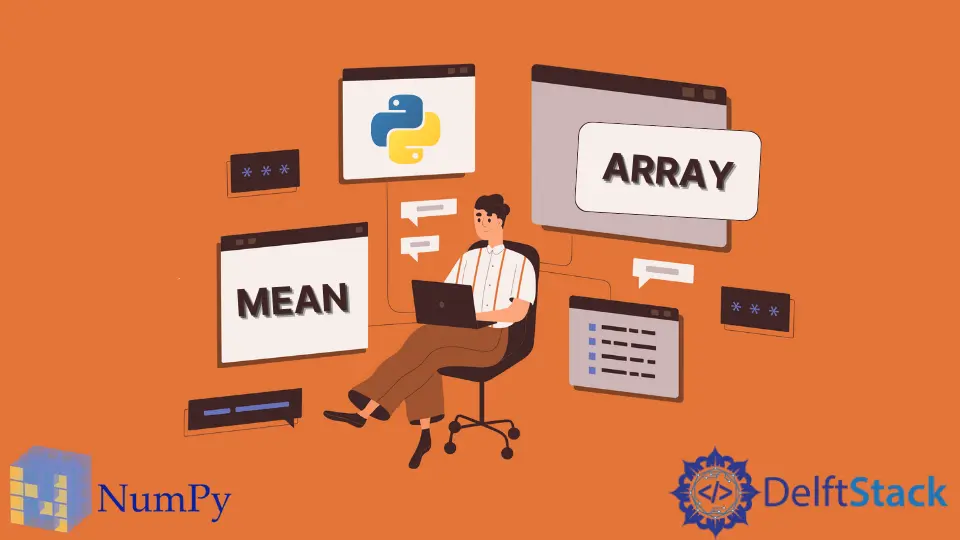
numpy.mean()
函式計算給定陣列沿指定軸的算術平均值,或者用通俗的話說-平均數。
numpy.mean()
語法
numpy.mean(arr, axis=None, dtype=float64)
引數
arr |
array_like 輸入陣列來計算算術平均值 |
axis |
None , int 或元素為整數的元組 計算算術平均值的軸。 axis=0 表示沿列計算算術平均值, axis=1 表示沿行計算算術平均值。 如果沒有給定 axis ,它將多維陣列作為一個扁平化的列表處理 |
dtype 是指 |
dtype 或 None 在計算算術平均值時使用的資料型別。預設為 float64 |
返回值
它返回給定陣列的算術平均數,或一個沿指定軸的算術平均數的陣列。
示例程式碼:numpy.mean()
與一維陣列
import numpy as np
arr = [10, 20, 30]
print("1-D array :", arr)
print("Mean of arr is ", np.mean(arr))
輸出:
1-D array : [10, 20, 30]
Mean of arr is 20.0
示例程式碼:numpy.mean()
與二維陣列
import numpy as np
arr = [[10, 20, 30], [3, 50, 5], [70, 80, 90], [100, 110, 120]]
print("Two Dimension array :", arr)
print("Mean with no axis :", np.mean(arr))
print("Mean with axis along column :", np.mean(arr, axis=0))
print("Mean with axis aong row :", np.mean(arr, axis=1))
輸出:
Two Dimension array : [[10, 20, 30], [3, 50, 5], [70, 80, 90], [100, 110, 120]]
Mean with no axis : 57.333333333333336
Mean with axis along column : [45.75 65. 61.25]
Mean with axis aong row : [ 20. 19.33333333 80. 110. ]
>>
np.mean(arr)
將輸入陣列視為扁平化陣列,並計算這個 1-D 扁平化陣列的算術平均值。
np.mean(arr, axis=0)
計算沿列的算術平均值。
np.std(arr, axis=1)
計算沿行的算術平均值。
示例程式碼:numpy.mean()
指定了 dtype
import numpy as np
arr = [10.12, 20.3, 30.28]
print("1-D Array :", arr)
print("Mean of arr :", np.mean(arr))
print("Mean of arr with float32 data :", np.mean(arr, dtype=np.float32))
print("Mean of arr with float64 data :", np.mean(arr, dtype=np.float64))
輸出:
1-D Array : [10.12, 20.3, 30.28]
Mean of arr : 20.233333333333334
Mean of arr with float32 data : 20.233332
Mean of arr with float64 data : 20.233333333333334
如果在 numpy.mean()
函式中給出 dtype
引數,它在計算算術平均值時使用指定的資料型別。
如果我們使用 float32
資料型別,而不是預設的 float64
,則結果的解析度較低。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe