Python End of File
-
Using
file.read()
to Detect EOF -
Using
file.readline()
with awhile
Loop -
Using the Walrus Operator (
:=
) withfile.read()
-
Using
file.tell()
andos.fstat()
to Compare File Pointer and Size - Using Iteration Over the File Object
- Conclusion
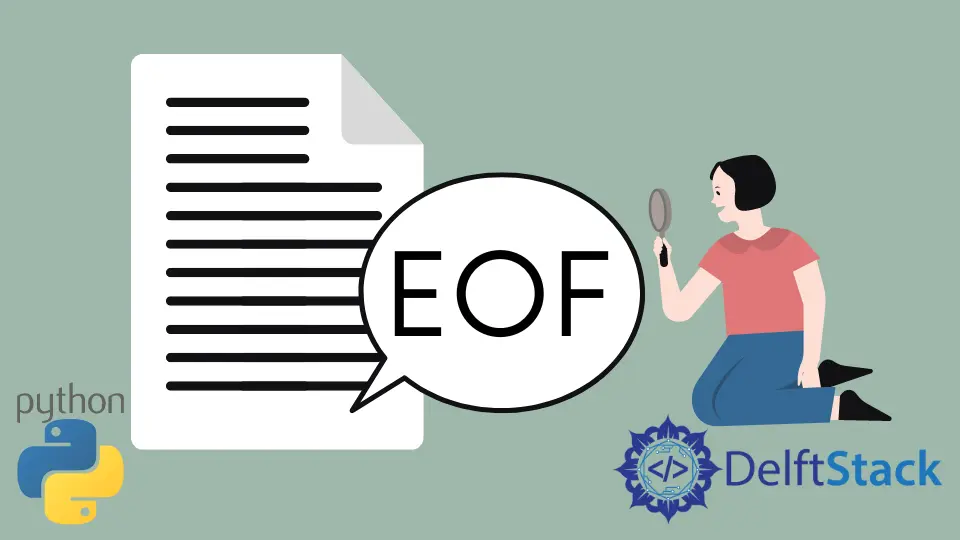
Detecting the end of a file
(EOF
) is a fundamental aspect of file handling in Python. Properly identifying EOF ensures that programs process files accurately without encountering unexpected errors. Python offers several methods to determine when the end of a file has been reached, each suited to different scenarios and use cases.
Using file.read()
to Detect EOF
The read()
method reads the entire content of a file or a specified number of bytes. When it reaches EOF, it returns an empty string (''
). This behavior can be utilized to detect the end of the file during iterative reading.
Example:
with open("example.txt", "r") as file:
while True:
content = file.read()
if content == '':
print("End of file reached.")
break
# Process the content
When opening the file, the open()
function is used with the mode 'r'
, allowing the file to be read.
Within the while
loop, file.read()
attempts to read the content. If the file is empty or has been fully read, it returns an empty string.
The condition if content == '':
checks for this empty string, which signifies EOF. Upon detection, a message is printed, and the loop terminates.
This method is straightforward but may not be memory-efficient for large files, as read()
without arguments loads the entire file into memory.
Using file.readline()
with a while
Loop
The readline()
method reads one line at a time from the file and returns it as a string. When EOF is reached, it returns an empty string, which can be used to detect the end of the file during line-by-line processing.
Example:
with open("example.txt", "r") as file:
while True:
line = file.readline()
if line == '':
print("End of file reached.")
break
# Process the line
The readline()
method reads the next line from the file each time it is called.
If you need to read only specific lines instead of iterating until EOF, refer to our guide on Reading Specific Lines from a File in Python.
When readline()
returns an empty string, it signifies that EOF has been reached, prompting the loop to terminate.
This approach is memory-efficient and suitable for processing large files line by line.
This approach is memory-efficient and suitable for processing large files line by line.
Using the Walrus Operator (:=
) with file.read()
Introduced in Python 3.8, the walrus operator (:=
) allows assignment and evaluation in a single expression. It can be used to read chunks of a file and detect EOF within the loop condition.
Example:
with open("example.txt", "r") as file:
while (chunk := file.read(1024)):
# Process the chunk
print("End of file reached.")
The file.read(1024)
reads up to 1024 bytes from the file.
The walrus operator assigns the result of file.read(1024)
to chunk
and evaluates it. If chunk
is an empty string, the loop terminates, indicating EOF.
This method is efficient for reading large files in manageable chunks.
This method is efficient for reading large files in manageable chunks.
Using file.tell()
and os.fstat()
to Compare File Pointer and Size
The tell()
method returns the current position of the file pointer, while os.fstat()
provides file statistics, including the total size. By comparing the current position with the total size, EOF can be detected.
Example:
import os
with open("example.txt", "r") as file:
file_size = os.fstat(file.fileno()).st_size
while file.tell() < file_size:
line = file.readline()
# Process the line
print("End of file reached.")
The os.fstat(file.fileno()).st_size
retrieves the total size of the file in bytes.
The tell()
method returns the current position of the file pointer. By comparing it to the total file size, the program can determine when EOF is reached.
This method is useful when precise control over the file pointer is required.
This method is useful when precise control over the file pointer is required.
Using Iteration Over the File Object
Python allows iteration over a file object, reading one line at a time until EOF is reached. This implicit handling simplifies the code.
If you specifically need to read only the last line of a file, check out our guide on Reading the Last Line of a File in Python.
Example:
with open("example.txt", "r") as file:
for line in file:
# Process the line
print("End of file reached.")
The for
loop iterates over each line in the file. When no more lines are available, the loop ends naturally, indicating EOF.
This approach is concise and memory-efficient, making it suitable for most line-by-line file processing tasks.
This approach is concise and memory-efficient, making it suitable for most line-by-line file processing tasks.
Conclusion
Choosing the right method depends on factors like file size, memory availability, and the need for efficient data processing. If you need to append new data at the end of a file, refer to our guide on Appending Data and Writing to a File in Python.
Iterating over a file object is often the simplest and most memory-efficient approach, while file.read()
is best suited for smaller files. The walrus operator allows chunk-based reading, which is a good balance between performance and memory efficiency. For cases requiring exact file position tracking, file.tell()
and os.fstat()
provide a reliable solution.
Below is a comparison table summarizing the different methods for detecting EOF in Python:
Method | Memory Usage | Efficiency | Best Use Case |
---|---|---|---|
file.read() |
High | Low | Small files |
file.readline() |
Low | Moderate | Line-by-line processing |
Walrus operator (:= ) with read() |
Moderate | High | Chunk-based reading |
file.tell() and os.fstat() |
Low | Moderate | When precise file control is needed |
Iteration over file object | Low | High | Large files, simple iteration |
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn