Tkinter Tutorial - Scrollbar
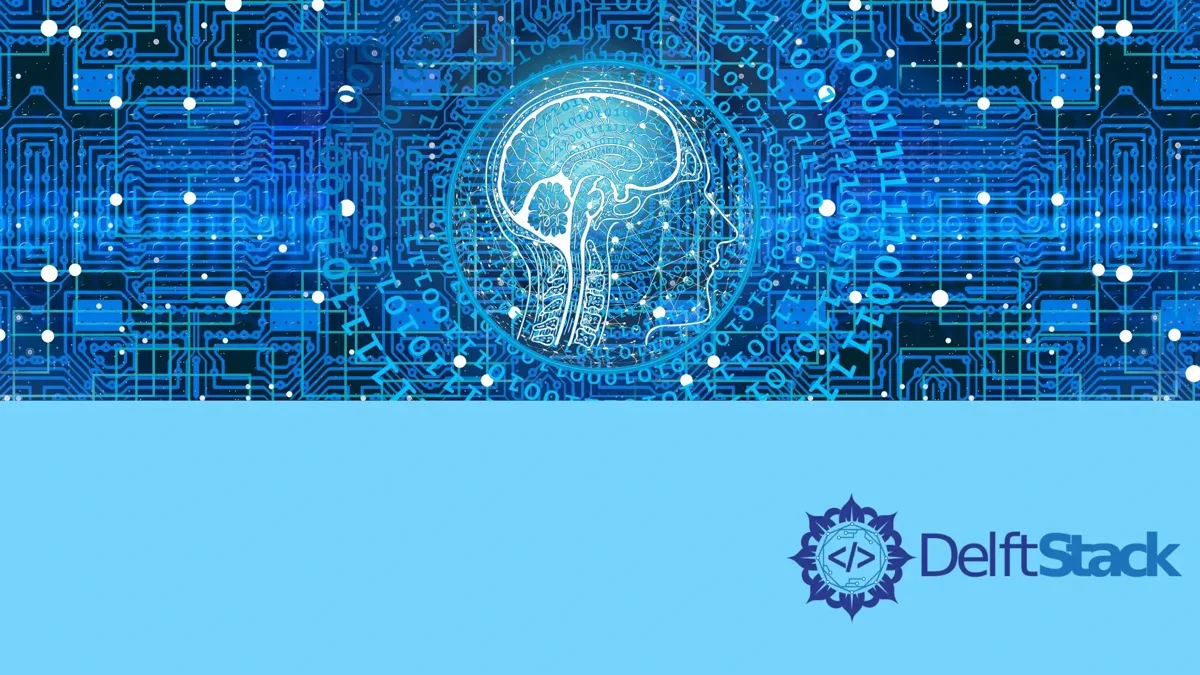
The Tkinter Scrollbar widget is normally used to scroll widgets like ListBox
, Text
or Canvas
vertically, or Entry
horizontally. It shows a slider in the right position.
Tkinter ScrollBar
import tkinter as tk
class Scrollbar_Example:
def __init__(self):
self.window = tk.Tk()
self.scrollbar = tk.Scrollbar(self.window)
self.scrollbar.pack(side="right", fill="y")
self.listbox = tk.Listbox(self.window, yscrollcommand=self.scrollbar.set)
for i in range(100):
self.listbox.insert("end", str(i))
self.listbox.pack(side="left", fill="both")
self.scrollbar.config(command=self.listbox.yview)
self.window.mainloop()
if __name__ == "__main__":
app = Scrollbar_Example()
self.scrollbar = tk.Scrollbar(self.window)
It initiates the Scrollbar
instance.
self.listbox = tk.Listbox(self.window, yscrollcommand=self.scrollbar.set)
self.scrollbar.config(command=self.listbox.yview)
We need configure both Listbox
and Scrollbar
to connect them together correctly.
- Set
yscrollcommand
callback toset
ofScrollbar
.yscrollcommand
is scrollable widgets’ option that is controlled by a scrollbar, and is used to communicted with vertical scrollbars. - Set
command
of theScrollbar
to theyview
of theListbox
. When the user moves the slider ofScrollbar
, it calls theyview
method with the proper argument.
Tkinter Horizontal Scrollbar
The horizontal scroll bar is used to scroll the widgets like Text
and Entry
in the horizontal orientation.
import tkinter as tk
class Scrollbar_Example:
def __init__(self):
self.window = tk.Tk()
self.scrollbar = tk.Scrollbar(self.window, orient=tk.HORIZONTAL)
self.scrollbar.pack(side="bottom", fill="x")
self.text = tk.Text(self.window, wrap="none", xscrollcommand=self.scrollbar.set)
self.text.insert("end", str(dir(tk.Scrollbar)))
self.text.pack(side="top", fill="x")
self.scrollbar.config(command=self.text.xview)
self.window.mainloop()
if __name__ == "__main__":
app = Scrollbar_Example()
self.scrollbar = tk.Scrollbar(self.window, orient=tk.HORIZONTAL)
It initiates a horizontal scroll bar by specifying the orient
to be HORIZONTAL
.
self.text = tk.Text(self.window, wrap="none", xscrollcommand=self.scrollbar.set)
To scroll the text horizontally, we need to set xscrollcommand
to the set
method of the Scrollbar
, but not yscrollcommand
as in the above example.
self.scrollbar.config(command=self.text.xview)
Correspondingly, the callback of the horizontal scroll bar should be connected with the xview
method but not yview
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook