NumPy Tutorial - NumPy Data Type and Conversion
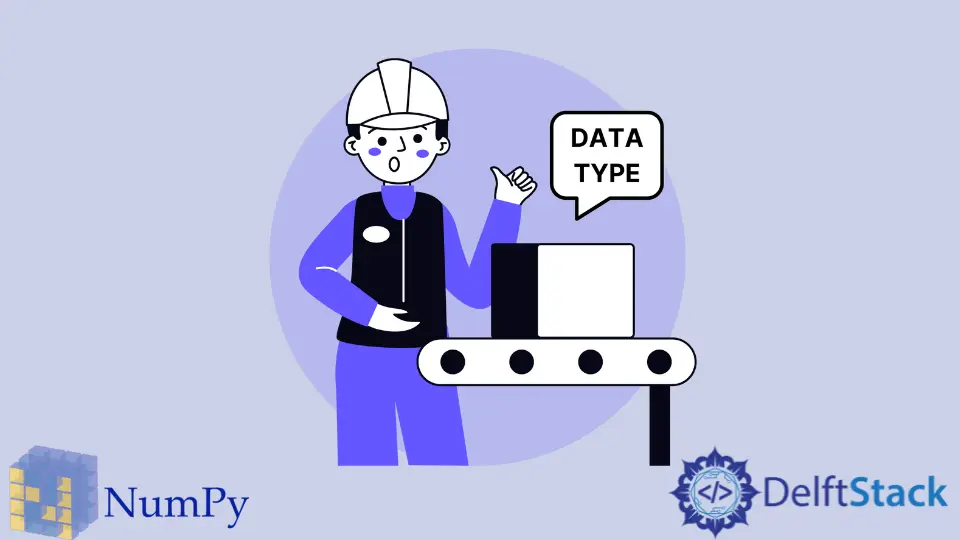
Data type - dtype
in NumPy is different from the primitive data types in Python, for example, dtype
has the type with higher resolution that is useful in the data calculation.
NumPy Data Type
Data Type | Description |
---|---|
bool |
Boolean |
int8 |
8-bit signed integer |
int16 |
16-bit signed integer |
int32 |
32-bit signed integer |
int64 |
64-bit signed integer |
uint8 |
8-bit unsigned integer |
uint16 |
16-bit unsigned integer |
uint32 |
32-bit unsigned integer |
uint64 |
64-bit unsigned integer |
float16 |
16-bit floating point number |
float32 |
32-bit floating point number |
float64 |
64-bit floating point number |
complex64 |
64-bit complex number |
complex128 |
128-bit complex number |
When creating a new ndarray
data, you can define the data type of the element by string or or data type constants in the NumPy
library.
import numpy as np
# by string
test = np.array([4, 5, 6], dtype="int64")
# by data type constant in numpy
test = np.array([7, 8, 8], dtype=np.int64)
Data Type Conversion
After the data instance is created, you can change the type of the element to another type with astype()
method, such as from integer to floating and so on.
>>> import numpy as np
>>> test = np.array([11, 12, 13, 14], dtype="int32")
>>> x = test.astype('float32')
>>> x
array([11., 12., 13., 14.], dtype=float32)
>>> test, test.dtype
(array([11, 12, 13, 14]), dtype('int32'))
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook