Python Tutorial - Data Type-String
- Create Strings in Python
- Access Elements of Strings
- Python String Operations
- Built-In Functions Applicable to Strings
- Python String Formatting
-
the String
Format()
Method
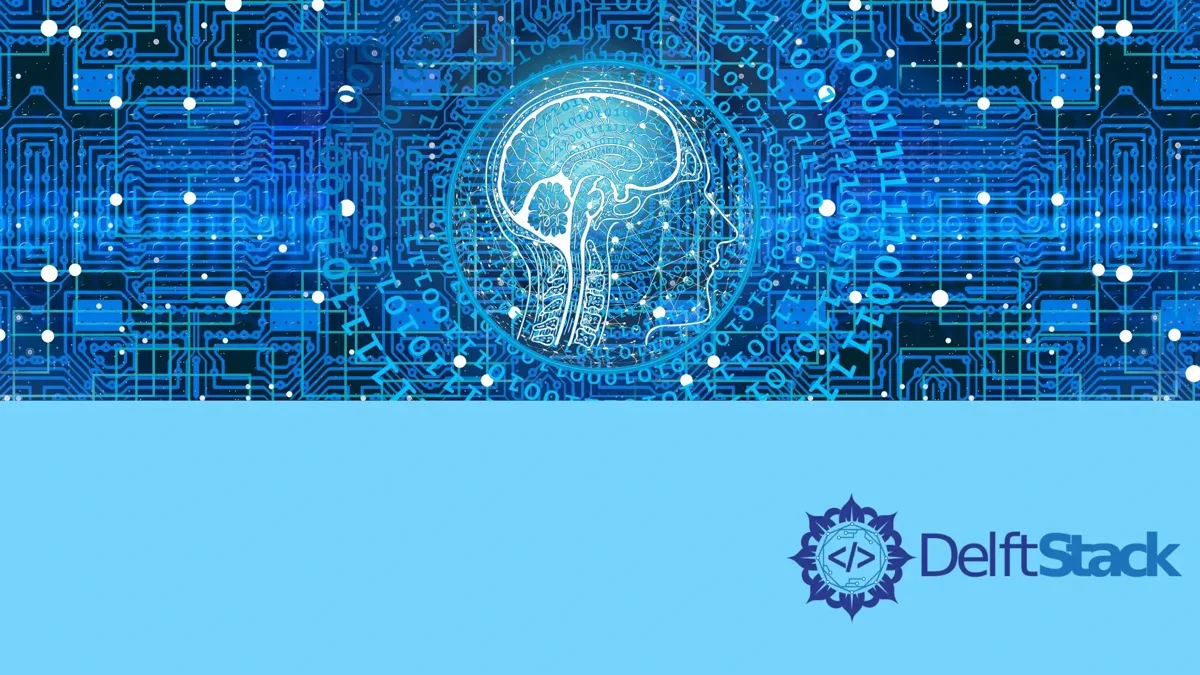
We will cover Python String data type in this section.
A string is a sequence of characters, represented by binary data in the computer. And in Python, a string is a collection of unicode
characters.
Create Strings in Python
A string can be created using single or double quotation marks. A multi-line string can also be created by using triple quotation marks.
x = "String of characters"
x = """Multiline
string"""
Access Elements of Strings
Slicing operator []
is used to access characters of strings. The index starts from 0 as other Python data types.
>>> x = 'Python Programming'
>>> print('x[0] =', x[0])
'P'
>>> print('x[4:15] =', x[4:15])
'on Programm'
>>> x[4] = 'l'
TypeError: 'str' object does not support item assignment
TypeError
will occur.Negative Indexing
It is allowed to use negative indexes to access characters in the string. For example, index -1
refers to the last character. Similarly, index -2
refers to the second last character.
>>> s = "Python"
>>> print(s[-1])
'n'
>>> print(s[-2])
'o'
>>> print(s[-3])
'h'
Delete Characters From a String
As strings are immutable, therefore, the characters from a string cannot be deleted. But you can assign a new string to the same name. This is demonstrated in the code below:
>>> s = 'Python'
>>> s = 'Program'
>>> s
'Program'
The entire string can be deleted using del
keyword:
>>> del s
Python String Operations
There are a number of operations that can be performed on strings; some of them are as follows:
Concatenate Two or More Strings
Two or more strings can be concatenated by using +
operator.
>>> s1 = 'Python '
>>> s2 = 'Programming'
>>> print(s1 + s2)
'Python Programming'
If you write two strings together, it will concatenate the strings as the +
operator does:
>>> print('Python ' 'Programming')
'Python Programming'
Parenthesis can also be used to concatenate two strings when the strings are in multiple lines.
>>> s = ('Python '
'Programming')
>>> s
'Pyhton Programming'
Iterate Through Strings
You can iterate through a list by using for
loop:
s = "Python"
for i in s:
print(i)
P
y
t
h
o
n
String Membership Check
The in
keyword is used to check the existence of a substring in a string.
>>> s = 'Python'
>>> print('a' in s)
False
>>> print('o' in s)
True
Built-In Functions Applicable to Strings
The functions that are applied to other types of sequences can also be applicable to strings. The commonly used functions are len()
to find the number of characters in a string, and enumerate()
which returns an object containing index and value of elements in the string as a pair.
Python String Formatting
Escape Sequence
Escape sequences are used when you want to print the special symbols (that has special meanings in Python) on the output stream. For example, if you want to print:
James asked, "Do you know Andrew's place?"
Here you cannot use either single or double quotation marks because the text contains both quotation marks. If you try to put this strings inside single or double quotations, you will have SyntaxError
.
>>> print("James asked, "Do you know Andrew's place?"")
SyntaxError: invalid syntax
>>> print('James asked, "Do you know Andrew's place?"')
SyntaxError: invalid syntax
The solution to this problem is that you use either triple quotation marks or escape sequences.
In Python, an escape sequence starts from a backslash. A backslash is an instruction to the interpreter.
If you are using single quotes in print statement, then all the single quotes from the text should be escaped. And in the same way, double quotes from the text should be escaped if you are using double quotes to represent a string.
# using triple quotation marks
print('''James asked, "Do you know Andrew's place?"''')
# escaping single quotes
print('James asked, "Do you know Andrew\'s place?"')
# escaping double quotes
print('James asked, "Do you know Andrew\'s place?"')
Consider the following table in which escape sequences of Python are described:
Escape sequence | Description |
---|---|
\\ |
escapes backslash |
\' |
escapes single quotes |
\" |
escapes double quotes |
\a |
an ASCII alert or bell |
\b |
generates a backspace |
\f |
generates Formfeed |
\n |
generates a new line. |
\r |
carriage return |
\t |
generates a horizontal tab |
\v |
generates a vertical tab |
\ooo |
octal character ooo |
\xHH |
hexadecimal character HH |
>>> print("C:\\User\\Python36-32\\Lib")
C:\User\Python36-32\Lib
>>> print("First Line\nSecond Line")
First Line
Second Line
Raw String r
to Ignore Escape Sequence
You can ignore the escape sequence in a string by making it a raw string that is indicated by r
or R
before the string.
In a raw string escape sequences are ignored. See the following example:
#without r
>>> print("C:\\User\\Python36-32\\Lib")
C:\User\Python36-32\Lib
#with r
>>> print(r"C:\\User\\Python36-32\\Lib")
C:\\User\\Python36-32\\Lib
You could see here that escape sequence is ignored.
the String Format()
Method
The format()
method is a powerful tool to format strings. In the formatted strings, you have placeholders {}
that are replaced by format()
method.
The positional and keyword arguments are used to specify the order of the replacement.
#default placeholder
>>> s1 = "{}, {} and {}".format('Bread', 'Butter', 'Chocolate')
>>> print(s1)
'Bread, Butter and Chocolate'
#positional arguments
>>> s2 = "{1}, {2} and {0}".format('Bread', 'Butter', 'Chocolate')
>>> print(s2)
'Butter, Chocolate and Bread'
#keyword arguments
>>> s3 = "{a}, {x} and {c}".format(x = 'Bread', c = 'Butter', a = 'Chocolate')
>>> print(s3)
'Chocolate, Bread and Butter'
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook