PyQt5 Tutorial - Push Button
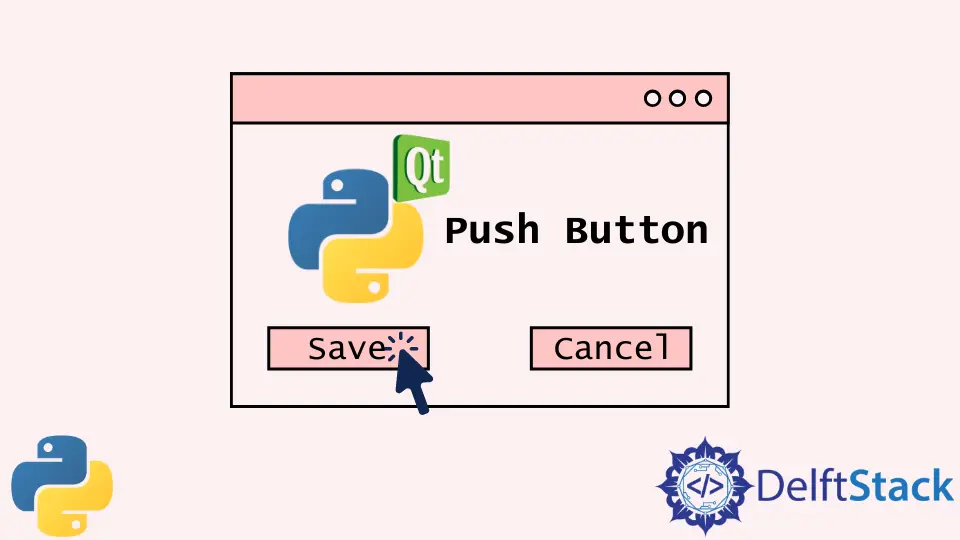
Push button widget QPushButton
is a command button in PyQt5. It is clicked by the user to command the PC to perform some specific action, like Apply
, Cancel
and Save
.
PyQt5 Push Button - QPushButton
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
buttonA = QtWidgets.QPushButton(windowExample)
labelA = QtWidgets.QLabel(windowExample)
buttonA.setText("Click!")
labelA.setText("Show Label")
windowExample.setWindowTitle("Push Button Example")
buttonA.move(100, 50)
labelA.move(110, 100)
windowExample.setGeometry(100, 100, 300, 200)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
Where,
buttonA = QtWidgets.QPushButton(windowExample)
buttonA
is the QPushButton
from QtWidgets
and it should be added to the window windowExample
the same as labels introduced in last chapters.
buttonA.setText("Click!")
It sets the text of buttonA
to be Click!
.
It’s actually not going to do anything.
PyQt5 QLabel
Label Widget Set Style
The style of PyQt5 QLabel
widget like background color, font family and font size could be set with setStyleSheet
method. It works like style sheet in CSS.
buttonA.setStyleSheet(
"background-color: red;font-size:18px;font-family:Times New Roman;"
)
It sets buttonA
with the following styles,
Style | Value |
---|---|
background-color |
red |
font-size |
18px |
font-family |
Times New Roman |
It is convenient to set the styles in PyQt5 because it is similar to CSS.
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
buttonA = QtWidgets.QPushButton(windowExample)
labelA = QtWidgets.QLabel(windowExample)
buttonA.setStyleSheet(
"background-color: red;font-size:18px;font-family:Times New Roman;"
)
buttonA.setText("Click!")
labelA.setText("Show Label")
windowExample.setWindowTitle("Push Button Example")
buttonA.move(100, 50)
labelA.move(110, 100)
windowExample.setGeometry(100, 100, 300, 200)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
PyQt5 QLabel
Label Click Event
The button click event is connected to some specific function with QLabel.clicked.connect(func)
method.
import sys
from PyQt5 import QtWidgets
class Test(QtWidgets.QMainWindow):
def __init__(self):
QtWidgets.QMainWindow.__init__(self)
self.buttonA = QtWidgets.QPushButton("Click!", self)
self.buttonA.clicked.connect(self.clickCallback)
self.buttonA.move(100, 50)
self.labelA = QtWidgets.QLabel(self)
self.labelA.move(110, 100)
self.setGeometry(100, 100, 300, 200)
def clickCallback(self):
self.labelA.setText("Button is clicked")
if __name__ == "__main__":
app = QtWidgets.QApplication(sys.argv)
test = Test()
test.show()
sys.exit(app.exec_())
When the QPushButton
buttonA
is clicked, it triggers the function clickCallback
to set the label text to be Button is clicked
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook