Python Tutorial - Data Type-List
- Create a Python List
- Access Python List Elements
- Add Elements to a Python List
- Delete Elements From a Python List
- Python List Methods
- Python List Membership Check
- List Elements Iteration
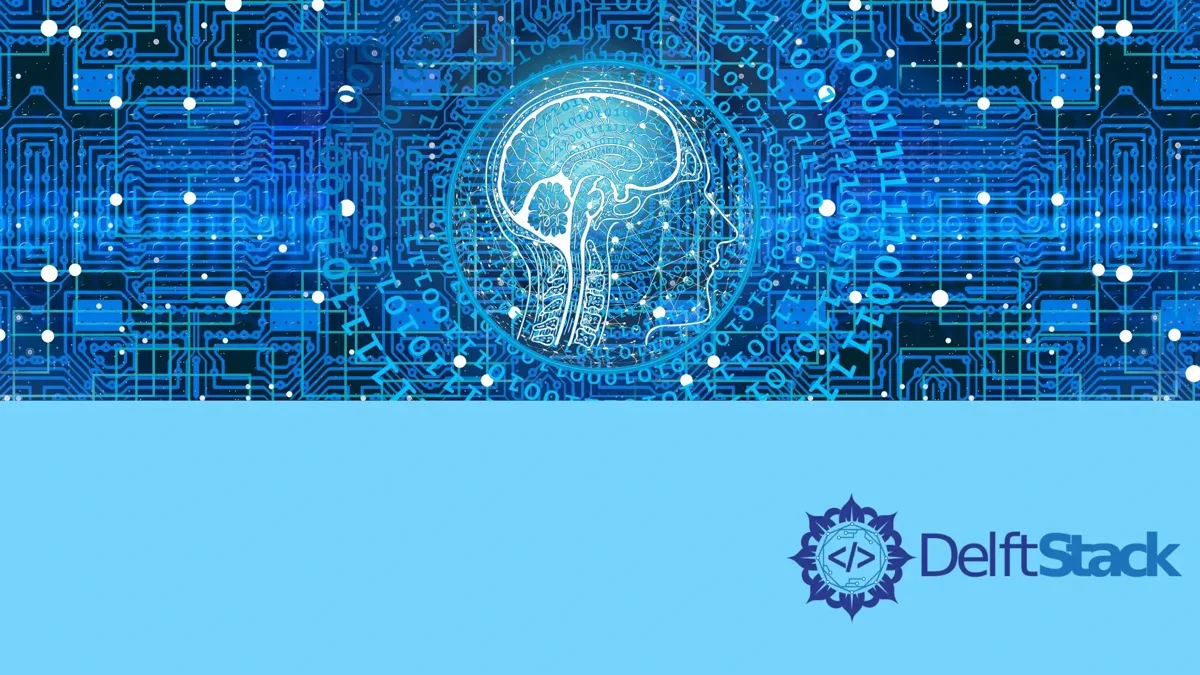
In this section, you will learn to create and update a list in Python programming. Further, you will learn how to slice a list in Python.
Create a Python List
A list can be created by using square brackets []
. The elements of a list are placed inside square brackets, and are separated from each other by a comma ,
. The elements of a list could have different data types.
l = [] # empty list
l = [2, 4, 6, 8] # elements of same data type
l = [2, "Python", 1 + 2j] # elements of different data type
You can also create a nested list, that is list inside another list.
l = [[2, 4, 5], "python"]
Access Python List Elements
The following are some of the way using which you can access list elements:
List Index
You could use index to access elements of a list. The index of a list starts from 0.
>>> x = [2, 4, 6, 8, 10]
>>> print('x[0] =', x[0]) # display the first element
x[0] = 2
>>> print('x[0:2] =', x[0:2]) # display the first two elements of list that is at location 0 and 1
x[0:2] = [2, 4]
>>> print('x[2:] =', x[2:]) # display elements from location 2 (third element)
x[2:] = [6, 8, 10]
The list element can be modified (updated, deleted, added) because list
is mutable. If the index is outside of the list index range, you will have an IndexError
.
TypeError
will occur.Python List Negative Index
It is allowed to use negative indexes to access elements in the list. For example, index -1
refers to the last element. Similarly, index -2
refers to the second last item.
>>> l = [2, 4, 6, 8, 10]
>>> print(l[-1])
10
>>> print(l[-2])
8
>>> print(l[-3])
6
Slice a List in Python
A slicing operator :
is used to extract a range of elements from a list.
>>> l = [2, 4, 6, 8, 10]
>>> print(l[1:3]) # print from location 1 to 3
[4, 6]
>>> print(l[:2]) # print from beginning to location 2
[2, 4]
>>> print(l[2:]) # print elements from location 2 onwards
[6, 8, 10]
>>> print(l[:]) # print the entire list
[2, 4, 6, 8, 10]
Add Elements to a Python List
Apend()
You can use the list method append()
to append an item to the end of a list.
>>> l = [1, 3, 5, 7, 9, 11]
>>> l.append(13)
>>> print(l)
[1, 3, 5, 7, 9, 11, 13]
Extend()
append(x)
adds the item x as one item to the list. extend(x)
method is the right method to extend the list by appending all the items in the given object x
.
>>> l = [1, 3, 5, 7, 9, 11]
>>> l.extend([13, 14, 15])
>>> print(l)
[1, 3, 5, 7, 9, 11, 13, 14, 15]
+
Operator
Besides extend()
method, you could concatenate two lists by using +
operator.
>>> l = [2, 4, 6, 8, 10]
>>> print(l + [12, 14, 16])
[2, 4, 6, 8, 10, 12, 14, 16]
Insert(i, X)
You could use insert(i, x)
method to insert the element x
to the given index i
.
>>> l = [2, 4, 6, 8, 10]
>>> l.insert(2, 0)
>>> print(l)
[2, 4, 0, 6, 8, 10]
Delete Elements From a Python List
The del
statement deletes one or more items from a list. You can also use del
to delete the entire list:
>>> l = [2, 4, 6, 8, 10]
>>> del l[4]
>>> print(l)
[2, 4, 6, 8]
>>> del l[0:2]
>>> print(l)
[6, 8]
>>> del l
>>> print(l)
Traceback (most recent call last):
print(l)
NameError: name 'l' is not defined
After a list is deleted by del l
, referencing this list l
is an error.
If you want to clear a list then you can also use clear()
method.
Python List Methods
The following table describes the Python list methods:
Methods | Description |
---|---|
append() |
add an item to the end of the list |
extend() |
add more than one items to a list. It works by adding elements of a list to another list (in which you want to add elements). |
insert() |
add an element at a desired location. |
remove() |
remove a particular item from the list. |
pop() |
remove an item from a given location and returns that item. |
clear() |
remove all elements from the list. |
index() |
return the index of the first matched element in the list. |
count() |
return the total number of items passed |
sort() |
sort elements of a list in ascending order. |
reverse() |
reverse the order of the elements of the list. |
copy() |
return a copy of an already existing list. |
Python List Membership Check
The in
keyword checks if an element is a member of the list or not.
>>> l = [2, 4, 6, 8, 10]
>>> print(5 in l)
False
>>> print(2 in l)
True
List Elements Iteration
You can iterate through a list by using for
loop:
l = [2, 4, 6, 8, 10]
for i in l:
print(i)
2
4
6
8
10
Built-In Functions Applicable to List
The following table is the collection of built-in functions that can be used with lists to perform different tasks:
Functions | Description |
---|---|
all() |
return True when all the elements of the list are True . It also returns True when the list is empty. |
any() |
return True when any of the element of the list is True . It returns False when the list is empty. |
enumerate() |
return the index and the value of all the elements of the list as a tuple. It returns an enumerate object. |
len() |
return the number of items in a list or the length of the list. |
list() |
convert a sequence (tuple, set, string, dictionary) to list. |
max() |
return the maximum number in the list. |
min() |
return the minimum number in the list. |
sorted() |
return a sorted list. |
sum() |
return the sum of all elements of the list. |
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook