Python Tutorial - File Operation
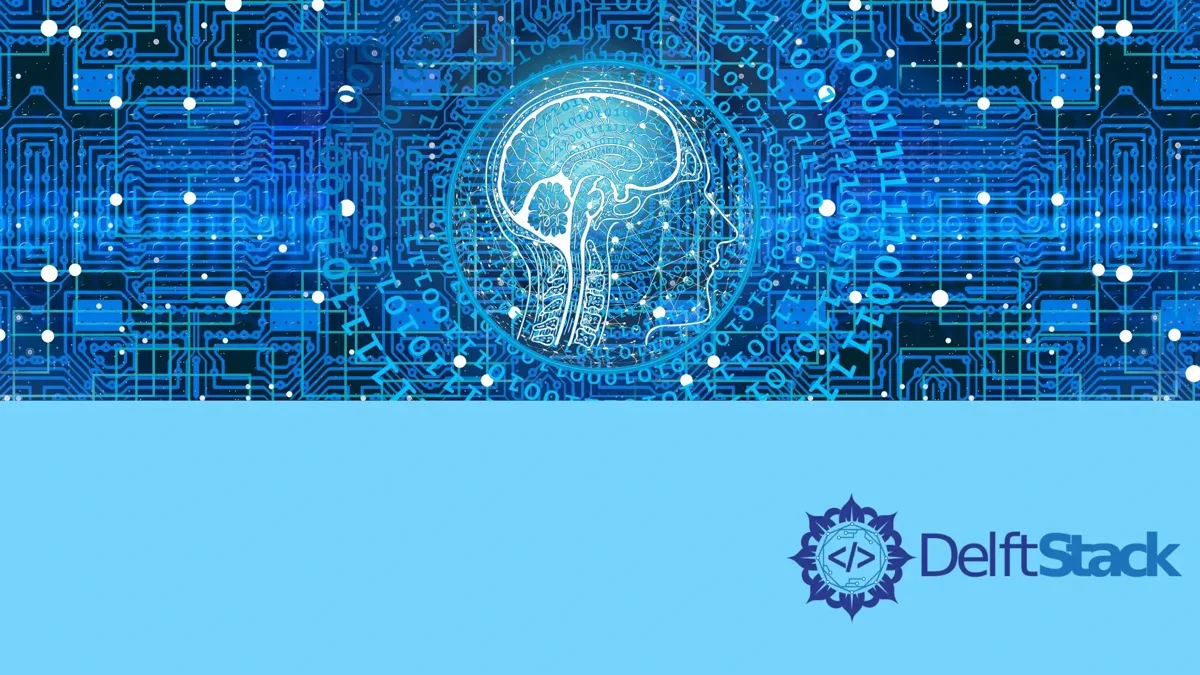
In this section, you will learn how to perform operations on files in Python programming.
Using Python you can open a file, read or write something to a file and then close a file. This can be done by using some file manipulating functions. To perform any operation on a file you have to create an object of the file first.
Open a File
A file can be opened using open()
function. A file object is created which is later used to perform operations on the file.
The syntax to open a file is as follows:
obj = open(fileName, mode)
fileName
is the name of the file, mode
defines the mode in which the file will be opened for example r
to open a file in reading mode.
fobj = open("PythonExamplefile.txt", "r")
The file PythonExamplefile
will be opened in read mode.
Modes to Open a File
Modes | Meaning |
---|---|
r |
File is opened in reading mode. |
rb |
File is opened in the reading mode in binary format. |
r+ |
File is opened in both reading and writing modes. |
rb+ |
File is opened in both read and writing mode in binary format. |
w |
File is opened in writing mode. If the file doesn’t exist, a new file will be created. If the file exists, it will be overwritten. |
wb |
File is opened in writing mode in binary format. If the file doesn’t exist, a new file will be created. If the file exists, it will be overwritten. |
w+ |
File is opened in both reading and writing mode. If the file doesn’t exist, a new file will be created for reading and writing. If the file exists, it will be overwritten. |
wb+ |
File is opened in both reading and writing mode in binary format. If the file doesn’t exist, a new file will be created for reading and writing. If the file exists, it will be overwritten. |
a |
File is opened in append mode. The new data will be written at the end of the file. If the file doesn’t exist, a new file will be created for writing. |
ab |
File is opened in append mode in binary format. The new data will be written at the end of the file. If the file doesn’t exist, a new file will be created for reading and writing. |
a+ |
File is opened in both append and reading mode. The new data will be written at the end of the file. If the file doesn’t exist, a new file will be created for reading and writing. |
ab+ |
File is opened in both append and reading mode. The new data will be written at the end of the file. If the file doesn’t exist, a new file will be created for reading and writing. |
Close a File
After performing operations on a file, a file must be closed. The close()
method is used to close a file.
fobj.close()
fobj = open("PythonExamplefile.txt", "r")
# operations on file
fobj.close()
File Object
When a file is opened, a file object is created which can be used to perform operations. For example, you can get information about a file such as its name, the mode in which the file is opened, etc.
fobj = open("PythonExamplefile.txt", "r")
print("File name", fobj.name)
print("File mode", fobj.mode)
fobj.close()
Result:
File name PythonExamplefile.txt
File mode r
Write a File
The write()
method is used write something on a file. The file must be opened to write on.
The syntax to use write()
method is as follows:
fobj.write("string")
Example:
fobj = open("PythonExamplefile.txt", "w")
fobj.write("Hello Python Programming")
fobj.close()
The following content will be written to the file:
Hello Python Programming
Read a File
You can read from a file by using the read()
method. Reading from a file is getting input from the file.
The following is the syntax to read from a file:
fobj.read(numberofBytes)
Here numberofBytes
is the total number of bytes that are read from the file.
fobj = open("PythonExamplefile.txt", "r")
content = fobj.read(5)
print(content)
fobj.close()
Hello
Rename and Remove a File
Rename a File
The rename()
method is used to rename a file.
The syntax of rename()
is as follows:
os.rename(currentName, newName)
Here os
is a module which lets you perform functions that are Operating System dependent.
Example:
import os
os.rename("PythonExamplefile.txt", "Python.txt")
The new name of the file is Python.txt
now.
Remove a File
A file is deleted by using remove()
method.
The syntax of remove()
is as follows:
os.remove(nameofFile)
Example:
import os
os.remove("Python.txt")
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook